TypeError: Cannot read properties of undefined (reading 'encoded') on skip function
34 Replies
track.requestedBy.id also undefined
Oh, I had the same problem often and I don’t know how to fix it, so I just delete the player
GG @hasselhoff, you just advanced to level 4!
I don't have this problem now, maybe you need to update moonlink
And this error also appears if someone tries to load unsupported links into your bot.
For example, yandex music or vk music which is not supported in your lavalink or just a link to an mp3 file
Any prevention?
GG @Nonsen, you just advanced to level 1!
Just set up a link check
Or shell I host my own lavalink?
To make the bot accept only links supported by your lavalink
It will be better
I using free lavalink so they might unsupport?
yes
Just do
Oh and how about access the track.requestedBy.id also undefined
And u see all info about your lavalink
This is a useless feature and I advise you to remove it because no one uses it.
player.position?
And it seems like the GuildMember object is simply stored there, and try logging track.requestedBy
Do you want to add a function that will show the number of the next tracks?
yes
By default this function is not there and you have to implement it yourself somehow
The docs kindda unreliable
I used to assign each track its own number when uploading.
I mean to get the current time of the track
But it worked very crookedly and that's why I removed it and just made numbers from 1-5 in the player itself
а
this one?
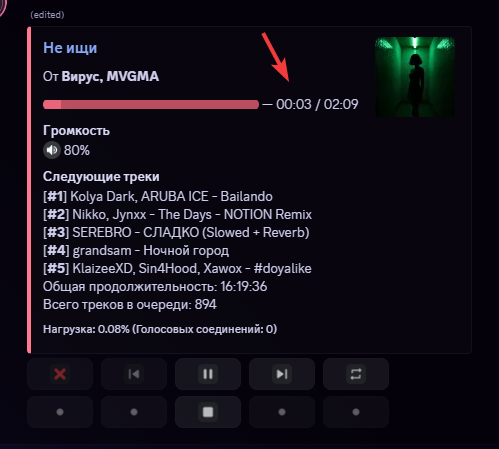
exactly
this is my func to get track time:
So you create your own function to track current time?
The function that they gave us not avaliable?
like this
This function converts the timestamp given by moonlink to normal time.
Yes I know how to convert time but the player.position always give 0
I have never used it
I always assigned the numbers myself
ok than thank you!
Last question which lavalink node you suggest
How is that?
I have my own
I think about to host my own
I am not sure which one to pick
Better host your own
This one? https://lavalink.dev
Lavalink Docs
Standalone audio sending node based on Lavaplayer.
yes