IndexOutOfRange Leetcode help
Hey,
having an issue with my leetcode problem.
I know that its supposed to be a bitwise XOR operation but I tried a different algorithm as im not that advanced yet.
"Given a non-empty array of integers nums, every element appears twice except for one. Find that single one."
Error:Unhandled exception. System.IndexOutOfRangeException: Index was outside the bounds of the array.
75 Replies
which line gave you that error?
It doesnt show, im using the leetcode editor so :/
well that error only appears when you're trying to access the array out of its bounds
it also doesnt show me for which testcase its failing
eg if array's size is 5 and you tried to access element 6, u get this error
you can only pick elements from 1 to 5
Yea, chatgpt said it might be due to this line "var index = Array.FindIndex(nums, value => value != 0);" as it will return -1 if there is no element thats not zero
yeah no i wouldnt rely on chatgpt 100%
but there must be an element thats not zero
Yea ik but I have no idea what else it could be
maybe the arrays size is 0
And it worked for 5 testcases so the algorithm shouldnt be completely false
use Console.WriteLine to debug the array's size
well theres written "non-empty arrays"
alr
just test
3
this might be the problem
x is probably -1
then this
nums[x] = 0;
threw the error
or this too yea this is what chatgpt said
but why would it be -1
well it means it didnt find any number that isnt 0 in the array
debug the values of the array
alr
its [0,0,0] lol
how tf
How about step through it with the debugger?
wdym by that?
does leetcode even have a debugger?
Im wondering what happened if it would be [0,2,2], then the final array would be [0,0,0]
which would error
OH ok, I have no idea what is leetcode
let me have a quick scan of your code
leetcode is a coding challenge website
yep this is the issue, original array is [1,0,1]
you are zeroing your array with
nums[i] = 0;
but how can I solve it
what is this even supposed to do?
"Given a non-empty array of integers nums, every element appears twice except for one. Find that single one."
yes, is that wrong?
why are you zeroing the values of your array?
and this is your code?
every zero is an element thats double, so at the end only the single element is still there, all others are zero. Thats why at the end I return the element that isnt zero
Yes, but im aware that its normally done with a XOR expression but I tried doing it on my own
No linq?
no , this would be linq with the XOR expression, but I just read that online.
public int SingleNumber(int[] nums) => nums.Aggregate((s, a) => s ^ a);
i dont think you need to do bitwise XOR operation
just count the values, return the one whose count is 1
I'm sure it's just something like array.Single(x => array.Count(...
that sort of thing
GroupBy should work too
yeah
wdym count? How do I remember the ones before
like, how do I store them
well, there are many ways
like using dictionary, linq, or whatever
Given a non-empty array of integers nums, every element appears twice except for one. Find that single one
This to me just reads as, assume every number in some given array appears twice except for 1
no need to count everything to make sure, just find the thing which there is only 1 ofyea thats what I tried, how would you do it?
if you want to avoid linq, just use a nested for loop
or just use linq š¤·
But that wouldnt be much of a challenge

a very simple 1 liner
I usually avoid spoonfeeding
its not much of a challenge if someone does it for you
My bad, sorry I've just joined š
yea, im not submitting your solutions, just interested. I havent really touched LINQ before so i dont even know what "=>" means š
is it like an "if?
thats lambda expression
you could just use a nested for loop
Ah ok, linq is just a bunch of .NET methods to make things easier to read and write, reducing the need to write for loops yourself in this case.
and yes
=>
is a lambdaHow?
without linq, you could create exactly the same result by writing for loops yourself.
the
Single
is a method, which iterates
through everything in x
here. With this fact, you can start your code with a for loopOk so:
Iterate through the array -> then what? I cant just compare the current element to the next one right?
It also takes a
lambda
returning a bool, known in most languages as a predicate, a conditionI did it lol
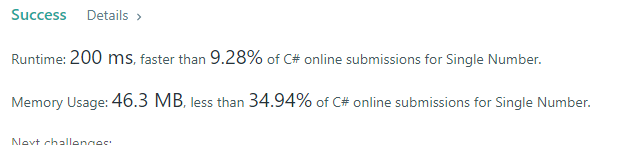
You can start by wrapping your own
Count
method
nvm then lolJust did this

