✅ How does CultureInfo works in C#?
en-us
and we try to compare something with de-DE
, their might be issues here? I know the "ß (Eszett)" stands for "ss" I think... so, what kind of issues might arise?
If we write something like this for eg:
```C#...Why deletion of last character in Entry not triggers observableProperty? (MVVM, Maui)
Appending to StringBuilder by Unicode code point?
✅ When to use each constructor?
Any good guides/videos to learn Abstract Classes?
Number doesn't get printed
✅ DateOnly.TryParse Vs DateOnly.TryParseExact use cases
DateOnly
data type. I didn't understand the difference between DateOnly.TryParse
and DateOnly.TryParseExact
. Normally, when we use the parse method, the string given is parsed according to our system culture? How does TryParse and TryParseExact differs?✅ How to handle websockets?
Button wont get a listener
Any good guides for learning Blazor WASM?
Project Subfolder Remains Hidden in Rider
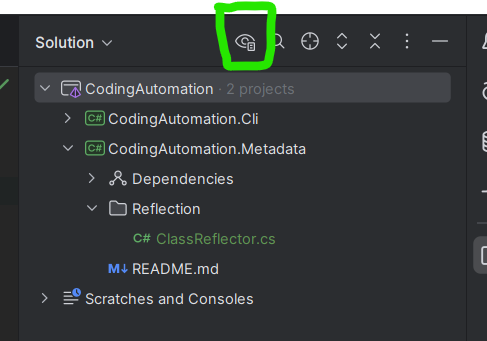
✅ Rider does not recognise WebImage Helper
WebImage photo = null;...
✅ Mouse position in console
✅ how to load sos from JetBrains CLion bundled lldb@19
How to Automate Code Compare and Merge
ASP Identity <> Microsoft Entra ID
AutoMapper example projects with mapping profiles
Repository Pattern and Clean Architecture
Visual Studio includes only x86 dlls in build
Need help Publishing / Trimming my Maui / Blazor app