Why is my EFCore code saturating my Postgres database connection pool?
I've got an API endpoint which applies a patch document to a resource on my database to update two values on a table, rather than re-sending all the table data. I've got a few endpoints feeding into postgres but this one seems to be saturating my connection pool for some reason.
Here's the code:
The endpoint is called every few minutes to update the system online status and current user count on a couple of virtual machines I'm running. As you can see in the attached image, I'm getting loads of
Client: ClientRead
wait events that don't disappear (there's 50 more if i scroll down). They're idle but, they don't disappear. This is the only endpoint that does this out of the 10+ enabled for the database.
Is there something I need to do in regards to closing a command sequence after applying a patch doc to a resource or something? I assumed _dbContext.SaveChangesAsync()
would just cut that off like any other database altering function.
Thanks!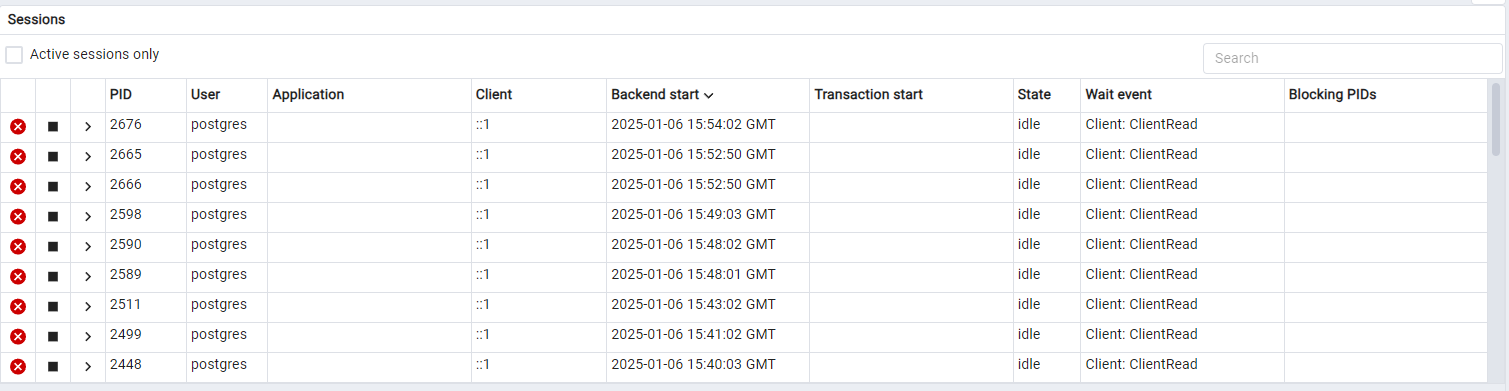
2 Replies
I figured it out. I'm calling the database asynchronously in a method that isn't even asynchronous. đź’€