I have no clue what I am doing wrong
Hello, I am very new to Solid, I am working with stores right now to represent this data structure:
I am using a store to store an array of these questions, and have an App component that renders them
I have no idea why, but each update I add a sub_question via the onlclick setQuestions, it updates the whole store to have the same values for each question in the question array, I have not found a way around this/why it is happenings, pls help. I have tried using a fixed index 0 for the setQuestions function, but this way still updates ALL the questions in the array each time i add a sub question to the fist item in the array. pls help
20 Replies
can you make a reproduction in the playground?
how do I do that, never used playground before
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
take the code u have and make it produce the same problem in there
ok
though one thing already stands out - you're reusing
default_question
it's possible that since you're reusing it, adding a sub question is adding it to that single question instance which is used for all the elements of the array
instead prefer to have a makeDefaultQuestion
function that returns a new object each timewill try
i did the playground thing btw, do you want me to send it as a link or smth
yeah plz
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
as a general rule if you can reproduce your issue in playground/stackblitz you'll be much more likely that someone will a) want to help and b) be able to fix your problem
thanks for the advice
also you're using
0
instead of i()
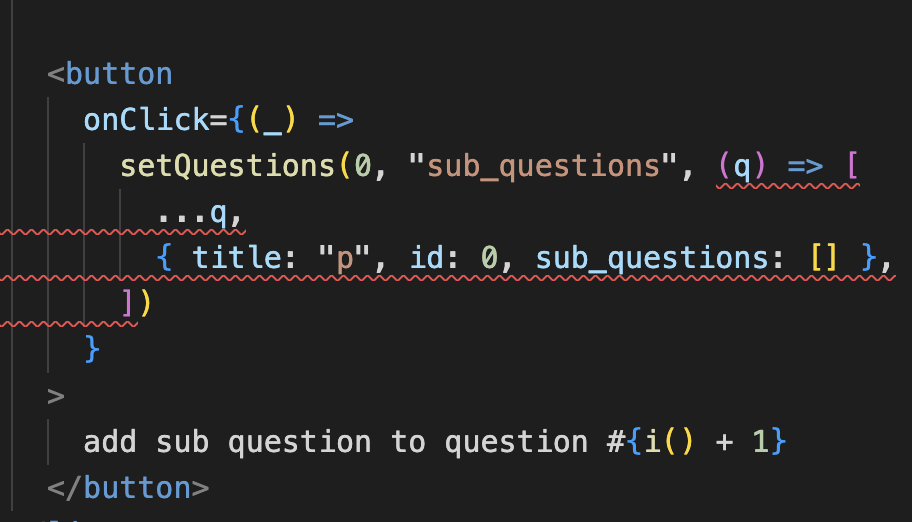
Yeah, anyways the issue got fixed by just making default_question a function, but I have no clue why that was causing a problem, can you explain it to me please?
when you create
default_question
as a single object, that same object is reused for all the entries of that array, so even though you may have 5 questions, they all actually point to the same object and so updating one updates all of them
using the function instead creates a new object instance each time, so each entry of the questions array is uniquedamn so under the hood Javascript just points to one shared memory adress where default_question is read instead of cloning it into seperate memory locations, that kinda sucks. well everything makes sense now thanks for your help.
