How do I add strings to a list?
So what I basically want to do is something along the lines of:
Title.Add(Console.ReadLine());
I want to save string values and then display them in the program if the user chooses to option. My problem lies in that I have no idea on how this works. I've looked at the MSDN and YouTube videos but I just can't seem to grasp it.
Also, I've built up a menu using switch and cases.
The biggest problem of them all is that I have yet to find a good explanation on the difference between
List<string[]> Title = new List<string[]>();
and
List<string> Title = new List<string>();
Since this is assignment, I don't want the answer, I just want to understand how to actually use them and the difference...
98 Replies
the difference between the two is the same as the difference between
string
and string[]
List<string[]>
is a list of arrays of strings
List<string>
is a list of strings
vs
the way i think of it is a
string[]
is an array, and a List<string>
is a string[]
with more overhead (not correct at all, but might help with a rough visualization)You're right, not correct. True that a list has more overhead than an array, but it also has more functionality. It's dynamically-sized for once
So it's not like it's overhead for no reason
im just trying not to overwhelm them with a full background of a linked list
List<T>
is not a linked listwait, theres a type for that
nm
So, @Blane, does that answer your question?
Hmm. I think I understand a little better. So the List<string[]> is like a bucket full of lists consisting of strings?
While List<string> is just a singular list of string?
(Sorry for the late response. Had to go grab food.)
yes
["abc", "def", "ghi"]
can be assigned to both a string[]
and a List<string>
but not a List<string[]>
But is it possible to assign ["abc", "def", "ghi"] to a list, name that list and then assign the list to a List<string[]>?
You couldn't do that, no, because an array (
string[]
) is not the same as a List<string>
So this assignment I'm working on wants me to make a program similar to a blog, where I first type in a title and then the post/description.
I'm not sure how to go about this however since I barely knew anything about the difference between lists and lists[]
huh...
Hmm
Why do you need lists if you're just providing a title and a post/description?
That seems like a case for classes, not lists or arrays
i think he meant the variable name
I'm sorry if I can't describe it properly...
Then you could have a
List<Blogpost>
you can .Add()
more blogposts todo you mean the name you give the variable? or an actual property?
Let me read through it again real quick
Unless we're doing the good ol' "we won't learn classes yet, so use arrays as a a stand-in for objects" with a blogpost being a
string[]
where [0]
is the title and [1]
is the bodyI'll use google translate to help me out
"The blog should be a list that can save string vectors"
oh, so it is
List<string[]>
woopsEvery individual blogpost should be a string vector
and they should contain at least two elements
Oof, so I was correct, then
kudos
He added in bold text beneath: "NOTE: You may not replace the vector list with a class"
So, it seems like you would have a
List<string[]>
that is the blog, and each individual string[]
would be a blogpostso your situation would look like:
as ZZZZZZZZZZZZ said
Yeah
ya know, im just gonna make this more difficult
ill leave this for Z
bye
I appreciate you taking the time to help me!
Or, more aptly,
So, you'd have your
and you would be adding blogposts to it with
i think you just gave the answer to their assignment...
I'm not sure how I could've obfuscated this answer lol
touche lol
So
var blog = new List<string[]>();
is to make the bucket which I will add the blog post to?
A bucket of buckets
Yes
Hmmmm....
I think I'm starting to understand this now but there are some gaps
Yeah, it doesn't make much sense to treat each blogpost as a bucket of strings rather than an object with properties
But that's what the requirements are
Let's maybe distinguish them. Each post on the blog is a bag of strings
And the blog is a bucket of those bags
So the blog in this case is both the Title + Post?
Doesn't help that english isn't my first language
Yes, the blogpost is a bag that contains the title and the post
Waaaait....
Hold on
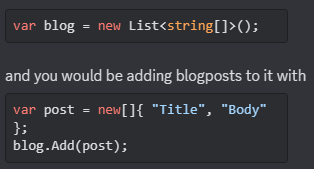
The second var post = new[]{ "Title", "Body" };
blog.Add(post);
What is the reason for the [] next to new?
