✅ WPF. Instance with the same key value for {'Id'} is already being tracked
I have two datagrids. They are filled with data from the database in this way:
49 Replies
there are also buttons that cause an error when pressed:
error:
Change
to
And use FindAsync first:
use entry to update the fields, it will be tracked
then savechanges
instead of instanciating a new order unless entry is null meaning it did not find that id
u should also not use the entities directly in your viewmodel / view code behind
u should use DTOs but I suppose this is a simple enough app to forgive that?!
✨
thx
$close
Use the /close command to mark a forum thread as answered
I updated the code taking into account the recommendations and it does save changes to the record, but when trying to create a new record, the same error pops up
normally u dont define the id your self its handled by the db unless u changed it to manual
so u wouldn't set the id
also u can move the SaveChanges outside the foreach u dont need to save per entry u can save at the end
// Update the entity using its primary key
// Set the properties of the new order entity
is Num your Key?
what is your entity like the class
oId its key
ah my bad I missed the oId
so u dont set the oId when u instatiate it right
+
do u have a builder instructions Order in your context?
it should by default assume Id is the auto increment primary key by entity rules
can u post the error message when u create a new instance?
are u using sqlite or some other db engine
sqlite
do u have any tool to open ur sqlite?
I wonder if its not updatingautoincrementing the key
I added the auto-generation id, but did not update the context in the program itself. Now I have done it and it has removed this error, but new records are still not saved.

db browser
u want to check if your column was properly created like that
so your Id would have INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT next to it when u collapse the table
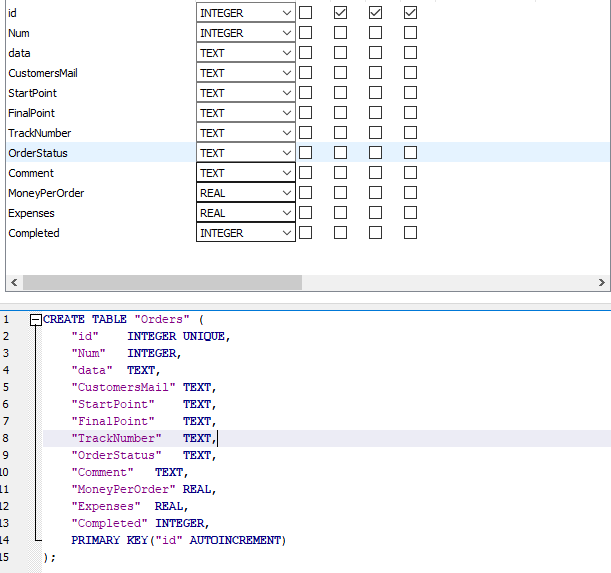
u dont need unique but u do need not null
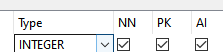
aside from that it does look like it wrote it right
in your DbContext u dont have any for modelbuilder right?
modelbuilder?
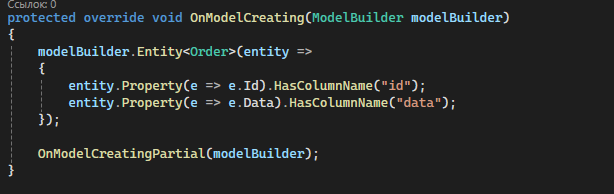
mmm I wonder if that could be screwing it
I dont really see anything wrong with your code aside from it
what should happen is
when you update, it will find if the db have an id already in it, grab it and whatever property u modify entityframework will track and update only those
and when u create a new one if u dont define the Id, it should make the database handle it and auto increment an id for it
but in your code for some reason its considering 0 as existent id
so maybe delete the row with id 0 in your db
other than that I am out of ideas here.
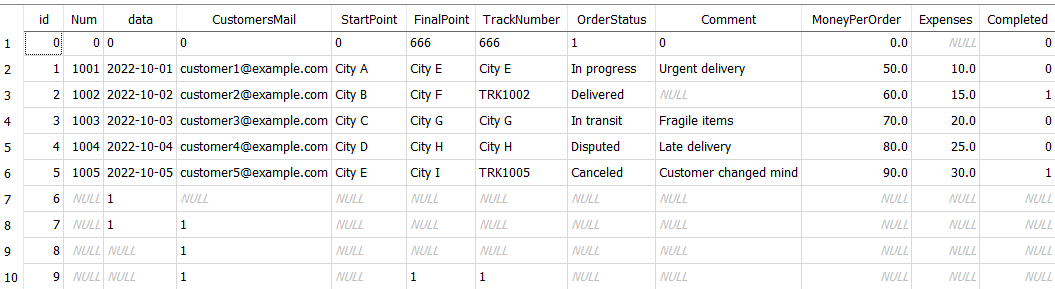
u ahve some weirdly null rows
these are the very records that I added. Then it turns out that the problem is already with displaying them in the program
if its a test db I would nuke it
and start fresh
u shouldn't be allowing rows with null ids
that shouldn't even be possible in first place
I overwritten the database and reconnected it to the program
ok did u remove all the bad rows from the table?
or emptied it?
I have banned an empty id. Do you think it's better to prohibit creating rows in which most of the columns are empty?
no u can have empty columns u cannot have empty ids
by the create table u have
i.e.: INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT
it shouldn't even happen in first place
I guess for some finik reason the nulls were screwing new entries maybe
so basically as long as ur column is properly create in the db
it shouldn't happen
as you can see its just a infinite loop that if I hit y it adds a new entry when I hit n it exits and saves it
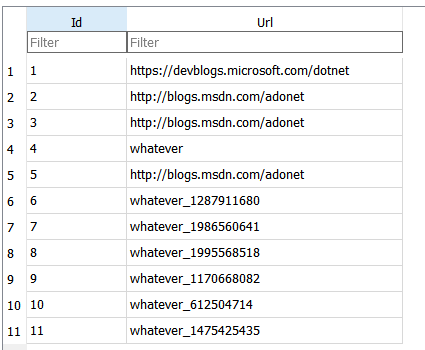
As soon as I overwritten the database so that the id was not empty, the code started working. But because my program displayed records with Completed = 1, and I created records where Completed = 0, so I thought they were not being created
I see
glad its working then.
its the id properly updating now too?
yes

🙂
thx again <3
no worries u can also do this
if its a property that must be filled
u append required to it and it will warn as error when u dont provide it