yep, you have to explicitly clone the object using
{ ...default_question }
(which is only a shallow clone) or do a deep clone or just construct a whole new objectbtw you can make your life a bit easier and do this
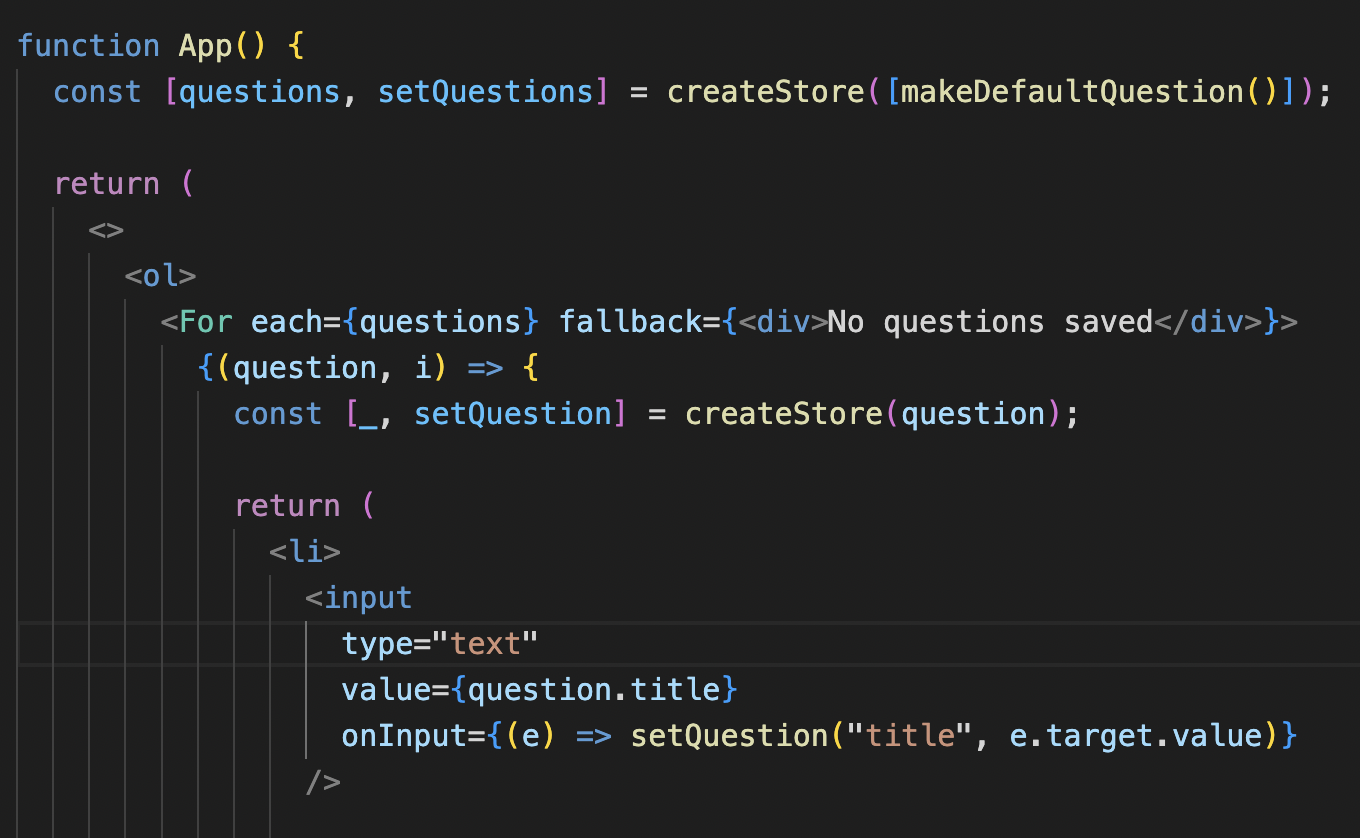
instead of doing
