getting the returning data after inserting record as string | undefined instead of string
Hey folks here is my drizzle schema
I don't why after inserting record in the database the returning data has undefined also instead of specific types like string on columns
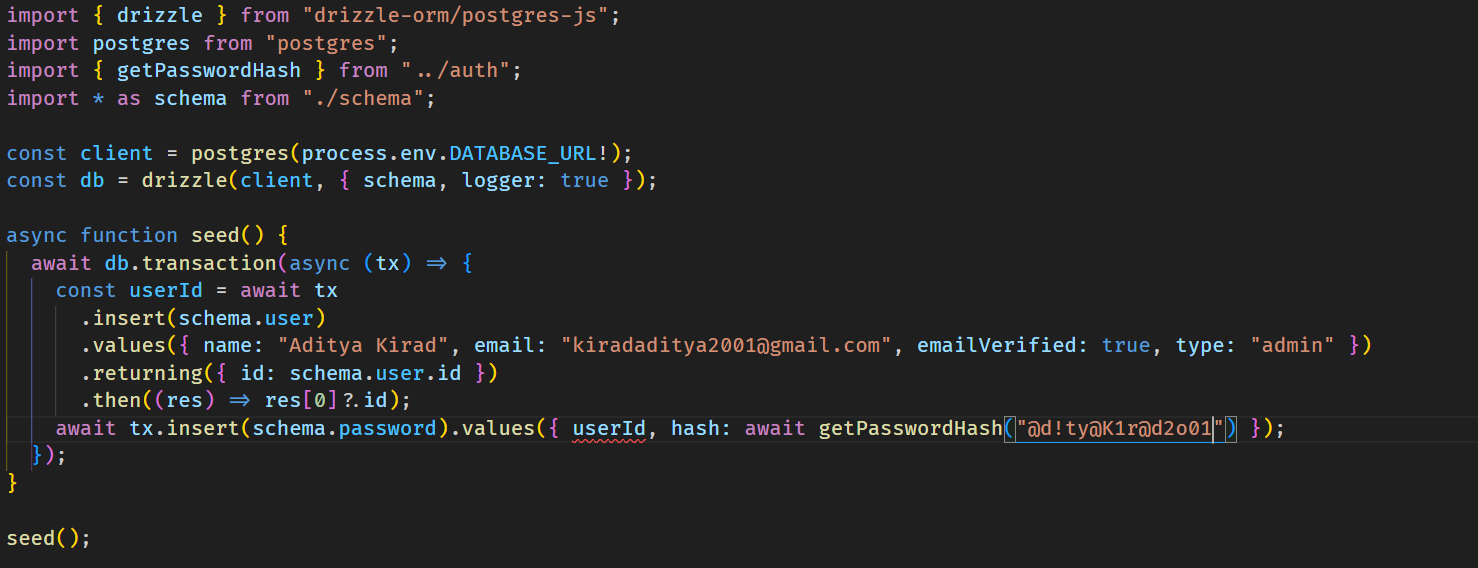
13 Replies
ofc it's undefined you clearly did this
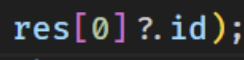
maybe do something like this:
const [{ id: userId }]
I had to I intentionally not did that in my other app I had the same code but there I didn't had this problem there here is schema I had in my that app https://mystb.in/6247b647461a3fd134 the only difference is there I was using sqlite and here postgres
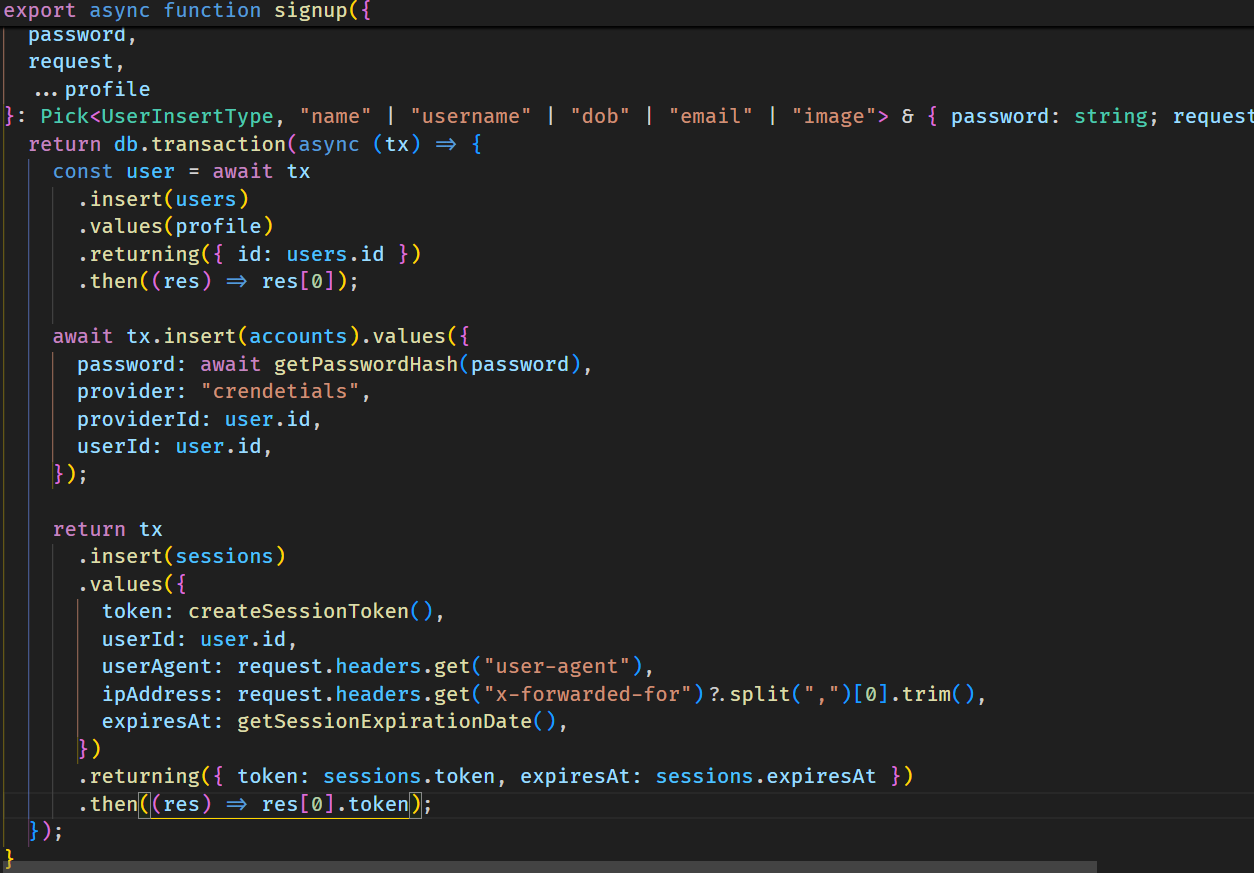
have you tried solution I gave you?
tried that already
it gives this error
Property 'id' does not exist on type '{ id: string; } | undefined'.
maybe use returnIds ?
postgres don't support that
TypeScript can't assume an array has a first value.
The same is true regardless of how you try to access it.
This is different from a tuple type.
If the second insert query relies on the first, then you should wrap it in an if statement.
@Aditya Kirad ping for notification
Firstly, in all of you example you are wrong
typeof
of value of in all examples will be number
instead of number | undefined
I just create new quakka sheet and run these examples you can see result in the images attached and secondly did you not see any of my previous screenshot in both screenshot there are similar type of queries the only difference is in first screenshot I'm using postgres as database and while in second sqlite is being used
