33 Replies
I've been trying threads but they only run once for some reason
code in a thread has to be in a loop if you want it to keep running
threads are relatively low level,
Task
s are an abstraction over themHandleCommandInput should not be recursive, it should just have a loop in it
ohh
otherwise you're going to hit a stack overflow because it keeps calling itself
I thought Console.Readline() would stop the rest of the function from executing
it does until a line is received from the console
that's unrelated
I mean I used it as a recursion break
besides that not doing what you think, i don't think you want that anyway
don't you want to handle commands until the program exits?
yes ofc, the input stuff works properly for me, my main problem is that the Server thread runs only once
hold on wrong ss
started debugging:
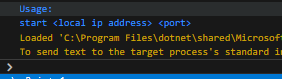
entered the command "start"
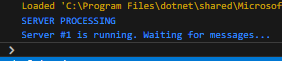
the SERVER PROCESSING runs only once
where it should run continously, on another thread
are you sure that's the case and it's not getting stuck somewhere in that loop?
but the Console.Readline() works fine
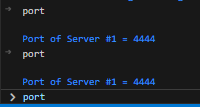
hmm
because i don't see it print out the second message after receiving data
the only problem could be this line "while (!token.IsCancellationRequested)"
otherwise the loop worked fine before I started trying all this multi-threading mess
idk I'm just so lost
can't find anything close to this in google
have you set breakpoints in that code to make sure it actually gets through one iteration of the loop?
I made it while(true) and it still runs only once
I mean it prints "SERVER PROCESSING" only once
but have you actually determined it's running once to begin with? or is some code in there blocking forever?
yeah, so it's never finishing the first loop
because you would expect to see that other message print out too
are you sending data to that endpoint?
only receiving data
well if you're not sending anything to the program it will sit there and wait
but not actually receiving any data rn
oh wtf
also, this is a really unreliable way to write network code
you're using UDP but don't seem to have any reliability or framing implemented
so there's 0 guarantee that a single call to recieve will give you all the data you're expecting and in the right order
it's explained in the docs https://learn.microsoft.com/en-us/dotnet/api/system.net.sockets.udpclient.receive?view=net-8.0#examples
yeah, I'm just testing and learning networking, but I'm calling the Console.WriteLine() before the doing the networking stuff
ohhh
do yourself a favor and use TCP instead
before you run into other issues caused by using an unreliable protocol
can't, making a game server
I must use udp
why does that matter
says who?
the protocol you choose should be based on actual performance and reliability requirements, not "it's a game"
for rapidly sent informations of the game, such as the player's position, I gotta use udp due to its less latency
are you writing a game networking system from scratch?
because that is significantly more complicated than you might think
basically trying to yeah
yeah, client interpolation, buffering all the data
etc etc
I didn't know that receive() method was stopping the execution, thanks