API performance acting weird
I dont get why sometimes when im calling my endpoint the api is taking 20 ms but then bam the occasional 500ms or even 7600ms up to ive seen even 9000ms response for the same endpoint, just by calling the same endpoint with a few second delays. Is it something im doing wrong code-wise? For example this endpoint:
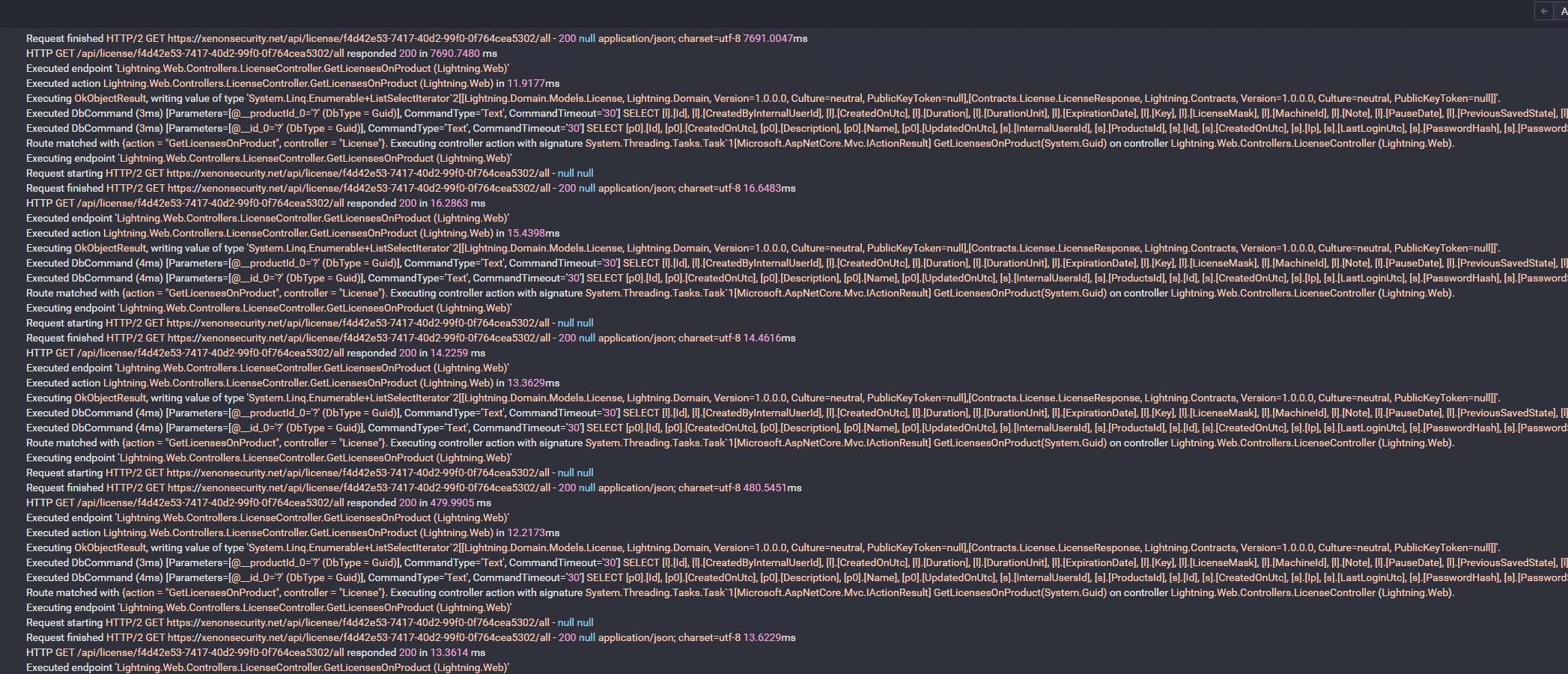
61 Replies
Wait, you're fetching a whole-ass product, just to check if it exists, then you fetch a bunch of licenses with that product ID...?
yeah is that stupid xD?
Why not just skip the check step? If the product doesn't exist, no licenses will be found, no?
