Pending State not getting resolved
I'm trying to use the traditional signup method. But, it is in a pending state forever and not getting resolved.
The login method is working. At least, it is immediately returning something.
I'm using Next.js for the frontend and Express.js with the backend and drizzle pg for an ORM.
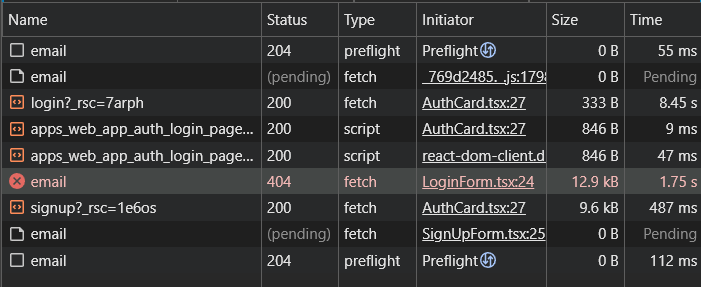
4 Replies
Update: It is the same for login method too
I'm getting requests to the backend server. But, something's happening. These requests aren't getting resolved
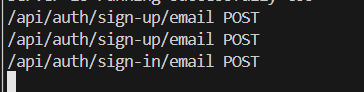
Can you show us how you setup your express server?
import dotenv from "dotenv";
import express, { Request, Response, NextFunction } from "express";
import cors from "cors";
import { router as contactRouter } from "./routes/contactRoute";
import { toNodeHandler } from "better-auth/node";
import { createDb } from "./db/config";
import { auth } from "./lib/auth";
import { sql } from "drizzle-orm";
dotenv.config();
const app = express();
// Middleware
app.use(
cors({
origin: "http://localhost:3000",
methods: ["GET", "POST", "PUT", "DELETE"],
credentials: true,
})
);
app.use(express.json());
app.use((req: Request, res: Response, next: NextFunction) => {
console.log(req.path, req.method);
next();
});
export let db: Awaited<ReturnType<typeof createDb>>;
async function startServer() {
try {
console.log("Attempting to connect to the DB...");
db = await createDb();
await db.execute(sql
SELECT 1
);
console.log("Connected to the DB successfully 🥳🥳");
// Set up auth routes after DB is initialized
app.all("/api/auth/*", toNodeHandler(auth));
app.use("/api/contact", contactRouter);
app.listen(process.env.PORT, () => {
console.log("Server is running successfully too");
});
} catch (error) {
console.error("Failed to start server:", error);
process.exit(1);
}
}
startServer();
This is the main file of the backend
Even the preflight request returns a 204 - No Content