Object reference not set to an instance of an object
I'm making a simple UDP packet sender but for some reason I have this error when I attempt to start sending. which is "Object reference not set to an instance of an object. here is my code.
43 Replies
I am pretty sure it has to do with the "string ip Address = textBox1.Text" but I am relatively new and do not know how to fix it
You declared but didn't assign.
use "new()" keyword.
Next time, please show where the error occurs, you did not specify where the breakpoint hits / exception throws.
Also Why not radioBox ? It would fit your application. Don't bother with checkBox values
Also check for constructor(s) for UdpClient
I dont know, I like checkboxes, do they raise any problems?
k
yeah its happening because of the chekcbox
Where do I put that?
this code is quite messy
string ipAddress = textBox1.Text; in textBox1_TextChanged doesnt do anything really
UdpClient udpClient = new UdpClient(); in if (checkBox2.ForeColor == Color.Green) is only available in that if clause not on the else where you are trying to close the client
and what default wrote
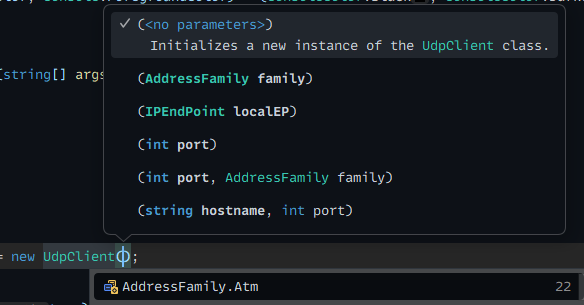
hmm, so how would I make it send when its green and not send when its not? Also I want to keep it secluded to like that checkbox if that makes sense
Check for constructors
like this?
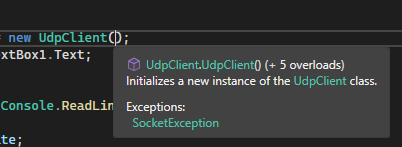
Yeah you should see from there
ah
how
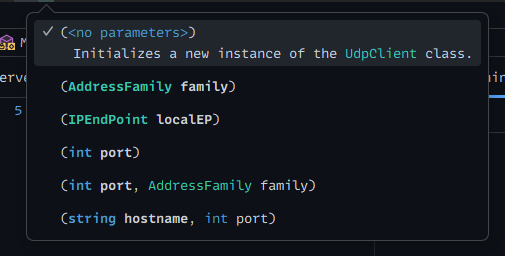
One of them accepts IPEndPoint right ?
So you can pass an ipEndPoint object

Like this
I personally hate the wrappers, both Tcp / UDP wrappers are useless
Raw sockets are way better
Yeah but it's easier to use/learn 😛
I have muy errors
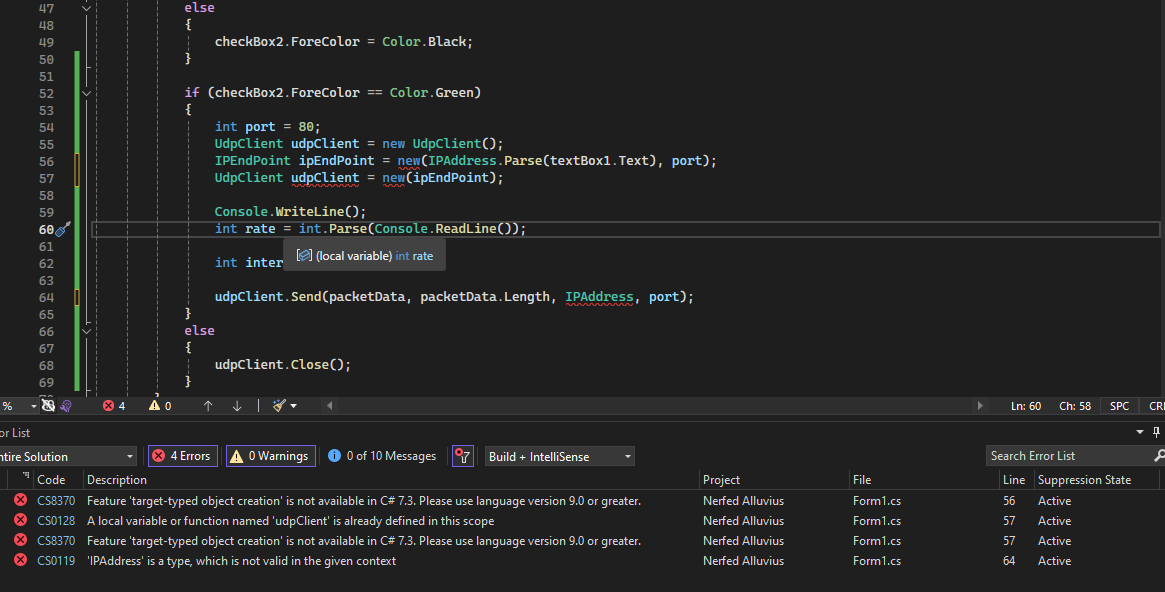

