✅ Beginner loop
I need to make it so if the user doesnt enter an integer it gives an error.
ive done this but if they enter 'cat' the error only shows up for a a split second and then the program closes.
do
{
try
{
Console.WriteLine();
Console.Write("Input test score: ");
testscore = Convert.ToInt32(Console.ReadLine());
}
catch
{
Console.WriteLine("Invalid Integer... Press Enter To Exit.");
Console.ReadLine();
return;
}
if (testscore == -1) { Console.Write("Well done, Pres ENTER To Exit...."); Console.ReadLine(); Console.WriteLine(); Environment.Exit(0); } else if (testscore < -1) { Console.WriteLine("Invalid Integer. Please Retry"); } else if (testscore > 100) { Console.WriteLine("Invalid. Please Enter Your Test Score."); }
else if (testscore >= 0 && testscore <= 49) { Console.WriteLine("You need to put in more work!");
} else if (testscore >= 50 && testscore <= 79) { Console.WriteLine("Could you do better? ");
} else if (testscore >= 80 && testscore <= 100) { Console.WriteLine("Well Done!");
}
} while (true);
} } }
if (testscore == -1) { Console.Write("Well done, Pres ENTER To Exit...."); Console.ReadLine(); Console.WriteLine(); Environment.Exit(0); } else if (testscore < -1) { Console.WriteLine("Invalid Integer. Please Retry"); } else if (testscore > 100) { Console.WriteLine("Invalid. Please Enter Your Test Score."); }
else if (testscore >= 0 && testscore <= 49) { Console.WriteLine("You need to put in more work!");
} else if (testscore >= 50 && testscore <= 79) { Console.WriteLine("Could you do better? ");
} else if (testscore >= 80 && testscore <= 100) { Console.WriteLine("Well Done!");
}
} while (true);
} } }
43 Replies
$paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
Your error is possibly because of this $tryparse
When you don't know if a string is actually a number when handling user input, use
int.TryParse
(or variants, e.g. double.TryParse
)
TryParse
returns a bool
, where true
indicates successful parsing.
Remarks:
- Avoid int.Parse
if you do not know if the value parsed is definitely a number.
- Avoid Convert.ToInt32
entirely, this is an older method and Parse
should be preferred where you know the string can be parsed.
Read more hereConvert.ToInt32, etc just uses Parse.
Issue with parse is that if the format is invalid, an exception will be thrown and crash your program if unhandled.
TryParse will give a boolean whether it succeeded or not
alright so you i just remove the try catch?
if you use TryParse, yes.
Is there any way at all to do it with try catch?
You can still do it with try/catch, just that your catch should be at the end of your loop and it shouldn't have a return in it
yes,
try/catch
with int.Parse inside a loop will work fineso i put catch down the bottom but on top of the while?
wat
The code you have right now is really hard to read, too much repetition and nested statements
think of what must be repeated and what must not
any way u can make it easier for you
i*
well, what I'm trying to say is that this would be easier for you too, if you could separate what parts go where
ahh
What distinct "steps" could we separate your code into?
okay so basically the task is just depending on the number it displays a certain message
but i need to include error handling for incorrect data types
Sure
so step 1 would be reading an input and making sure its a valid number
yes
what would be step 2?
the message that it displays depending on the number entered
okay, maybe rephrase that to "print a message based on the score"
but yeah, it works
so, what parts needs to repeat?
the number that the user is entering
it needs to loop until -1 is typed in, then it exits
weird task but thats what ive been assigned
Okay, so the program as a whole repeats until -1
yes
I see.
Well, that explains your current code. With a few tweaks, this structure works.
But only because its a simple program - if it were to grow this would quickly become unmanagable
yes this is all i need to do
once i do the error handling its all done
You have only a single loop, the one for your entire program.
That means that if the user enters an invalid number, your entire program starts over
how I would write this would be that the "get and validate a number" thing would be its own part of the code, and it wouldnt be able to leave that loop until the user entered a valid number
yes
But you can keep it as is, you just need to fix some small issues - your usage of
return
specifically
entering an invalid number probably shouldnt stop the program
why not just say "invalid number, try again" and.. go again?would that be easier?
?
I dont understand the question.
ive done this but if they enter 'cat' the error only shows up for a a split second and then the program closes.because tahts what your code does.
yes so, if i enter a number its fine. but i need it so if user does not enter an integer it displays Invalid Integer... Press Enter To Exit.
that
return;
there means "stop this method"instead of doing that it closes instantly
even without the return the program shuts down
.. respectfully, no it doesnt
as soon as a type a letter and hit enter, it shuts down
could my visual studio be bugging
have you perhaps set it to do the really stupid thing of running the last compilable version of your code on errors?
uhhh how would i check that?
id hope not
okay, so i just cleaned and rebuilt solution
i did not know that was a thing
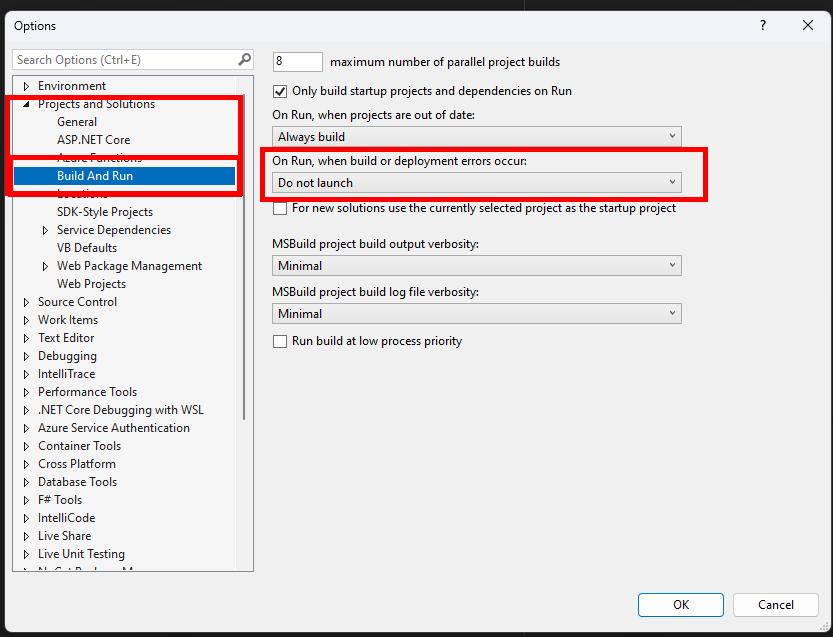
yep
make sure this is set to "Do not launch"
i have spent so long trying to fix this
and all i had to do was that
sorry about that
thankyou