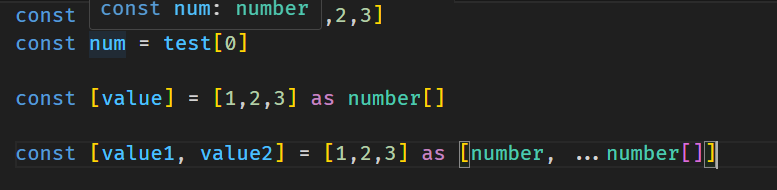
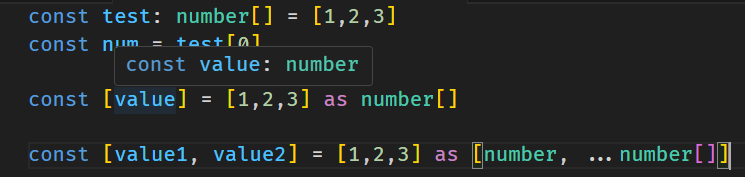
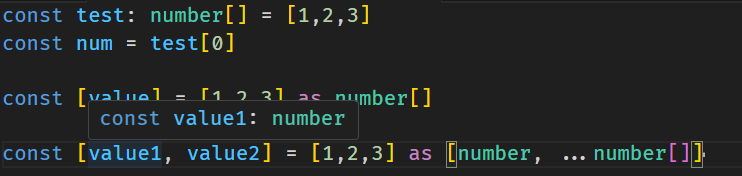
while retrieving the data using select query and then getting the undefined also makes sense but getting the data back after inserting it in database and then getting undefined on type doesn't makes sense
I see. In your postgres app, do you have
"noUncheckedIndexedAccess": true,
in your tsconfig? That causes the behavior I described.
I prefer having that property on in all my projects, because it catches potential runtime errors that TS wouldn't otherwise catch.
Example with it off:
https://www.typescriptlang.org/play/#code/MYewdgzgLgBGCuBbARgUwE4QFxyW9A2gLowC8MxA3AFDWiSxhm4oYQEAMRNA9DzAJgA9APxA
Example with it on:
https://www.typescriptlang.org/play/?noUncheckedIndexedAccess=true#code/MYewdgzgLgBGCuBbARgUwE4QFxyW9A2gLowC8MxA3AFDWiSxhm4oYQEAMRNA9DzAJgA9APxAthank you so much yes turning it of removed that error but as you said I will keep it turned on
@Sillvva just one more help can you tell even after using the guard clause why the userId type is not narrowing down to
string
it's still string | undefined
On this line, you should
throw
. tx.rollback()
throws internally, but I guess TS doesn't realize that. Adding throw
adds the type narrowing you're looking for. Otherwise, TS assumes the code is allowed to continue. And since userId hasn't been defined in the undefined case, it can still be undefined.
I believe it also rolls back if an error is thrown. That's all the rollback function does internally.