Your C# version is older so you need to specify the type when using "new" keyword
sweet, that fixed it. however
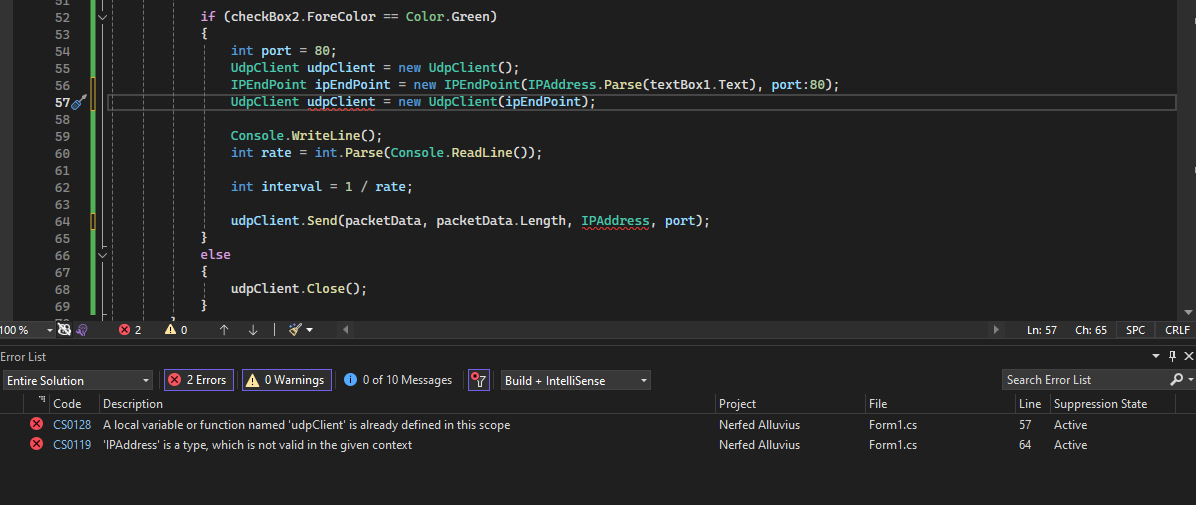
Just read it man 😦
They are indicated with red underline
ok
I read but do not understand
is a class not an object

It expects an object

this is an object
That you created with "new" keyword.
that makes sense
thank you
np 👍
sorry but 1 more thing
Just ask
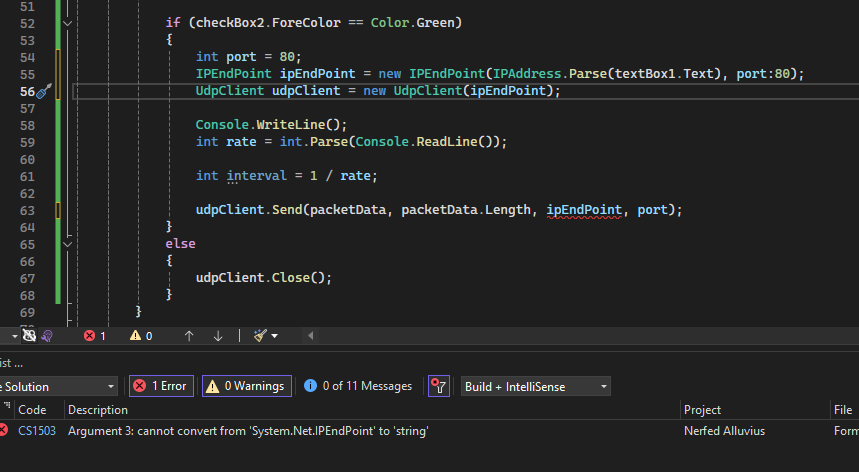
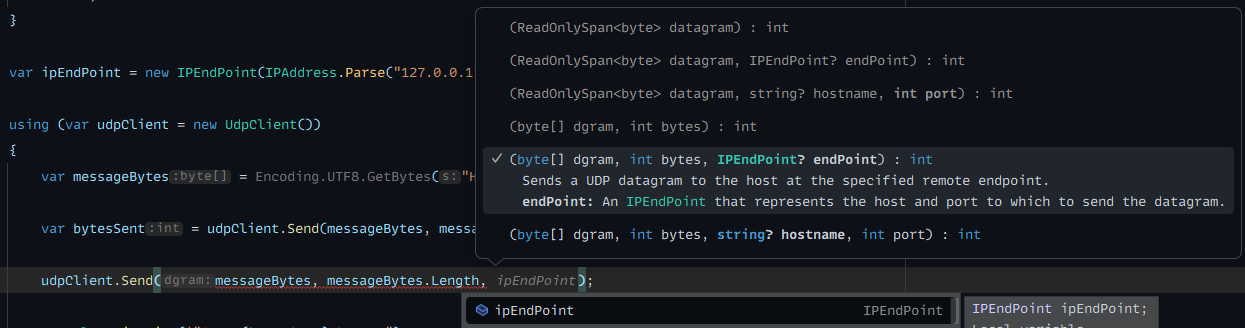
It expects either just endPoint or hostname with port as you see
But you are passing ipEndPoint object
which is not a string

Hover the mouse on object and you can see it's type
Which is "IPEndPoint?"
They're presumably using the overload listed below that
Yeah
I'm just explaining the fundamentals of IDE and logic behind objects
You can pass ipEndPoint without port
oh ok
yeah that makes some more sense now
thank you for help