To indicate it's an array
its shorthand
the full version is:
it makes writing code faster
But since both elements are strings, the compiler can figure out that it's strings we want to have there
So we don't need to specify it explicitly
Aaah... So if I were to write it, I would type it out like
string post = new string[] {"Title", "Body"};
yup
hmm okay
Then it makes more sense
Let me think real quick
string blog = new List<string[]>();
string post = new string[]{ "Title", "Body" };
blog.Add(post);
this is also dependant of which language version youre using.
if youre using .net Framework (not .net Core) then you have to manually change the language version
so using
new[]
(or new()
) would throw an errorThis would work, yes
I honestly don't remember which one I'm using
string blog = new List<string[]>();
would throw an error because youre assinging a List<string[]>
to a string
No
Yeah
would not work
As oke said
thats a separate issue, so still valid
Neither would
hmm
So to make sure I get it...
This is where I create the "bucket" or where I will give the myStringList its title?

I think what messes me up is the {} afterwards...
Also, I feel really dumb and sorry for taking up so much of your time
I'm very slow
using
{}
instead of []
is dependant on how you set the variable
im going to use the long version ({}
) in case youre using a different language versionSo when I have used lists before, we use [0], [1], [2] and [i] and so on.
this is a constant length, and will always be that assortment of values until its changed
what you want is this datatype:
You can
is equivalent to
Let's maybe skip some of the syntax sugar
oh, forgot to do that with the inner parts too lol
It's equivalent to
I think I can make it work if I figure out how to register what the user types as the title and blog.
The question is how I do that. I've tried with Console.ReadLine(); but I can't get that to work.
Console.ReadLine()
prompts the user for text in the console
you have to type text, then press enter for the application to get the stringoooh wait. I accidentally used the vector list instead of the normal one
There's no "vector" in C#, by the way
Just so you know
okay... so
List<T>
is a list
T[]
is an arrayLanguage barrier
based on the description,
List<string[]>
was the right oneYou know what. I'll just send you a screenshot
i was under the impression C#s
List<>
== C++s vector<>
It's the case 2:
Yes, but it is not called a vector
ohh, okay
You should create the blogpost array first
Then add that array to the list of blogposts
i dont think we'll be able to explain this properly without inadvertently answering your assignment for you
Yeah
Hmm...That's true
okay. Let's see. I now know (at least I think I do) what the difference between the <string[]> and <string> is
1. Make an array of strings of length 2
2. Read user input into the
[0]
th element for the title
3. Read user input into the [1]
st element for the body
4. Add the array to the list
string[]
is an array of strings
string
is stringOkay. That makes sense
List<string[]>
is a list of arrays of strings
List<string>
is a list of stringsList<string> liknar en shoppinglista där du skriver en vara per rad.
Exempel:
- Mjölk
- Bröd
- Ägg
- List<string[]> liknar en inköpslista där du grupperar varor i kategorier. Varje kategori kan innehålla flera underposter.
Exempel:
* Mjölkprodukter:
- Mjölk
- Fil
* Bröd:
- Rågbröd
- Vetebröd
- Använder du List<string> så kan du enkelt lägga till eller ta bort varor på listan. List<string[]> används om du vill ha en mer strukturerad lista med underkategorier.
aaaaaaah
List<string> - Enkel inköpslista
Denna kod visar hur man använder en
List<string>
för att skapa en enkel inköpslista:
I detta exempel skapas en lista inköpslista av typen List<string>
. Sedan läggs olika varor till listan med metoden Add()
. Slutligen loopar vi igenom listan med en foreach-loop och skriver ut varje vara.
List<string[]>
- Grupperad inköpslista
med kategorier
Denna kod visar hur man använder List<string[]>
för att skapa en inköpslista med kategorier:
Här skapas en lista inköpslista
av typen List<string[]>
. Varje element i listan är en egen array med strängar, som representerar en kategori med tillhörande varor. Vi lägger till kategorier med hjälp av Add()
och en ny array med varunamn.
När vi loopar igenom listan går vi först igenom varje kategori (kategori
), sedan skriver vi ut kategorinamnet. Inuti den loopen går vi igenom varje vara (vara
) i kategorin och skriver ut den.oke
REPL Result: Success
Console Output
Compile: 482.941ms | Execution: 41.809ms | React with ❌ to remove this embed.
oke
REPL Result: Success
Console Output
Compile: 467.845ms | Execution: 51.133ms | React with ❌ to remove this embed.
Thank you guys so much for the help. Was finally able to understand it now after some sleep. I really appreciate you guys taking the time out of your day to help me.
The Swedish explanation helped a lot!