Self-Relation in Drizzle ORM?
I'm trying to create a folder/file structure in postgresql with drizzle orm
I have the following table:
but im getting this error in typescript
'folder' implicitly has type 'any' because it does not have a type annotation and is referenced directly or indirectly in its own initializer.ts(7022)
Sorry if I overlooked something, I'm new to drizzle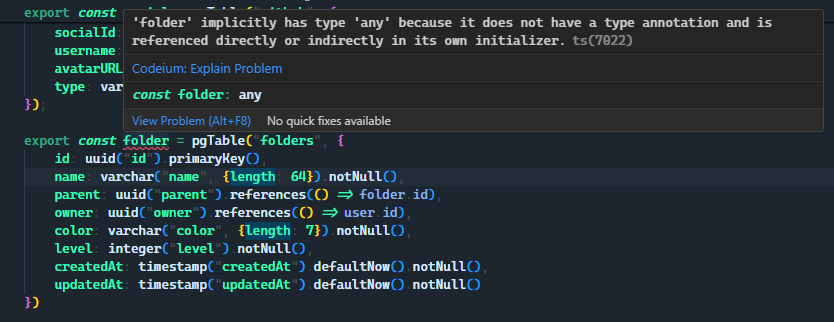
4 Replies
https://orm.drizzle.team/docs/indexes-constraints#foreign-key
If you want to do a self reference, due to a TypeScript limitations you will have to either explicitly set return type for reference callback or user a standalone foreignKey operator.
Drizzle ORM - Indexes & Constraints
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
thanks a lot, searched on google for self reference in drizzle orm and this page didn't show up idk why
Found it just by checking the section on foreign keys in indexes, but it's also showing up in the docs search.
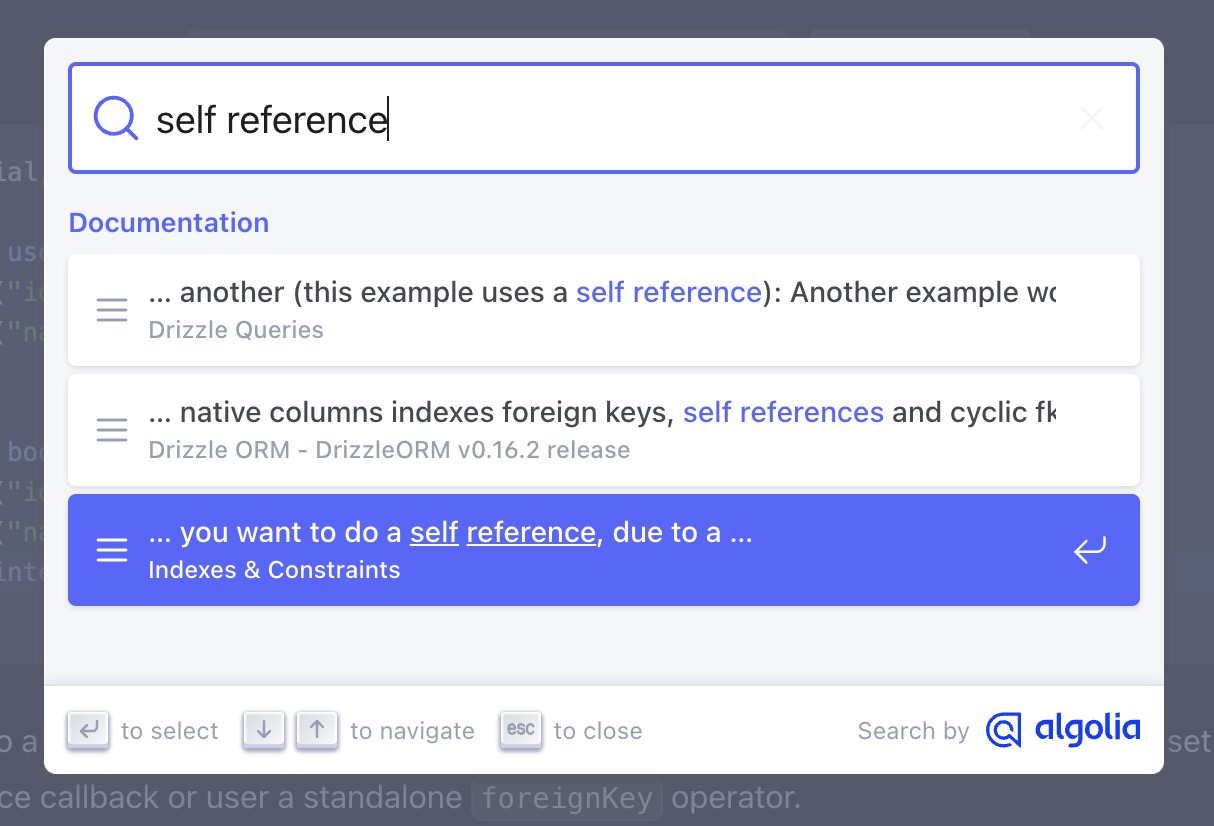
okay, thanks