β JSON Deserialize Derived Class
Hi, i'm struggling to deserialize my object with the Newtonsoft.Json Librairy. I've a collection of
Step
named Steps
which can be a Stepspecial
or Stepnormal
. I'm trying to write my JsonConverter to deserialize the collection of Step
but when i try to deserialize i keep getting the stack overflow error because jObject.ToObject call back ReadJson
:
Any idea how to solve this and write a good ReadJson
method ? i've thought about the populate
method but not sure this is the good way of doing it.45 Replies
can you $paste the json?
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
BlazeBin - cgqvpondvcnu
A tool for sharing your source code with the world!
well it seem that i solved my problem just by adding this setting ....
JsonSerializerSettings settings = new JsonSerializerSettings { TypeNameHandling = TypeNameHandling.All };
but i do not really uderstand why and if it's the good solutionyou should avoid Newtonsoft unless you have an edge case that forces u to use it.
System.Text.Json is the way to go these days.... with your data your modesl would look like this for STJ https://paste.mod.gg/wifpvbzpkwvj/0
BlazeBin - wifpvbzpkwvj
A tool for sharing your source code with the world!
no need to write any corverters either
unless I missed out something from your json
why do we avoid Newtonsoft ?
thanks for the solution i'll try to do it this way
it's pretty much obsolete with STJ
and doesn't perform as well
because its inefficient, slow,
the same people that created newtonsoft made STJ
Given the number of its popularity, I thought it was a good librairy
newtonsoft was made in the acient era where there was no json reason reader and there was a wide range of dirty json in the market that needed handling
ok good to know
its popular because it existed when nothing existed
so that is years until .net core came out
do i really need to specify the name of each property for the json ?
but the performances alone should speak for it self.
u dont if they are the same except casing
I do because if I need to change it later for whaterver reason
I dont need to change the underlying variable name or vice-verse if the naming in the json
i understand thanks i will quiclky try this and let u know if everything work well π
I was trying to find the benchmark they did comparing both but I couldn't find lol
If you're already deep into Newtonsoft with things like custom converters switching to STJ might not be trivial
i've got the same problem as before, when i deserialize the array of step, it only use the class
step
and ignore the subclass stepspecial
and stepnormal
that's good i've started yesterday so i can still change from newton to STJSTJ can have some behavior thats unexpected, but generally reasonable. If you're serializing to an interface, only the members of that interface will be serialized.
here is the good class : https://paste.mod.gg/pymisjadpkel/0
BlazeBin - pymisjadpkel
A tool for sharing your source code with the world!
You should totally use the built in JSON class.
Pretty sure someone else already said that tho
I think the problem, as I understand it, is that you have
Step[]
as your target, but want to include derived classes as a part of the resultyep that's it
I don't know if that's something trivially done by System.Text.Json. If you try to deserialize to
Step[]
, you're going to get an array of Step
only.i want to have stepnormal and stepspecial instead of only step
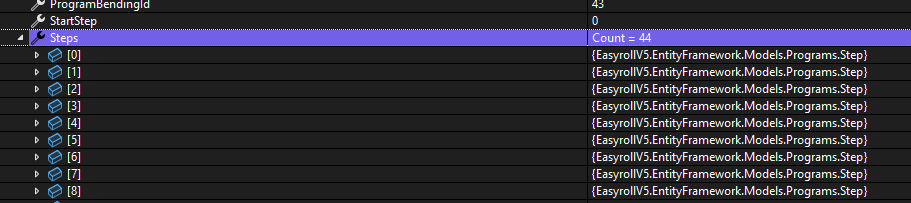
Now, I'll assume you are not planning to allow typenamehandling
yup and i can't really figure it out how to say to STJ to select the good class when i want to deserialize my object which contains this list
JsonDerivedTypeAttribute would probably be what you're looking for, if .NET 7+ is your target
it seems like the issue with this is the data itself, it does not include a type discriminator field
You could take a look at https://github.com/dmitry-bym/JsonKnownTypes if you're going to stick with netwonsoft
GitHub
GitHub - dmitry-bym/JsonKnownTypes: Simple way to serialize and des...
Simple way to serialize and deserialize polymorphic types for Json.NET - dmitry-bym/JsonKnownTypes
so you have to infer what C# type it is by the presence/absence of properties or simply make a single model with all the potential properties but marked optional
but you probably want to add a descriminator field
As far as your current converter is concerned
take a look at changing
StepSpecial? stepSpecial = serializer.Deserialize<StepSpecial>(reader);
to StepSpecial? stepSpecial = jObject.ToObject<StepSpecial>(serializer);
i'm open to switch to STJ, i just need to figure it out how to handle the desesrialization of my polymorphic object
do you have control over the json schema?
I think you're recursively entering the same serializer by trying to deserialize the derived type.
what do you mean ?
yup and with newtonsoft i've found the solution how to do it, rn i'm looking to find how to do it with STJ
are you creating this json or is it coming from a program you can't change?
y i'm creating the json
How to serialize properties of derived classes with System.Text.Jso...
Learn how to serialize polymorphic objects while serializing to and deserializing from JSON in .NET.
to resume i want to save programbending as a json in order to export/import it from a usb
do this
perfect that was what i was looking for ! π
Well i should be careful about my vocabulary it would have save some time i think π
polymorphic object
/derived classes
Thank you all for helping me !