How do I implement this?
Hey, I'm struggeling a bit to figgure out how to implement what is shown in the attached gifs. Bascially I need a Coordinate System and want move content inside it at runtime with a mouse. The content should also be interactable.
The whole thing is to be used in a software program in which sports tournaments are to be managed. Here, fixtures are to be displayed graphically (mov) and assigned to corresponding games in the "game center" (GIF)
My Question is basically:
1. Is there already an Windows Forms element allowing or similar to this?
2. If there is nothing that is usable for this problem. How would I go about doing something like this and is it even possible? I don't need like a how to, but I don't know what to google for and read.
If you have any other idea how to tickle/avoid this problem, let me know.
Edit:
I forgot to mention that the toolbox has a "FlowLayoutPanel" but this won't be usefull here right?
I would be very happy to recive an answer.
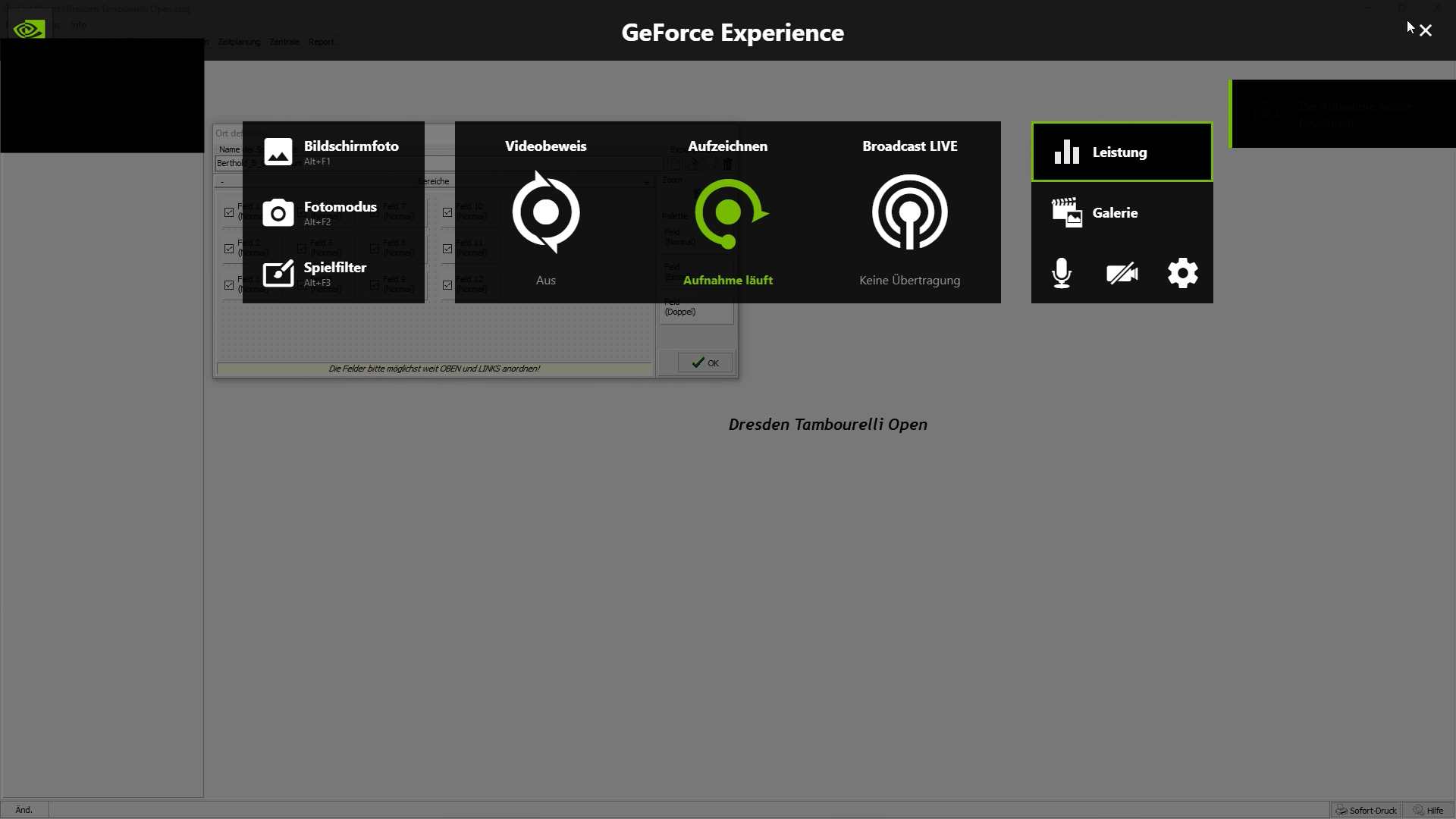
23 Replies
FlowLoyoutPanel
is an automatic layouter and controls the layout of all controls added to it. It is not relevant to you if you wish to move things around like this.
I don't know of any readymade control for this, but I wouldn't be surprised if there is a 3rd party control that can be used.First of all, thank you for your answer.
As a probably more experienced developer, is there some kind of Marketplace or Packet Manager that allows me to search for this specifically or can I just search in Nuget?
I have no idea, I don't do GUI apps
should i ask in #gui ?
I'd probably use google 😛
Yeah tahts a good idea
i would do that as well, but idk what to search for, cause i cant frame my problem in a search
alright, thanks again
Actually, come to think of it, iirc you can quite easily make any control be click-and-draggable.
and winforms layouting already uses an X/Y coordinate system
just make
MouseDown
and MouseMove
event handlers and attach all things you want to be draggable to themhm, thank I'm gonna try this
thats all the code for it.
oh wow, thanks
ngl. a bit shocked how quick you did that.
If I want to rasterize it a bit, I just need to round up the x/y Values of the Mouse point right?
hm?
oh, yeah
ok, sorry my english vocab is not the best 😄
no no you're fine, I just didnt expect it
ah sorry, one last question: to group them together its probably not enough to use a group box right?
hm, what do you mean by group them?
wait a sec
ok so, what you suggest works fine if you have like a button or label. But if I want to group elements together. Like move a label with a button at the same time, I need to probably calculate the position for the other object right? Or is there some way to like set the button as a child to the label, so it will move whenether I move the button?
Hm, yeah that might complicate things a tiny bit.
I tried with groupbox and panel, the problem is that you must click in the box itself, not on the child control
there is a workaround for that ofcourse
I will probably just work with a picture box. I think this will work.
alright, I feel like I now know a bit more to solve this problem myself.
I would like thank you once again for you help.
I figured out a workaround, if you're interested
yeah
ofc
the "workaround" is the if statement in
MouseMove
MakeDraggable
is just a helper method I use to make a control and any child controls it has draggable
if you want to be able to have groups within groups, you need to make the workaround if recursive
but that felt overkill for this demowow, thanks
how did you make the groupbox accept the input? It just gives me the MouseHover Event.