Intersection Observer Issues
I'm working on a game and I want some elements to animate in when they become visible, so I'm using intersection observers.
I came up with this solution, and it works perfectly on firefox, but it sometimes bugs out on chrome. Sometimes the elements don't appear, and the screens stays like on pic related. Here's the relevant code
I know there's a library, but since this is a learning project I'd like to do it from scratch to understand things better.
repo: https://github.com/kxrn0/fem_hangman
GitHub
GitHub - kxrn0/fem_hangman
Contribute to kxrn0/fem_hangman development by creating an account on GitHub.
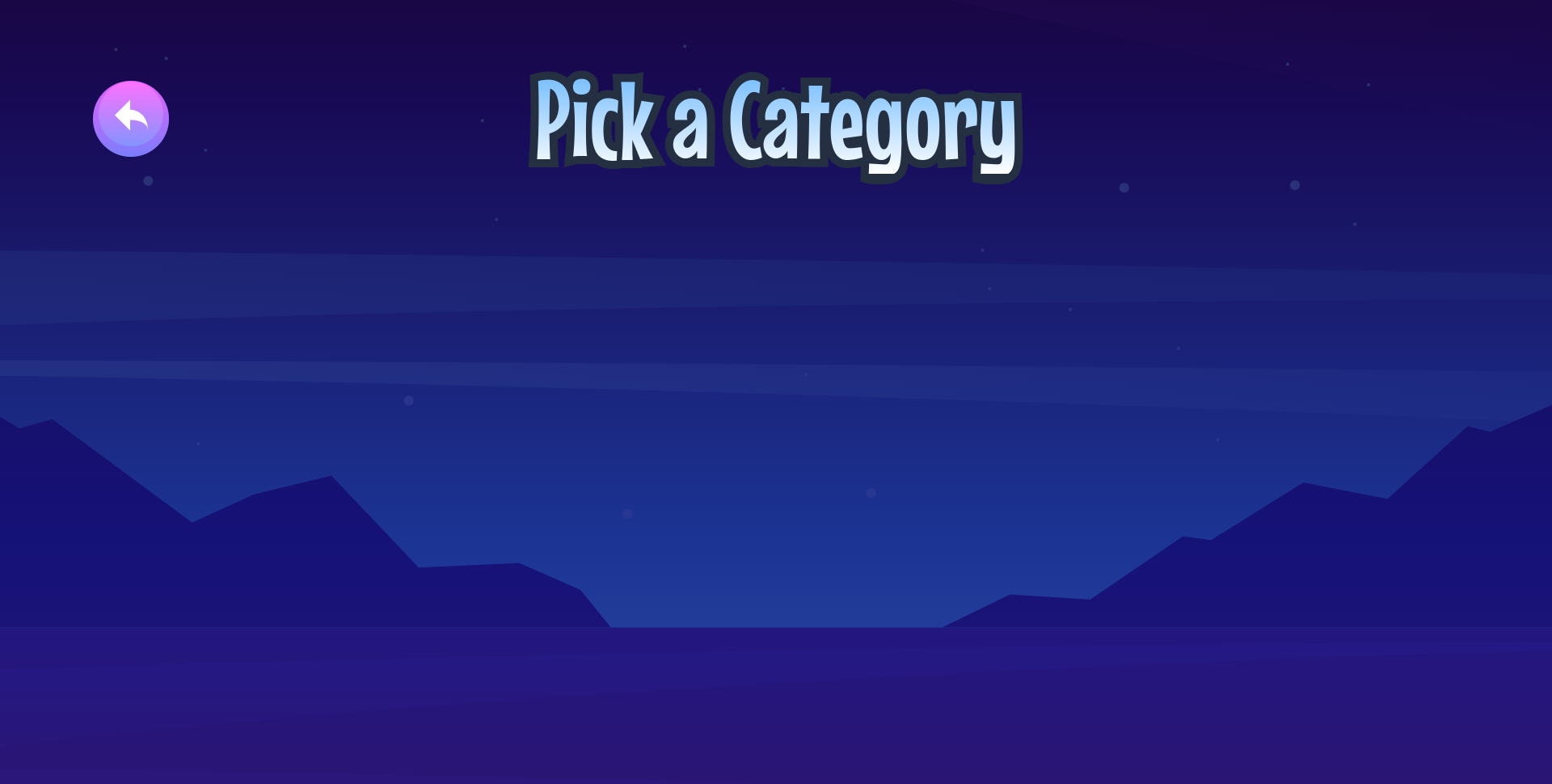
4 Replies
from just looking at ur code here: are you sure you want to
disconnect()
on each unmount/cleanup of that Cmp
-component?if you could make a minimal repro on http://playground.solidjs.com i can have a deeper look, but i m too lazy to git clone
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
removing
onCleanup(() => observer()?.disconnect());
does seem to get rid of the bugI just looked again at ur code. It worked a bit differently then I initially thought: I thought you had 1 global intersection-observer and then used this
set_observer
to observer.observe(...)
the element, but instead you are creating a new IntersectionObserver
for each element.
In that case you do want to remove the observer onCleanup I suppose and your code should work. Maybe it's due to the fact that with <div ref={set_observer}/>
set_observer
is also called during unmount (with value undefined
)... idk 🤷♂️ i would have to run the code to be able to see that.
I would personally go for 1 global IntersectionObserver and use observer.unobserve
to unobserve the element during cleanup/unmount.