Besides,
.AnyAsync()
will check just for the existence
Instead of loading the whole-ass objectwell the way I wrote my api is that I could have multiple "products" that have their own set of users and licenses
so I should only get the licenses for that product
But if a product does not exist (you get a null) how can a license that references it exist?
It cannot
oh yeah thats true lol
So you'll get an empty list
No need for that check
fair I see what you mean now
but could that really be the cause for it performing so bad?
Also, you seem to be fetching everything about the entity from the database, and only then do you pick the props you need
Probably not the cause
soo basically do the select inside the GetAllAsync function
But the faster you recognize how idiotic of an idea the repository pattern on top of EF is, the better :KEKW:
Whoever is pushing it may step on a LEGO with every step
May his socks be always wet
hahah
ive started to wonder about that actually
At most you want a service
do you have any suggestion what to follow instead?
Repositories are useless
just do the ef stuff inside my service?
EF is a repository
Ye
alright
but its weird according to the seq logs there are no other requests happening at the same time
just me spamming the one endpoint a bit
first I was thinking if there was any other calls blocking it or something..
but makes no sense to me why it would shoot up like 9000ms
You'd have to check with the profiler
I even expanded the server to 16 cores and 64 gb ram
i see
Nothing here — even with the repos and all — suggests the request should take any more than, like, 200ms
could it be a server issue or something idk
Unless the amount of data you're loading is massive
Im not even sure how to profile when its on the server
And you only trim it to something sensible after fetching
i just use a windows server
So the issue doesn't happen locally?
no it doesnt actually
there its like constant 20ms
Any reason to use Windows Server and pay out of the wazoo instead of just getting a random $5 Linux VPS?
but then again ive not rly tested with more than 20 licenses locally..
but on the server its not even a lot its like 100-200 licenses and performing like this
uhhh
just cause I have knowledge about how to use IIS cause we use IIS at work
and no knowledge how to set up on linux
Try filling the local db a bit and see if you can repro the issue locally
okay ill test it
Setting it up on Linux shouldn't be hard if you just dockerize it
yea i pay like €170 for the server per 4 months lol
Or even just rawdog it and run the
.dll
on bare Linux, even without any reverse proxyyou got any recommendations for providers?
$vps
Cheap recommendations
https://www.scaleway.com https://www.digitalocean.com
https://www.linode.com https://www.ovh.co.uk
https://www.time4vps.eu https://www.vultr.com
https://www.hetzner.com https://contabo.com
Always free options
https://cloud.google.com/free
https://www.oracle.com/cloud/free/#always-free
ah ty
well i use one that is close to hetzner
called avoro
I like OVH and Hetzner, personally
ill do some research on it appreciate it
sooo lets say i decide to remake this shit from scratch
do I use EF still or is dapper even faster
and no repository pattern that time
They're about the same nowadays
ah ok
i just wish there was like an easy "best practice" follow this and it will be good lol
Oh boy, best practices is another can of worms 😄
on youtube the asp.net guys ive seen on there just talk about repositories thats why i went for it
They all do lmao
My theory is they learn from similar YT videos and just keep propagating the repo agenda
LOL
If you were to ask about best practices, particularly on this server, you'll no doubt be pointed to $vsa
ima go through all of that
thanks man
that is super useful
But, again, not a gospel
wait I have one more question
Shoot
So in my api I have a feature thats kinda like a program builder...where it compiles something specific to the user and sends it back
this takes like 30 seconds per build
should I host this on a 2nd server
rn im doing it on the same
like my api is just a tool for authentication and mangaging your software
so I was thinking how to deal with the builds taking so long time, I dont want it hogging resources
Right... I guess you could do that, yeah, to have a guaranteed amount of resources for the API itself
yeaa
Could delegate it to some sort of a CI/CD server maybe, too
So you pay for the actual usage, instead of having to shell out however much for a server that might just be sitting idle 90% of the time
oh yea
true, those requests dont happen often
yeah im definitely gonna be rewriting things
watching one of those videos on that VSA structure, i love it
its way way cleaner than what im doing rn
In case it wasn’t already brought up, only measure performance in release when it comes to ASP. NET Core