✅ Configure base address in HttpClient in Dependency Injection
Hi! I want to add http client with configuration for the service
ILocationService
It has an implementation NominatimLocationService
where I get this http client. The problem is that I get default http client without configuration. What have I done wrong? Also is there a way to configure HttpClientHander
in Dependency Injection
? I need it to set automatic decompression
34 Replies
well but you are saying "configuration for the service
ILocationService
", not NominatimLocationService
ILocationService
is an interface for NominatimLocationService
that I use in controller to fetch location datayes, but you are registering custom httpclient with NominatimLocationService, not ILocationService
i don't know if that's an issue, but still if you are injecting NominatimLocationService via ILocationService then you should probably change one of the two
I tried registering for
ILocationService
and NominatimLocationService
and still getting exception
and this is when trying to make a call, not when constructing NominatimLocationService, right?
Yes I'm calling method and passing relative address
as of now i wouldn't know better than this
also because i have to go to sleep
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
fair enough
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
The problem is that in constructor I receive httpClient without baseUrl that I configured in
Program.cs
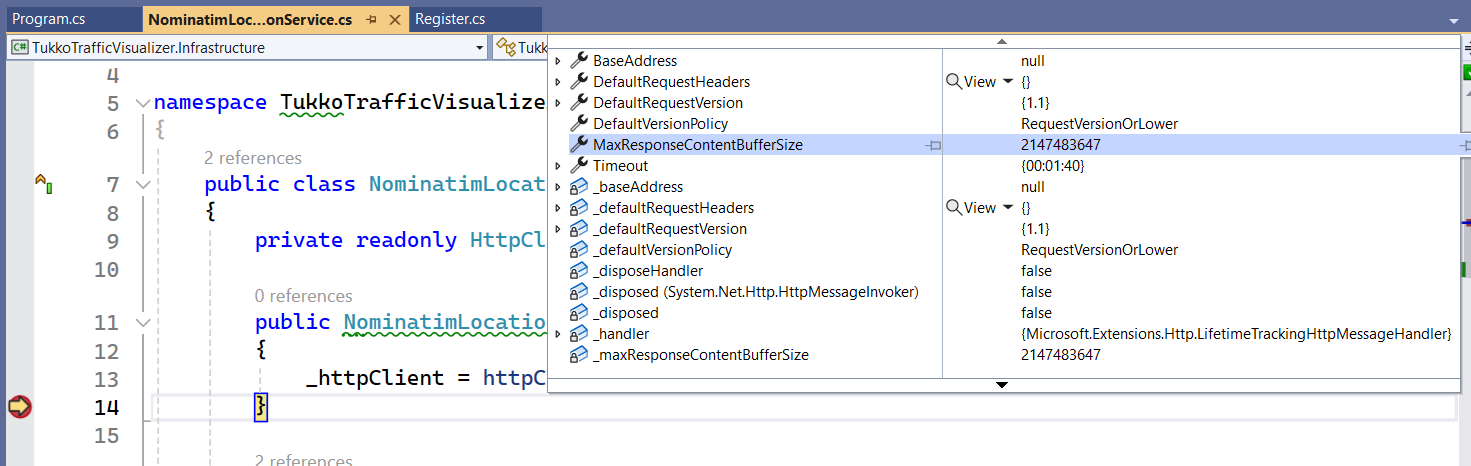
I tried the ways you suggested but they didn't solve my problem
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
I changed to this but base address is still null
If I set base address in constructor, everything works fine
afaik, your configuration here seems right, Maybe if you show the rest of your program/startup.cs there might be something weird going on there and would be easier to troubleshoot
Nonetheless, this is ok
As long as the HttpClient being supplied is transient, there's no downside
Microsoft does it in their examples:
https://learn.microsoft.com/en-us/aspnet/core/fundamentals/http-requests?view=aspnetcore-8.0#typed-clients
Make HTTP requests using IHttpClientFactory in ASP.NET Core
Learn about using the IHttpClientFactory interface to manage logical HttpClient instances in ASP.NET Core.
For the automatic decompression, use the extension method
ConfigurePrimaryHttpMessageHandler after you do AddHttpClient<>
return an action that instantiates the HttpMessageHandler with automatic decompression
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
eventually he could try starting from scratch to just try the httpclient injection
Program.cs
Search class
The place where I use search class
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
Because he mentions it in his post?

Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
Yeah, I attempted to reproduce the error here and couldn't get it too
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
Sorry I didn't answer earlier, here is the link: https://github.com/aleksandrmakarov-dev/traffic-visualizer
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
thank you for the answer and sorry for wasting your time, maybe error is related to my computer if it works fine on yours. If you have any experience with Redis, what .net library can you recommend? I get every minute >150 data items about road situation, item has nested structure and I need to delete previous data using prefix and save new items
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
do you mean create a new post in help section?
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
okay, got it