Reading in a File 2D Arrays
I have to code a program that will read in a 2D array of 9 x 9 integers from a file that represents a Sudoku board solution
My goals are to
1. Read in the file and display it on the form (it is a text file)
2. After this, I have to write a validator for the solution
Right now, I’m focusing on part 1 which is reading in the file
Usually, the goal when reading in a file is to do the following
(Writing code to select the file)
1. OpenFileDialog oFD = new OpenFileDialog();
2. if (oFD.ShowDialog() == DialogResult.OK)
(File selected so open to read it)
3. StreamReader oSR = new StreamReader(oFD.OpenFile());
4. int Records = int.Parse(oSR.ReadLine());
(Initializing the arrays)
5. Set array = new int [Records];
6. for (int i = 0; i < array.length; i++)
Array[i] = int.Parse(oSR.ReadLine());
This is the procedure I usually go through to read in a file
My issue is since this is a 2D array, I get the error cannot implicitly
(My code for this 2D array, I get an error when setting SudokuBoard to Records): https://paste.mod.gg/ttybylvutrvb/0
https://paste.mod.gg/gokonoflypqv/0( here is how I normally code it in)
Anyone have ideas on how I code it in to read in the file?
BlazeBin - ttybylvutrvb
A tool for sharing your source code with the world!
BlazeBin - gokonoflypqv
A tool for sharing your source code with the world!
