TcpClient being Disposed
I am pretty new to C#'s Dispose system, i came from JVM stuff so i'm pretty familiar with OOP.
Error:
Code:
8 Replies
its erroring from when im trying to send the handshake packet
you aren't disposing the client anywhere here, where is the code that constructs one of these objects?
this is the class that is creating the ServerClient:
i thought it was just because i was storing the TcpStream inside the class but i tried just method and idk
not sure why it ain't workin
works fine up until i try to connect aka sending the HandshakePacket
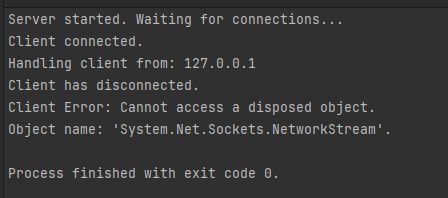
what code logs "client has disconnected"?
legit very messy but i just wanna understand why its doing this
wait-
maybe its breaking the loop...
one sec
it is 💀
thanks for the help, its disposing cuz im closing the connection
i'm so dumb
yep, i just had to send the handshake packet before i started listening for packets
:pepeOK:
fyi, TcpClient and raw threads are kind of outdated
Socket and Tasks are preferred instead
epic
ty