That's a linq method
yea
I have used linq before but just simple expressions
I always use the => as an if
š Imma start to learn it properly tho
That doesnt really answer your challenge
you just happened to return 0, which is the correct answer
but if leetcode sent you another input, it may not work
no, it tests with hundreds of testcases
the issue in my code just was that when the single number is a zero, the logic breaks basically
but your elements arent all zero, so the variable will be false
they are cuz my code is setting every double int to zero, so just the single number will be left, which if its a zero would be an all zero array
so that means, you iterate from the start of
x
, to the end. At every iteration you need to know if this number appears once, or more. You could do this with a nested for loop. You'll need to count the occurrences of the occurence in the inner loop
Looking at your code, I don't think there is a need to use FindIndex herewhat do u mean by
double int
poor choice of words but every digit that appears twice lol
my code is a mess and overcomplicated ik
š
Beginning programming always is, don't worry š
alr, I will try to do it this way and see if the runtime decreases and at the same time its another challenge lol
If you can assume there is only 1 thing in an array which appears once, and the rest twice. You should be able to just have a nested for loop. The inner loop counting the occurences of the element targeted by the outer loop. After the inner loop, but still inside the outer loop, I think you could bail out if
==1
ooh
thanks
That's OK, there are, as R ^ said, many ways to do it. The cleanest is with linq but probably better to learn things like this without first
Thanks, this worked even better and way less code. Gonna try it with LINQ too.

No worries, I sketched out something like this; if you wanted to compare
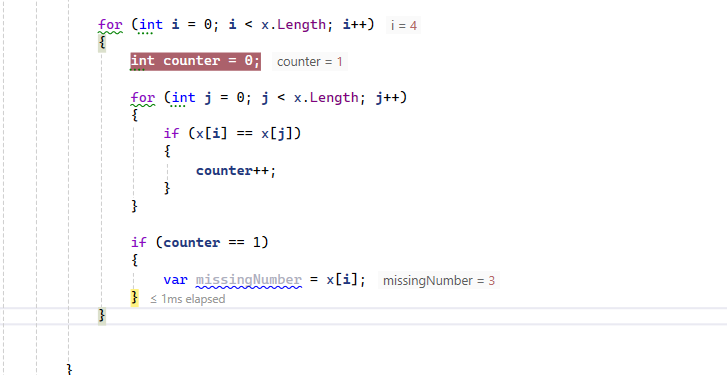
If you're interested in what's likely, or similarly going on inside those LINQ
Maybe not quite as naive, but effectively similar
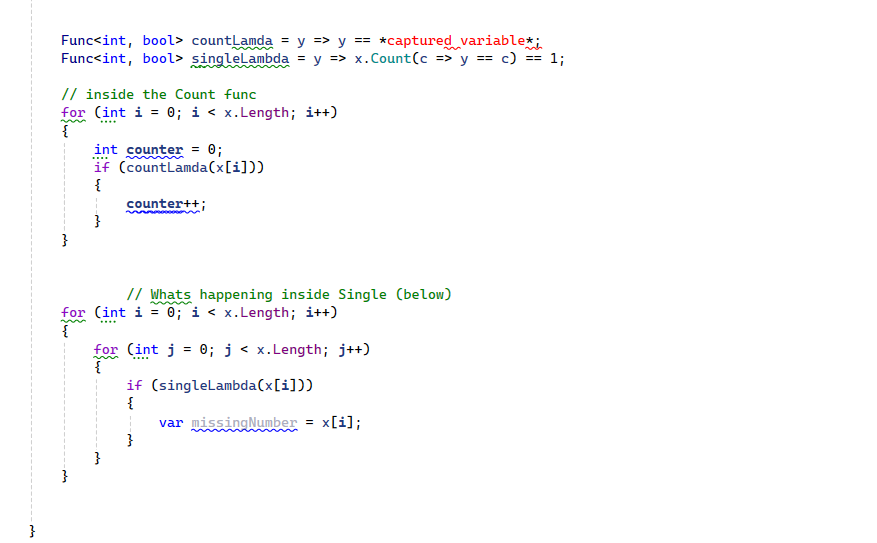
So actually, the for loops might be a little more efficient with 2 iterations instead of 3 here with LINQ.