setQuestions(i(), "title", ...)
you can get a setter for that specific question and call setQuestion("title", ...)
same goes for sub questions or any other objects in a store
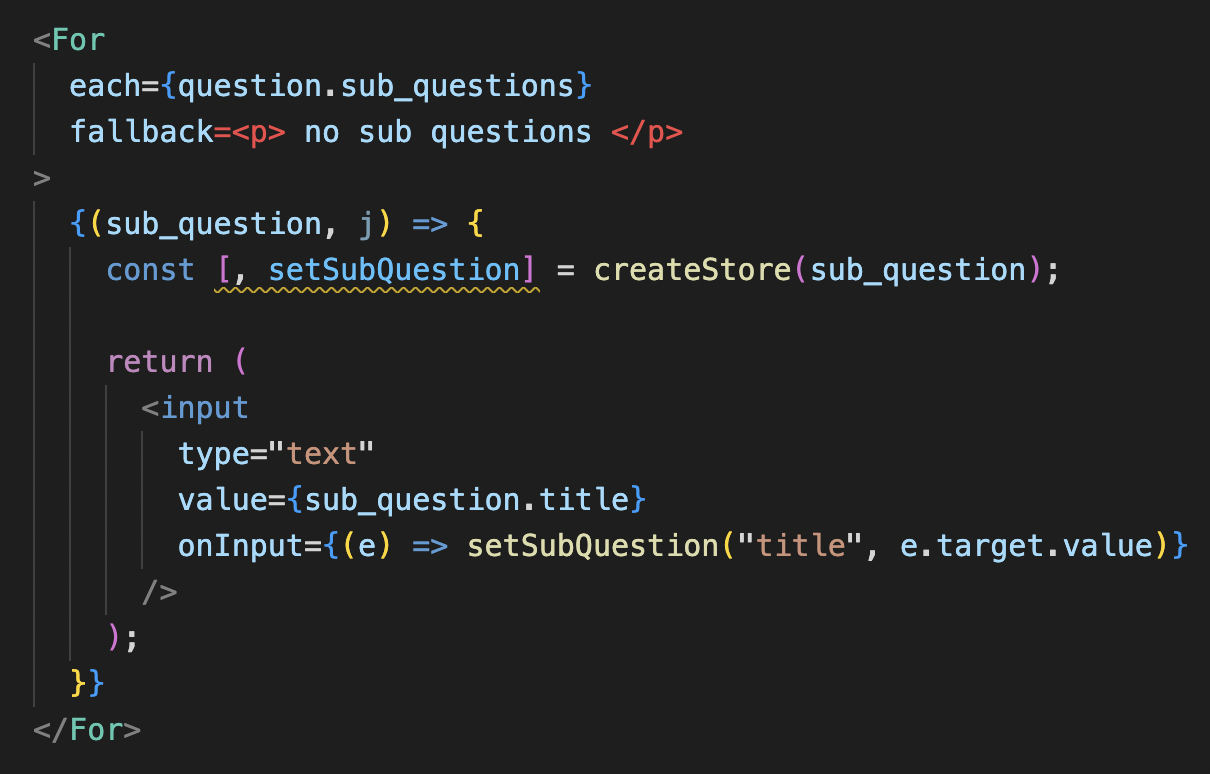
setStore supports paths
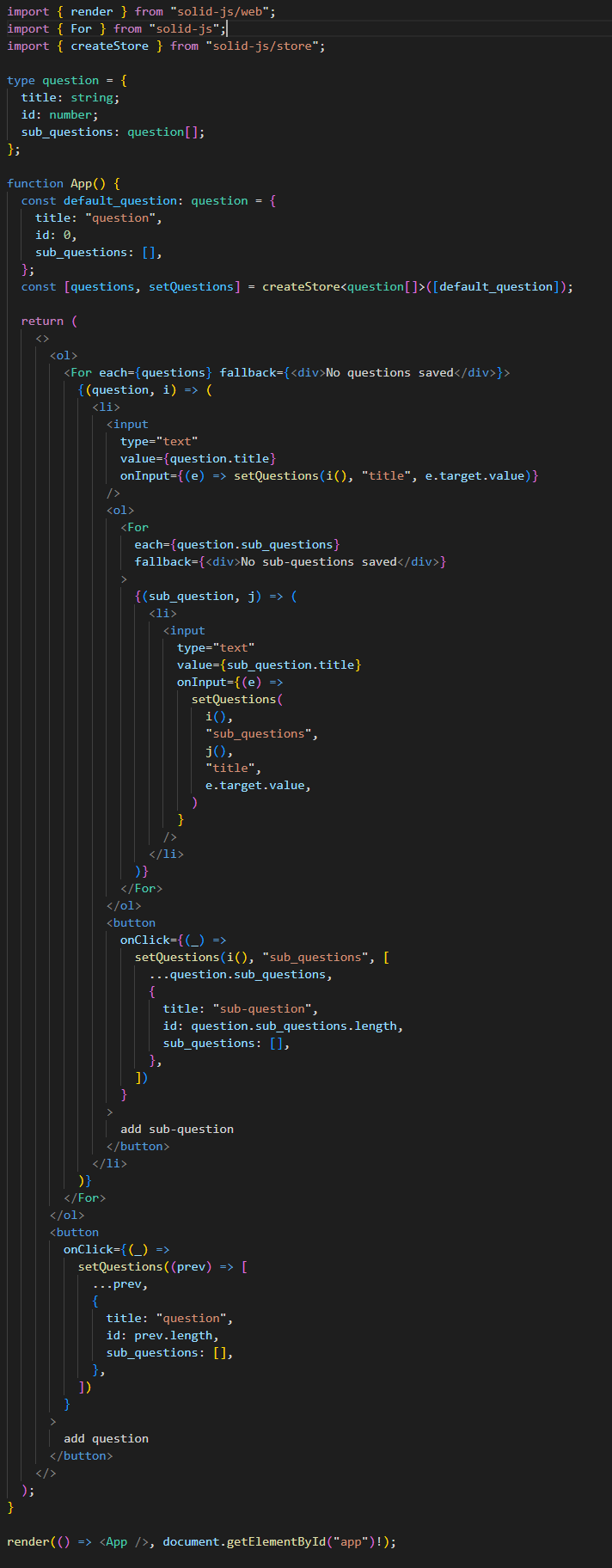