Running SQL statement manually for in-memory database
I have an in-memory sqlite database:
and I'm having trouble running migrations on that database
14 Replies
this is the output from
await db.run(sql)
:
this is my only migration file, created by drizzle:
other tables are omitted
the drizzle error:
@opaldraggy
so, the output from this:
is:
I'm not sure if DDL statements are included on the output, but it doesn't seem to be failing... so, I'm not sure what's going onI suspect that you need to
split
on --> statement-breakpoint
and run any non-empty results as separate statements.got it. I'll try running only the users table creation to see what happens
also: drizzle can make it a little hard to track down the actual sql errors; so if you can find a lower-level interface to run the sql, you might get better debugging info (like directly using libsql)
Aside: you may want to remove your email from being shared above. Depending on how much you like being spammed by bots that harvest emails from various public places.
yep, that's exactly the issue, removing everything but the user table creation from the migration did the trick... now, how can I split those statements into multiple files?
or... do you have a better idea how to tackle this problem?
duh... I literally just:
thank you so much for the help @opaldraggy
I recently found out you can also "push" the entire schema to the DB at once instead of running individual migrations -- but I'm not sure whether that's exposed as its own API; and tbh, I personally trust it less.
But mentioning in case it's useful.
I've seem something like that in here:
import { pushSQLiteSchema } from "drizzle-kit/api"
but I did not find any docs on thatI think it's ~ documented here: https://orm.drizzle.team/docs/drizzle-kit-push
Drizzle ORM -
push
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
I had some issues with just splitting the migration file by
--> statement-breakpoint
, some table creation scripts are out of order, like the first image (creating first the n:n
table than the 1:n
table).
this is the new alg:
it mostly works, I'm able to insert data into most tables, but even so, there are still some tables that are not being created: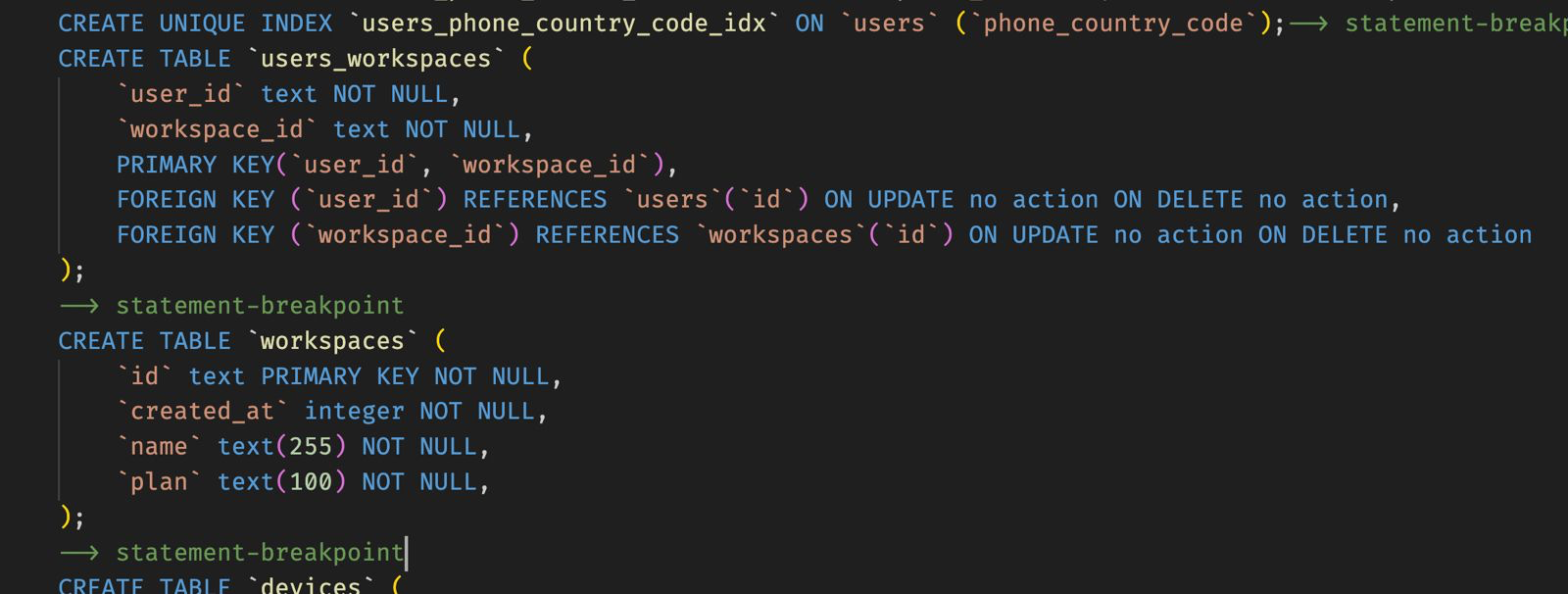
migrate()
function creating the session table: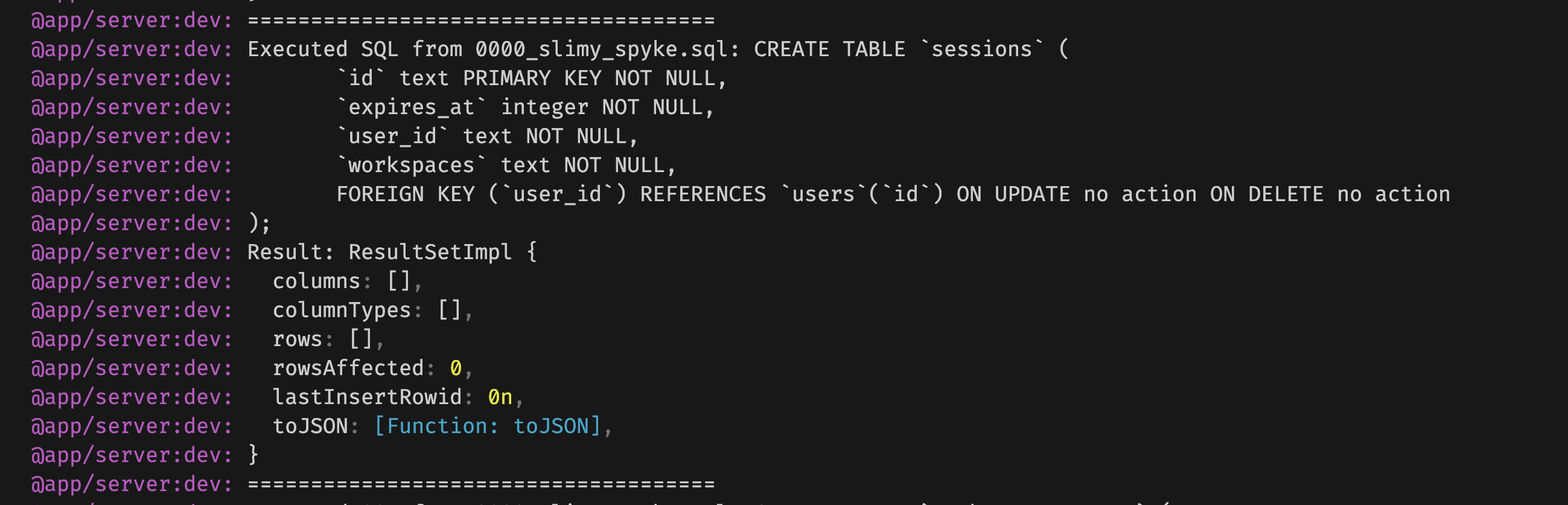
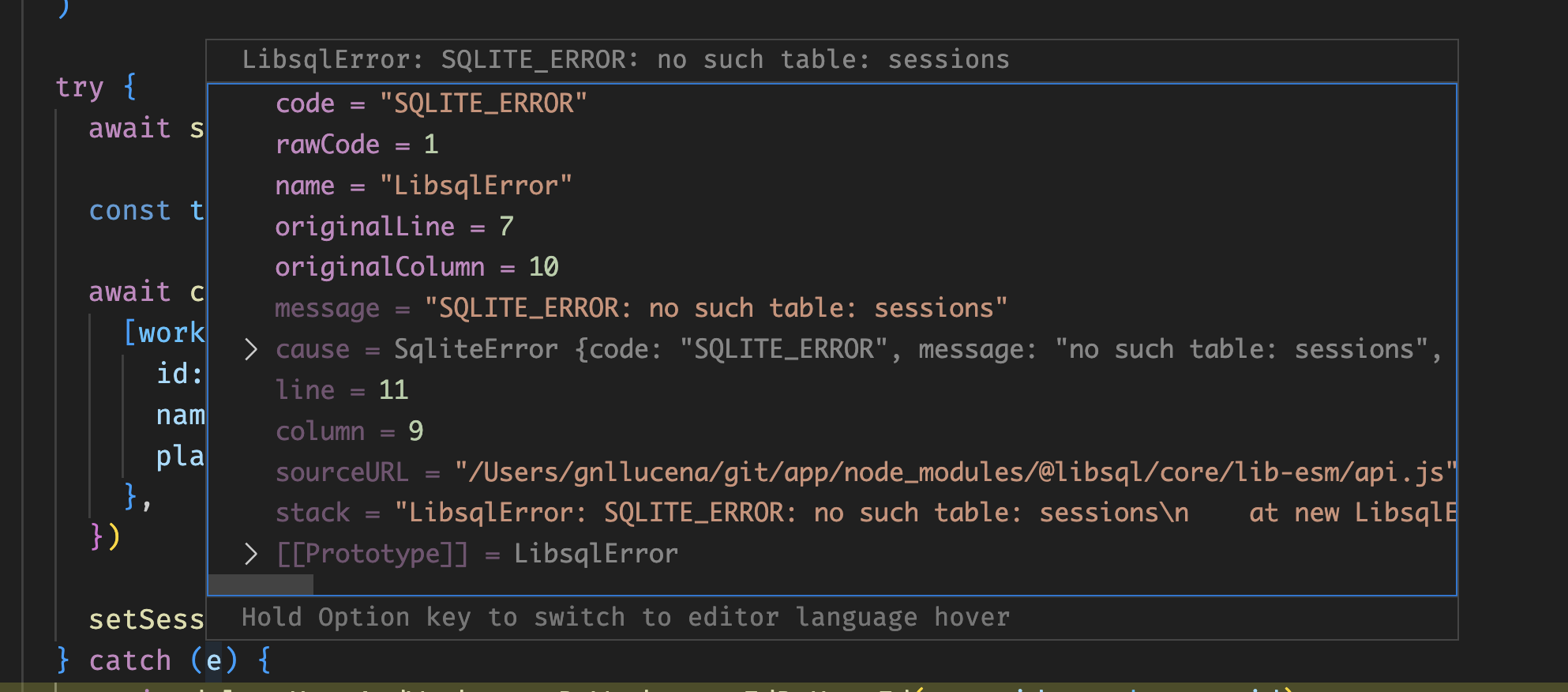
not sure what to do anymore to fix this, any ideas @opaldraggy?
you can turn off foreign key constraints while you're doing the migration, and turn them back on after. I believe that's what some of the migration batching scripts do in drizzle.
If you cant find an implementation that's exposed publicly by drizzle itself, try:
before your migrations, and then:
after.