Errors while attempting to use Apify Proxy
I have created a very simple actor that uses the
gotScraping
library to retrieve the HTML from a set of YouTube video pages. The page URLs are passed as an input array.
When the actor runs, it correctly retrieves the first page in the sequence, but crashes when attempting to retrieve a different proxy for the second page (see attached screenshot)
What puzzles me is that the error does not get caught by the catch
block in my own code; it seems to crash at a lower level.
I am also not clear why I am seeing the warning message WARN ProxyConfiguration: Apify Proxy access check timed out.
and how to correct this issue. None of the docs seem to mention it.
Lastly, can you please clarify: if I want a different proxy for every request, should my call to Actor.createProxyConfiguration
be inside the loop, or prior to the loop?
Hoping somebody can advise. Many thanks,
Tim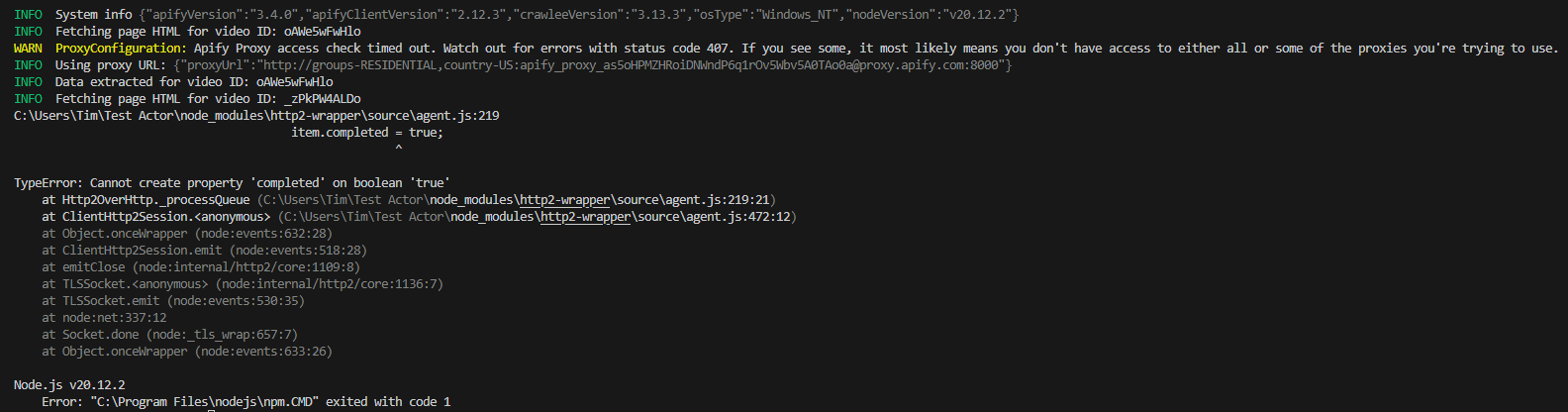
2 Replies
Someone will reply to you shortly. In the meantime, this might help:
Hey 👋
You should move the proxy declaration outside of the for loop. It's by nature a rotating proxy I believe (you can ping any IP service like https://www.ipify.org/ and see its result to confirm). Also, I'm wondering why do you want to use raw gotScraping instead of the available crawlers (which should lift most of the work?
ipify - A Simple Public IP Address API
ipify API is a simple public IP address API, easy enough to integrate into any application in seconds.