Receiving Game Controller Input while Minimized (WinForms C#)
Pretty general question here. How would I go about receiving input from my user while they aren't tabbed into the program or have a different window as their foreground window? I'm using the InputSimulator Nuget package (InputSimulatorStandard version), and I'd like for my program to send inputs to a game window when it receives controller input. My only issue, now, is that the controller input isn't received unless I have my application as the foreground window.
This timer runs when a checkbox is
on
, and is stopped when that checkbox is turned off
. It functions fine, but I can't receive said controller input unless the app is opened, which kind of ruins the whole point of this functionality.
I've experimented with the use of GetAsyncKeyState()
, but as far as I know, that wouldn't work for a controller.26 Replies
Did staff approve?
I remember seeing the same thread where they said to contact staff to validate that it does not break ToS
Yes they did. Here is the proof, now we can help!
https://discord.com/channels/143867839282020352/1233614811523452981
@Mustafa Where is the
Gamepad
class from? Which package?Don't gui windows receive input messages from controllers, etc?
So you can just hook into wndproc
I don't think they do when not focused, but I could be wrong.
I believe they do
yeah they did
Windows.Gaming.Input
@Mustafa I'd look into https://learn.microsoft.com/en-us/uwp/api/windows.gaming.input.gamepad.fromgamecontroller?view=winrt-22621
Look at the C# example.
RawGameController
seems to be what you're looking for.Alright thank you, I'll take a look
Some people talk about using P/Invoke to hook to the OS directly, but apparently that could trigger anti-virus and other stuff since it's "less official"
yeah hooking to the OS would probably cause a lot of problems for anyone with an antivirus which I'd rather not deal with
Hooking the window messages would be ideal. Significantly less trouble.
Moreso window messages of the process, not your program.
Hooking into roblox?
If that is allowed by the ToS
Nah, Roblox's anticheat doesn't allow you to hook processes to roblox
It falls under their cheating policy as far as my knowledge goes
I see.
I assume your program is for controller support, or am I wrong?
it's basically just a program that allows for more freedom of roblox utilities and customizations. I've had a lot of requests to add options for controller players to zoom in/out, so I thought it'd be a good idea to add it
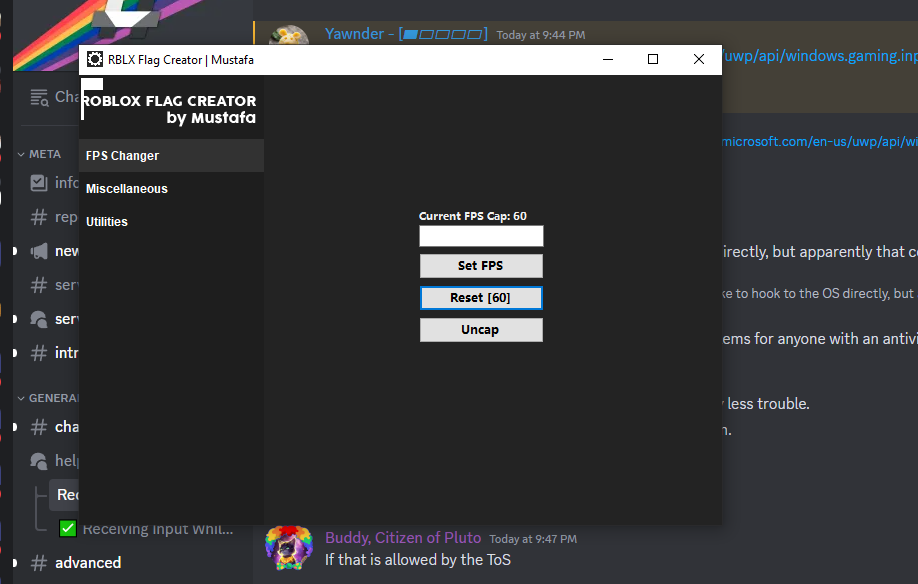
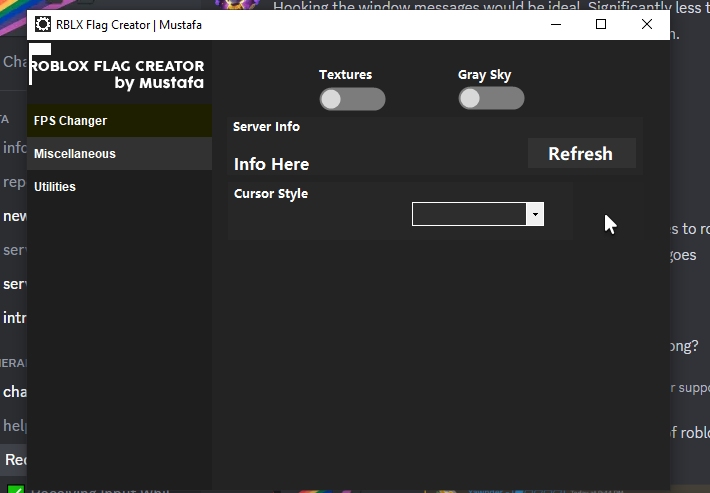
Ah, I see. Then messages doesn't really matter then.
my other features are just edits to roblox files so i'm not actually attaching anything to the roblox process
yeah
I just assumed you wanted to "convert" the inputs.
My apologies
Nah, no need to apologize. I appreciate that you're helping
The way roblox works right now, the game that my program is mainly designed for doesn't have a good zooming feature, and it doesn't map any dpad buttons to zoom
So I thought it would probably be convenient to just make it so that dpad up would zoom in and dpad down would zoom out
You can use Spy++ to check whether your program receives inputs in background, if it does then great! Otherwise you might have to use a wrapper
Spy++ is included with the installation of VS
Alright, thanks. I'll look into that as well as the solution provided above by Yawnder and see what I can manage to get.
Should be in the Tools directory of your VS path
@Yawnder - [▰▱▱▱▱] I implemented the method used in the article you sent me. While the input does work, it still only registers when I have my program as the foreground window, whereas I would need it to work while I'm minimized to achieve the functionality I'm aiming for.
@Buddy, Citizen of Pluto My program doesn't receive input in the background
The issue isn't my program as a whole but rather the method for receiving controller input
the controller input is only received when i have my program as the foreground window