Infinite loop
it cannot find a new number, this is the testcase it fails on: [TestCase(100, 100, 1)]

17 Replies
Your message says
[TestCase(100, 100, 1)]
, but your image says [TestCase(1, 100, 4)]
?yes, sorry copied the wrong one
but it fails on both
gets stuck in an infinite loop
Which of the two loops does it get stuck in?
the hasOccurred loop
Works here? https://dotnetfiddle.net/djowvV
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
Btw, you can simplify it to:
Or:
If you ask it for more values than as possible to generate it will get stuck, for sure:
Opdr3RandomNumbers(1, 1, 2)
will get caught. You probably want some guards to avoid that. Something like Opdr3RandomNumbers(1, 10000, 10000)
might take a while to run, as it will need to generate a lot of random numbers before it happens to stumble across the 10000 that it needsit gets stuck on Opdr3RandomNumbers(10, 15, 6); aswell
Yep, of course
You've said "Give me 6 unique numbers between 10 and 15", and there are only 5 unique numbers between 10 and 15
so if i want to include 10 and 15 i should make the bounds 1 higher and lower?
With
Random.Next
, the lower bound is inclusive and the upper is exclusive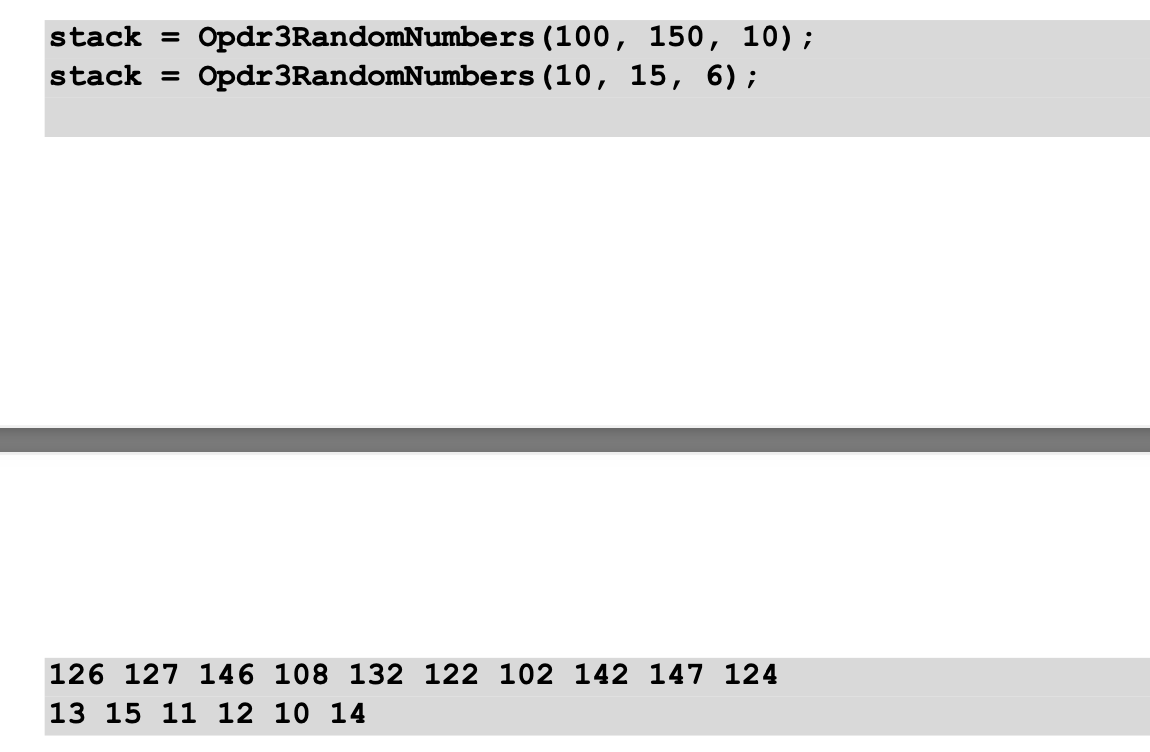
this is what i am trying to reproduce
So if you want the upper bound to be inclusive, you'll need to add 1 to it, yes
okay thank you
This is how Python's
random.sample
does this FWIW: https://github.com/python/cpython/blob/main/Lib/random.py#L363
That can take what's effectively an ICollection<T>
(so something which has a known length, but all items don't need to be listed in advance), and generate a random sample from itGitHub
cpython/Lib/random.py at main · python/cpython
The Python programming language. Contribute to python/cpython development by creating an account on GitHub.
thank you, it finally works