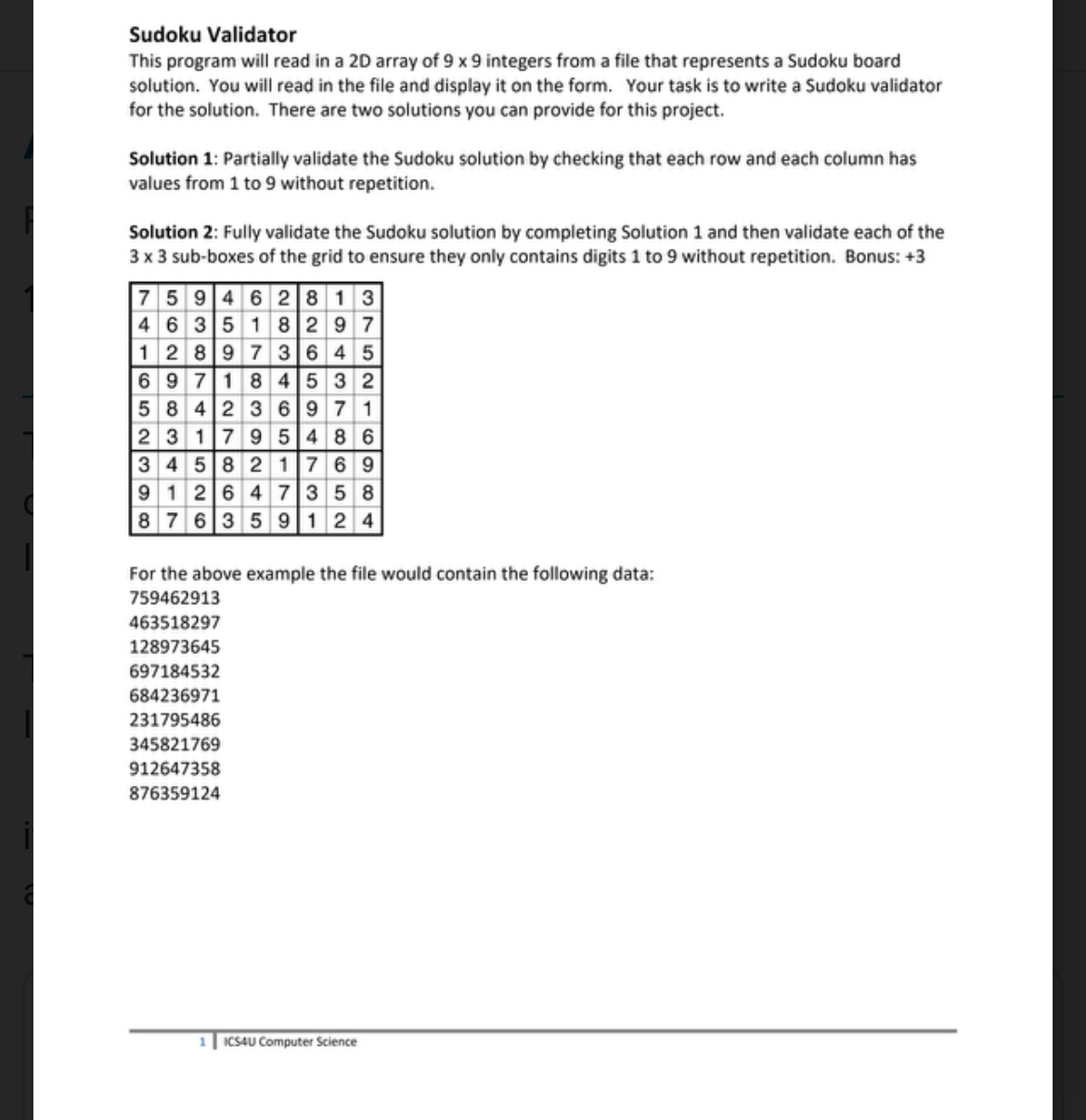
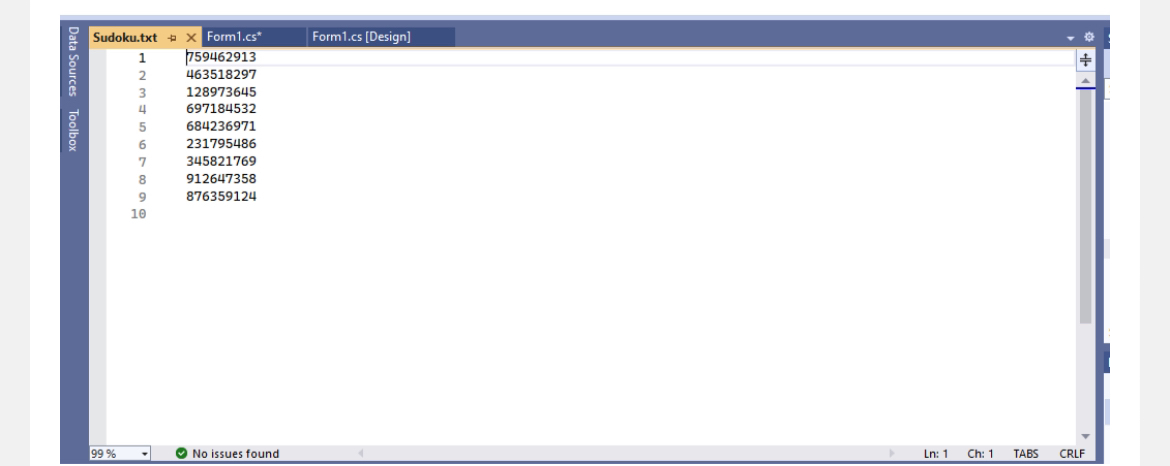
33 Replies
Is it an exam?
i swear i saw this same question asked earlier today
No, just an assignment
Array
I have to code a program that will read in a 2D array of 9 x 9 integers from a file that represents a Sudoku board solution
My goals are to
1. Read in the file and display it on the form (it is a text file)
2. After this, I have to write a validator for the solution
Right now, I’m focusing on part 1 which is reading in the file
Usually, the goal when reading in a file is to do the following
(Writing code to select the file)
1. OpenFileDialog oFD = new OpenFileDialog();
2. if (oFD.ShowDialog() == DialogResult.OK)
(File selected so open to read it)
3. StreamReader oSR = new StreamReader(oFD.OpenFile());
4. int Records = int.Parse(oSR.ReadLine());
(Initializing the arrays)
5. Set array = new int [Records];
6. for (int i = 0; i < array.length; i++)
Array[i] = int.Parse(oSR.ReadLine());
This is the procedure I usually go through to read in a file
My issue is since this is a 2D array, I get the error cannot implicitly
(My code for this 2D array, I get an error when setting SudokuBoard to Records): https://paste.mod.gg/ttybylvutrvb/0
https://paste.mod.gg/gokonoflypqv/0( here is how I normally code it in)
Anyone have ideas on how I code it in to read in the file?
