Workflows as Code | Windmill
We are releasing in beta Workflow as code for Python and Typescript. No more excuse to use Airflow or Prefect: https://www.windmill.dev/docs/core_concepts/workflows_as_code
Workflows as Code | Windmill
Flows are not the only way to write distributed programs that execute distinct jobs. Another approach is to write a program that defines the jobs and their dependencies, and then execute that program. This is known as workflows as code.
7 Replies
hey, are there plans on making a typescript decorator? the API for ts does seem annoying to use
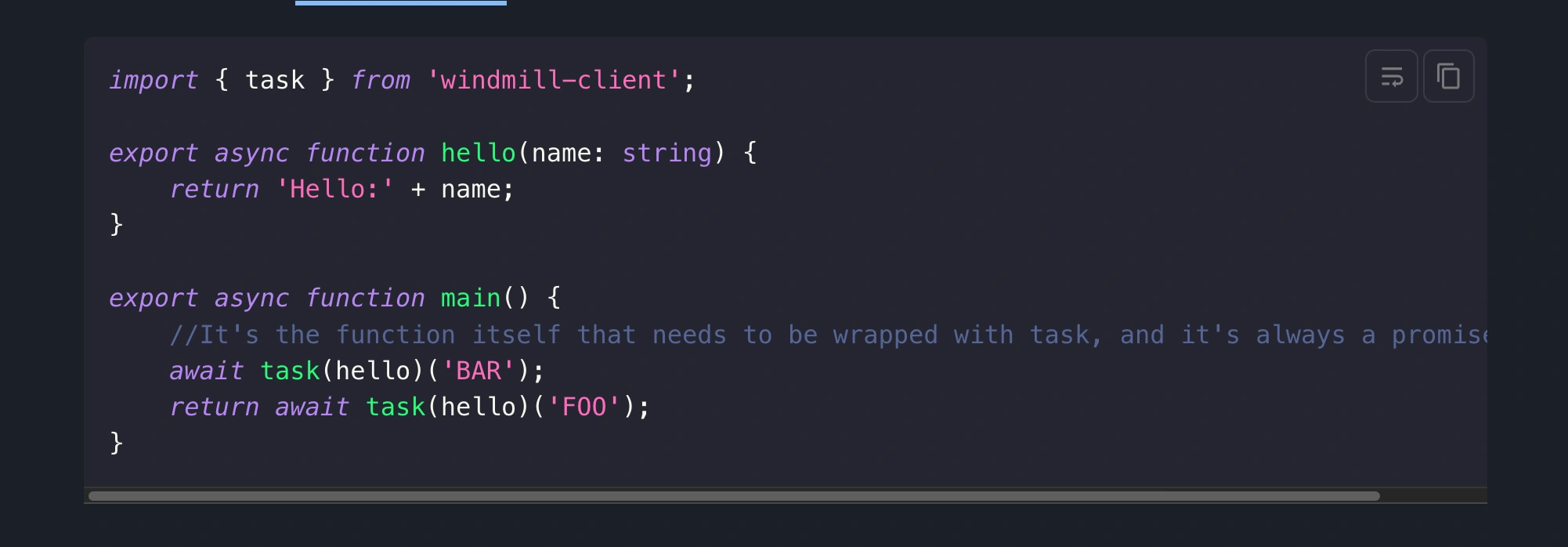
issue is typescript doesn't allow decorator at top level, it require to declare a class
which is also annoying
damn, i forgot
would you be interested in supporting alternative class based approach though?
@flow and @task decorators seem like a neat idea
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
yeah that doesn't look that bad
small update - you can't do this
InternalErr: Internal: Could not add completed job 018eb467-59be-90bb-ee5d-5ddfa1a0f5d4: error returned from database: invalid input syntax for type jsonseems like the individual tasks have to use the
function hello(...)
syntax and therefore the windmill.task()
call must be done in main()
me and @invakid404 also did some further tests and for some reason the tasks can't destructure objects inline:
Error: Job 018eb466-87ee-1607-3b3c-cadb4504bab6 was not successful: {"name":"ExecutionErr","message":"error during execution of the script:\nmain function was not findable (expected to find 'export function main(...)'"}actually, the example i gave isn't related to the destructuring 🤔 we did however fix an error regarding "right hand destructuring assignment" by removing the inline arg destructuring
@fr3fou I don't know if you saw but the task code is open-source: https://github.com/windmill-labs/windmill/blob/main/typescript-client/client.ts#L188
GitHub
windmill/typescript-client/client.ts at main · windmill-labs/windmill
Open-source developer platform to turn scripts into workflows and UIs. Fastest workflow engine (5x vs Airflow). Open-source alternative to Airplane and Retool. - windmill-labs/windmill
it's possible to contribute with your own syntax sugar