Reading in a File C# 2D arrays
I have to code a program that will read in a 2D array of 9 x 9 integers from a file that represents a Sudoku board solution
My goals are to
1. Read in the file and display it on the form (it is a text file)
2. After this, I have to write a validator for the solution
Right now, I’m focusing on part 1 which is reading in the file
Usually, the goal when reading in a file is to do the following
(Writing code to select the file)
1. OpenFileDialog oFD = new OpenFileDialog();
2. if (oFD.ShowDialog() == DialogResult.OK)
(File selected so open to read it)
3. StreamReader oSR = new StreamReader(oFD.OpenFile());
4. int Records = int.Parse(oSR.ReadLine());
(Initializing the arrays)
5. Set array = new int [Records];
6. for (int i = 0; i < array.length; i++)
Array[i] = int.Parse(oSR.ReadLine());
This is the procedure I usually go through to read in a file
My issue is since this is a 2D array, I get the error cannot implicitly
(My code for this 2D array, I get an error when setting SudokuBoard to Records): https://paste.mod.gg/ttybylvutrvb/0
https://paste.mod.gg/gokonoflypqv/0( here is how I normally code it in)
Anyone have ideas on how I code it in to read in the file?
BlazeBin - ttybylvutrvb
A tool for sharing your source code with the world!
BlazeBin - gokonoflypqv
A tool for sharing your source code with the world!
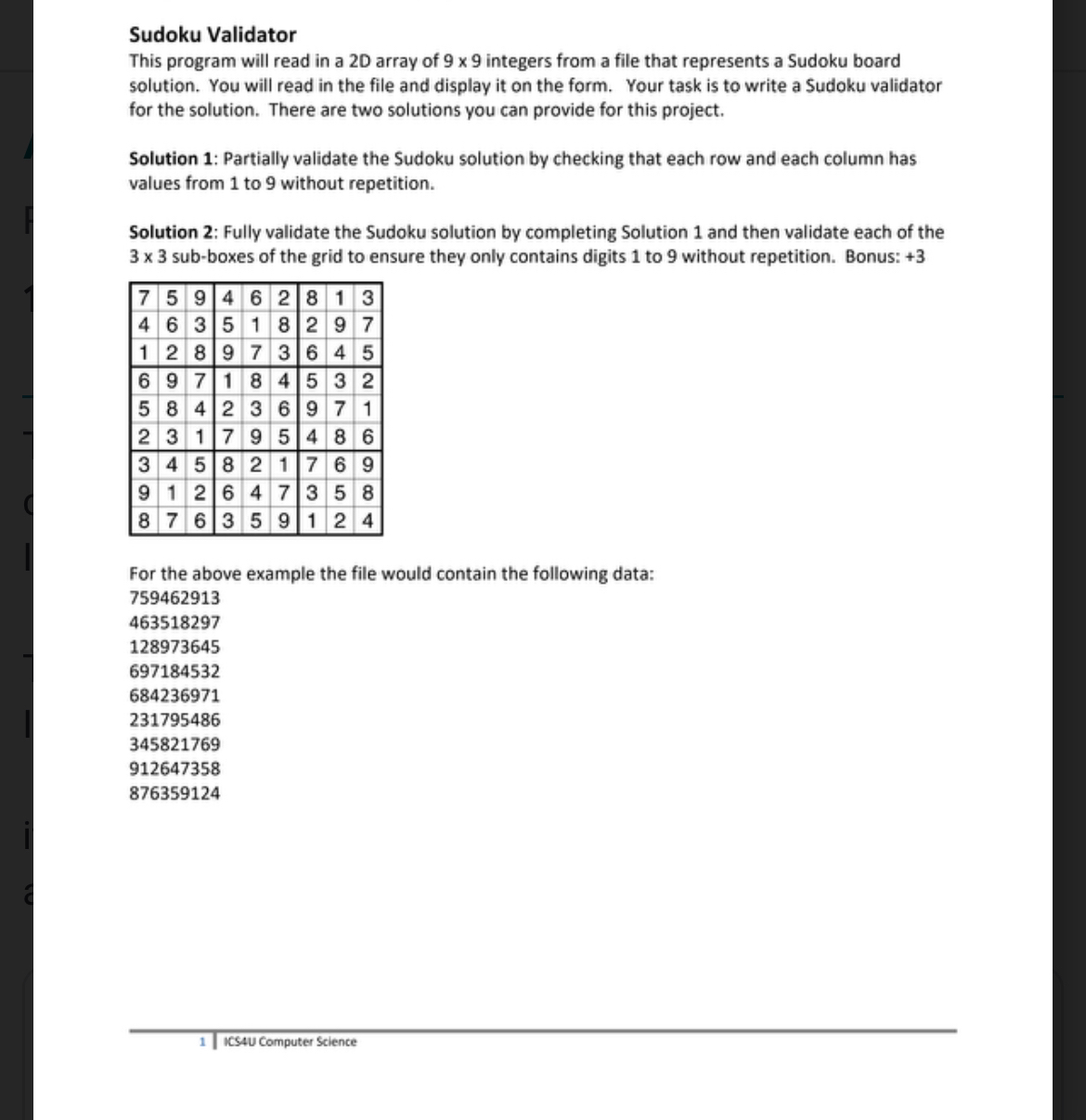
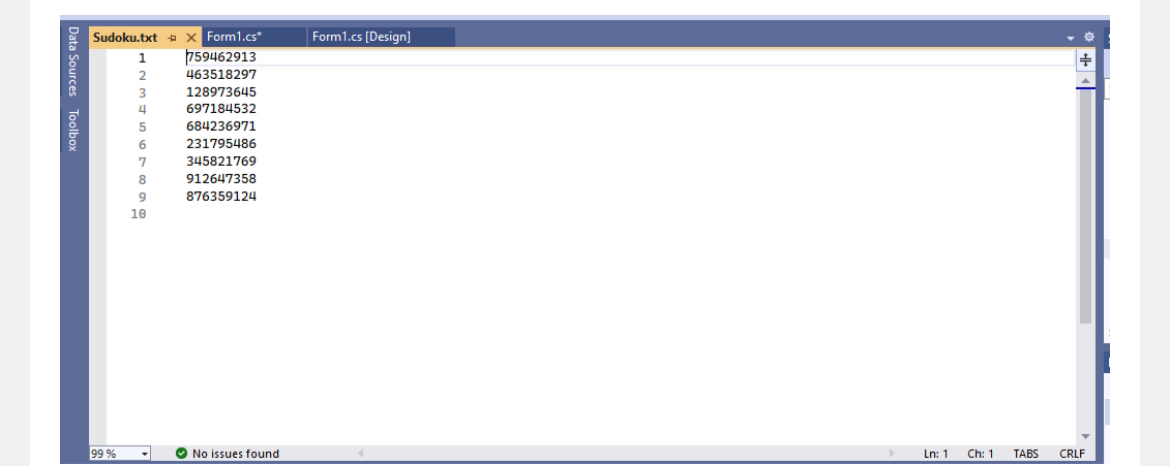
7 Replies
If it's a 2D array, you will need to treat the lines as one dimension, and number columns as another dimension
So, with an
int[9, 9]
array, go through the first dimension, and for each of them, go through the second one
In pseudocode:
Thanks for responding!
Can I ask, what do you mean with the for line_idx = 0; ?
The index of the line
Use the fact, that a
string
is just a char[]
under the hoodAngius
REPL Result: Success
Result: <>f__AnonymousType0#1<char, char, char>
Compile: 274.397ms | Execution: 76.017ms | React with ❌ to remove this embed.
Gotcha, I kinda understand this, I can use this to loop through (after I set it equal to records), my only problem is that I have to make it = to records and I'm not sure how to
I did something similar to that with the loops hough, it makes sense given it is a row and column
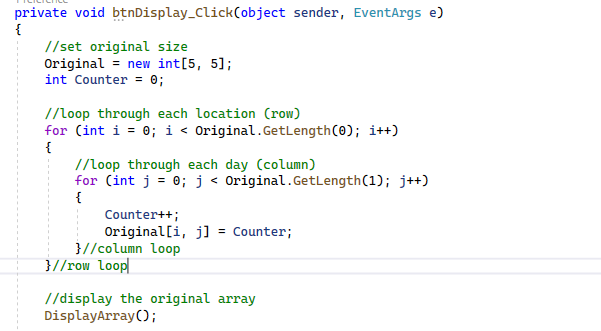
I'll try this out
I tried this out and my error was that when setting my array it couldn’t convert it to the single again
The same type of implicit error cane up
Or can anyone help me out