Embed Type
Link
Quoted by
<@901546879530172498> from #Reading in a File C# 2D arrays (click here)

React with ❌ to remove this embed.
please don't repost
Ya I asked it but I didn’t get a response in a while, I thought I could post it again
My bad
Was worried it would get hidden since it’s been a couple of hours
Sorry about this
np, it just gets difficult to know what help you've already received if you're posting the exact same thing
Does anyone have any ideas on how to do this with a 2d array? Angius was doing a good job but he went offline
I think what confuses me here is that he never sets Records = to the 2d array, I usually did that before looping through
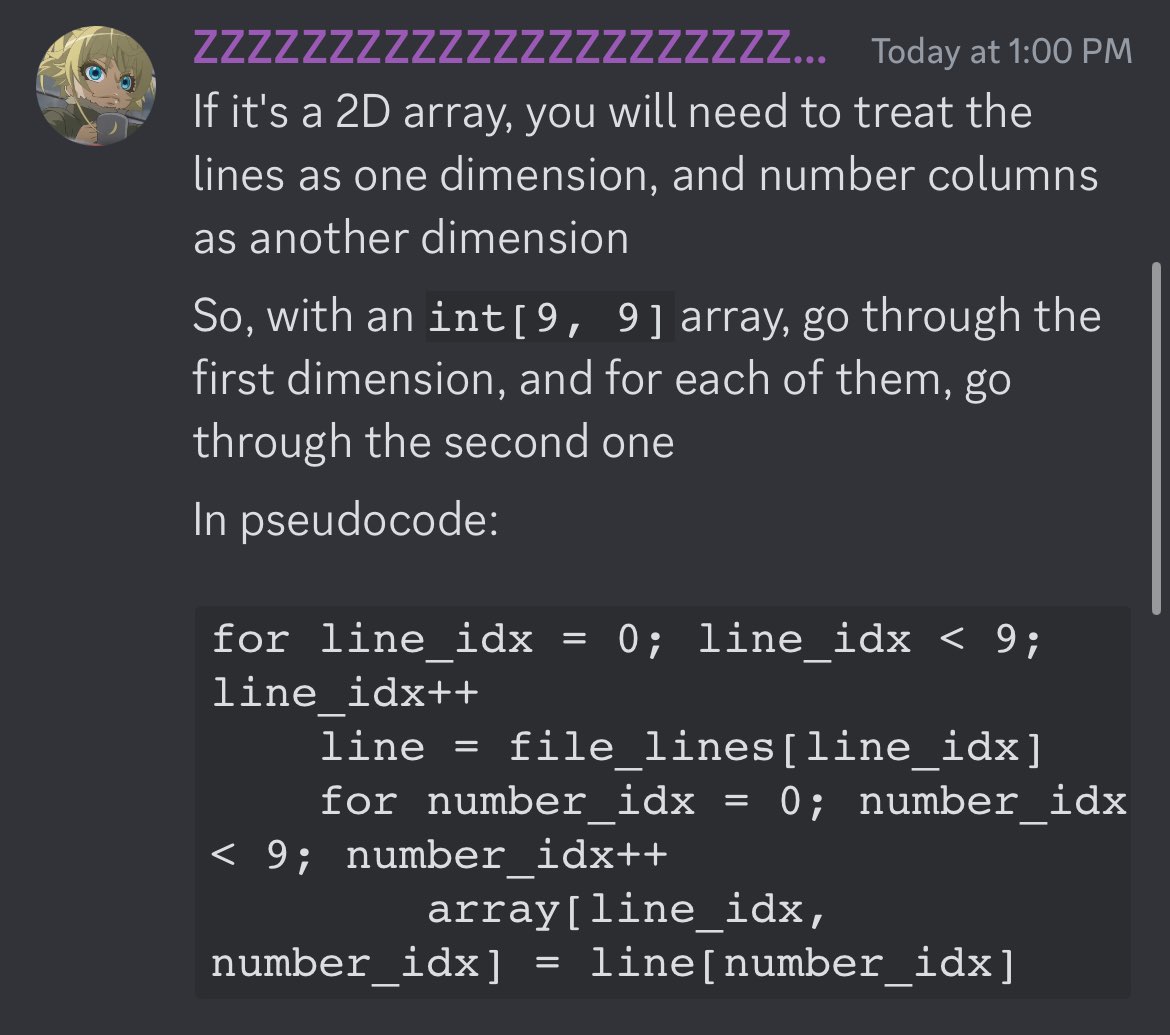
Well that pseudocode provided is for reading the lines from the file into the array, if that's what you mean
Yea I know, I’m just a bit confused on it, like for example, how do I have line_idx > 9 if it is a 2d array? Also for
The line = file_lines[line_idx], what does that mean?
