Adding a form to a Livewire component
I added custom livewire component https://filamentphp.com/docs/3.x/forms/adding-a-form-to-a-livewire-component
gettitng error
Livewire page component layout view not found: [components.layouts.app]
Here is route inside web.php
i
Route::get('/admin/suppliers/{record}/line-items', ListSupplierLineItem::class);
27 Replies
Laravel
Components | Laravel
A full-stack framework for Laravel that takes the pain out of building dynamic UIs.
I want to use filament layout path?
What do you mean by "Filament layout path"? You want to use Filaments layout? Why don't you create a Filament Page then?
I created the filament page
but do not want to register with any resource as it has it's own permissions
That's a Livewire component and not a full page.
Why don't you just create a page via
artisan make:filament-page
?can I create a page without adding into any resources?
I want to give different permission to the page
as
resource
use canViewAny
and my page has different permissionYes, you can.
getting 404
Does the page show in the sidebar?
unfortunately no
Just noticed, you cannot auto register it anyway. Cause you require a url param. You should link to it from the table.
Also I think
/admin
is redundant, because your panel is probably admin which would result in /admin/admin/
urlafter removing
/admin
getting
Missing required parameter for [Route: filament.admin.pages..suppliers.{record}.line-items] [URI: admin/suppliers/{record}/line-items] [Missing parameter: record].
even I passing the recordWhere does that
$id
come from? Did you check that it’s not empty?yes, it's not empty
I did receive the $record on mount
slug creating the issue
https://filamentphp.com/docs/3.x/panels/pages#generating-urls-to-pages
How I can get the parameter value inside the page?
and I think it's a query base parameter.
will not work with path parameter
Where did you put that part of code and where is
$id
coming from? I wanted to know whether $id
is setinside AdminPanelProvider
What do you mean by "slug creating the issues" when you say that you received "record" on mount?
the error
[URI: admin/suppliers/{record}/line-items] [Missing parameter: record].
the record should be resolved as it's get on mount
functionHm. The method call and slug look good to me.
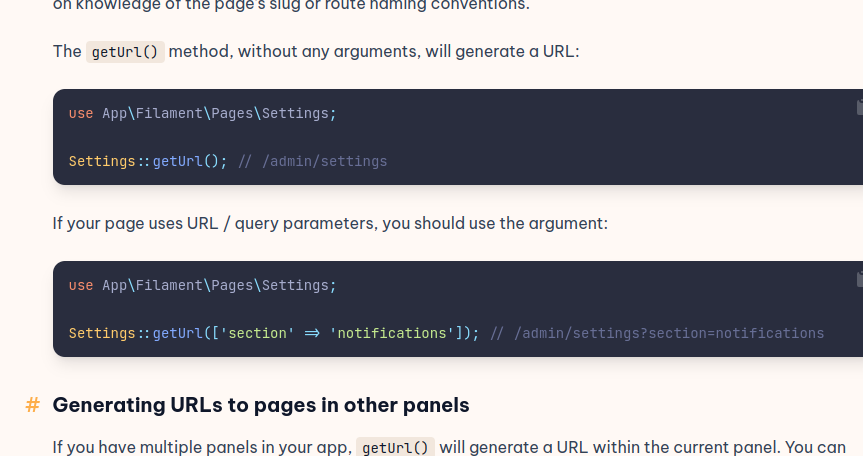
Where I can access the parameters pass to getUrl inside page?
Should I open an issue on GitHub?
I am still not sure this is a Filament issue
I don't know it's filament issue or livewire
Is there another way I can achieve the same functionality?