Can I .prepare() a transaction?
Surprisingly I can call prepare inside transaction callback but it doesn't make sense to me since we usually using prepare() as a variable out of block scope.
```ts
await db.transaction(async (tx) => {
const q1 = tx.update(...).set(...).where(...).prepare();...
Multiple records with same columns and values - using joins
I am using
innerJoins
and this is the result I get. Sorry for the bad formatting.
But except the fieldd
(which is different in all results) I get everything else the same
```ts
[...Test CloufFlare D1 migration
https://orm.drizzle.team/docs/migrations
```
import { drizzle } from 'drizzle-orm/mysql2';
import mysql from 'mysql2/promise';
import * as schema from './schema';...
Is it advised to always specify columns
When making queries (
db.query
or db.select
), it is generally best practice to specify the return columns you want? or will that not impact performance in most cases (unless your expected return query is large)Is there a good way to do "exists"
Im using pg. Is there a clean way to do a query analagous to something like
await db.user.exists({where: {x: y} });
...Smarter way to get schema type
typeof googleDriveConnection._.inferInsert
typeof googleDriveConnection._.inferSelect
is there a smarter/cleaner way to get the type of a schema?...$with using sql operator doesn't return type?
```
const companiesWithPrimaryAddress = db
.$with("companiesWithPrimaryAddress")
.as(
db...
How to select part of a string in Drizzle?
I have dates in ISO format (Stored as Strings) in my SQLite database. I need to be able to find a match only querying the year-month-day. Attached are two different ways I have tried this and neither seem to work.
...
const timers = await db.select().from(timerSchema).where(sql`to_tsvector ('simple', '${timerSchema date}') @@ to_tsquery('simple', '${queryDayString}'))`;
const timers = await db.select().from(timerSchema).where(sql`to_tsvector ('simple', '${timerSchema date}') @@ to_tsquery('simple', '${queryDayString}'))`;
Default UUID as text type
What is the recommended way to set the id (primary key) field as a text type with a default value of uuid
Does drizzle support a way to make an array transaction?
In prisma, you can do something like this
Is there a way to do something analogous in drizzle? I know you can do something like...
await db.$transaction([query1, query2])
await db.$transaction([query1, query2])
cannot push
```
error: relation "users" does not exist
at /home/ahmetkca/Projects/Oymak/overi/node_modules/.pnpm/[email protected]/node_modules/drizzle-kit/bin.cjs:24545:21
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async PgPostgres.query (/home/ahmetkca/Projects/Oymak/overi/node_modules/.pnpm/[email protected]/node_modules/drizzle-kit/bin.cjs:25506:21)...
Computed/derived fields in Drizzle Orm
Hi,
I am new to Drizzle, so sorry if it is a stupid question, but while picking it up, browsing the docs, etc... I noticed something missing I had normally with many other ORMs in the past: computed fields (as Prisma call them, for example as what I mean).
I don't know if I missed something, I didn't find an explanation in the introduction/philosophy of the docs... but I would like to know what the recommended path here and the stance of Drizzle on the subject (is it not implemented yet, not a desired feature because of complexity, performance, etc)....
Describe where statement for null children
Hello everyone. I have this code:
```typescript
export type CategoryId = number & { __typeName: 'Category' };
...
executing query builder sql
Dumb question but what's the best means of executing the result of my query builder? https://orm.drizzle.team/docs/goodies#standalone-query-builder
```ts
// what's the best way to execute this sql / parms?
const { sql, params } = query.toSQL();...
Migrations with new notNull() columns
what's the proper way to add a notNull() column through a migration? I just tried to add a column in my schema like
and generated the migration with the standard
foo: text('foo').notNull()
foo: text('foo').notNull()
npx drizzle-kit generate:pg
command..."No Such Column" Error
I'm using TypeScript, DrizzleORM, and SQLite (better-sqlite-3)
```ts
export function searchTodosAndShares(listIDs: string[]) {
if (listIDs.length === 0) return { shareMeta: [], todoMeta: [] }...
Type Helper to select fields
Hello there ! A picture is worth a thousands words. I'm trying to write this helper, but it breaks inference. Does anyone know of something that could work ?
```ts
export type Select<T> = {
[P in keyof T]?: boolean;...
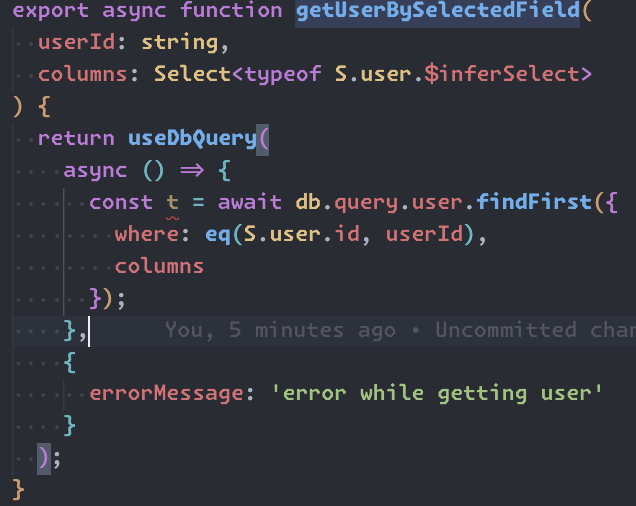
how to use types on frontend
Hey guyz, according to: https://discord.com/channels/1043890932593987624/1179496917420490782 it is possible to get types for inserts or reads; thats super dope, but I have a question, I am not sure how to use those types on fronted.
Got two folders, one for backend with expressjs + drizzle and second one for frontend (svelte + sveltekit). How can I import those types?
backend...
Drizzle MySQL Many to Many relations
I am trying to define a many to many relation ship between the employees and strengths tables.
I am getting the error on the employeeStrengthRelations definition. Type 'any[]' is not assignable to type 'Record<string, Relation<any>>'....
I am getting the error on the employeeStrengthRelations definition. Type 'any[]' is not assignable to type 'Record<string, Relation<any>>'....
Drizzle with MySQL - most optimal way to insert row and immediately retrieve inserted row
Hey, thanks for helping me out :)
I'm currently using Drizzle in a serverless application with PlanetScale. Here is some code where I insert a row and immediately after retrieve it:
```typescript...