So the for loop would be reading through each of these lines from your input file:
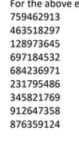
so
file_lines[0]
= 75462913Yea I know this, I’m just actually confused on what line = file _lines[line_idx] really means. If I make an array with Sudoku.Length > 9, I will probably get an error again right
Oh, do I have to code each individually?
no, that's what the for loop would do for you
Ya, I understand that (which is why I named my post reading in a file 2D arrays,) my next thing is actually coding this
I think I am kinda confused on what line = file_lines means
Your input file is made up of 9 lines
file_lines would represent those 9 lines
file_lines[line_idx] is a single line from those 9 lines
Would SudokuBoard.GetLength represent the line index ?
Also you realize the records variable i have, what does it actually do in my program
Sorry if these are basic questions just trying to gain some understanding
well StreamReader,ReadLine() in your input file would give you "759462913" as a string
so then you're doing
int.Parse("759462913");
which means Records = 759462913;
I kinda get this
I’m wondering though if file_lines means I actually like hard code everything
I'm still not sure what you think you'd need to hardcode. You read all the lines to start with into
file_lines
, then you loop through all those lines one by one, then you'd loop through the line itself and insert each digit into your 2d array. The for loops will "automatically" go through each item (line) inside file_lines
and each character in each line respectively
What part are you thinking may need hardcoding?I was mainly looking at this example and seeing how you Parse’d the first line, thinking I’d have to do it for each
I was just explaining what you were doing in your existing code 🙂
Right, really the main concern is understanding what “file_lines” actually is as a variable and like “line”
Well that's what I explained to you already:
You read all the lines to start with into file_linesOr you can still use streamreader and just read it line by line you might be getting too caught up on the pseudocode, pseudocode is there to provide a "high level" explanation of what should be done in your actual code i.e. you need to: - get the data from the file - loop through each line of the data - loop through each character of each line - insert each character into your 2d array
Hey, are you still there? Just got out of class now
yeah
(someone else can jump in even if the original person isn't around)
My bad! Sorry
So I’ve added the 2 loops as you can see here
My next step is to set Records = file_lines[line_idx], this is where I get confused though @SinFluxx
Like I’m kinda confused but what is meant by file_lines, like I somewhat get we have to set it to it, although I’m not sure exactly what “file_lines” is
Sorry if these are simple questions I’ve just been struggling a bit
ok so
file_lines
is just a hypothetical name you might give to a variable where you're storing the contents of the file. And to use that way of doing things you'd read the whole file up front, rather than StreamReader where you'd read the file line-by-line
i.e. you can use File.ReadAllLines()
to read the whole file into an array, and use the for loops as above to loop through each lineOh okay, so it isn’t applicable here where I’m using StreamReader this right
You wouldn't be accessing the lines from the file using an array if you're using StreamReader, no. Is StreamReader something you have to use?
Yeah
I can try asking my teacher about it
it's fine to use
so you'd instead need to do something like: