How do I check if user has permission to execute command
What the title says. I found
ApplicationCommandPermissionsManager#has
and tried it with but all that method does is to check if that specific user has an override in the permissions.
I want to check if the user's combined permissions (from all roles and individual overrides) are sufficient to invoke the command. Do I really have to iterate through every single channel in the guild and every single role on the user and call has()
all those times to figure this out? Surely not, because that wouldn't even cover admin permissions AFAIK.33 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.Im kinda confused - why do you need to check?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I need to check because I have a button that invokes a command
Yeah exactly
Ahhh okay
The best thing would be if I could get a Bitfield with the command permissions
Just like default permissions
But I can't find anything like that
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
There is
<ButtonInteraction>.memberPermissions
I need the permissions for the command though
Which is the calculated permissions for the member in the channel in which the interactionwas executed
Yeah command permissions cant be simplified into a bitfield
Figured as much
You're saying this isn't possible then?
I don't necessarily need a bit field
I still kinda think you've overcomplicated it?
So you have a command A that sends buttons, and when a button is clicked it will execute command B
And you want to know if the user has permission to execute Command B
In that channel?
Correct 👍 Small detail is that there is no command A, it's just buttons on a message
But the buttons do invoke command B (and C, and so on)
One button per command
Alright well for starters, per channel isnt necessary
You probably would need to do it per role though to check
But uhhh
et voilà
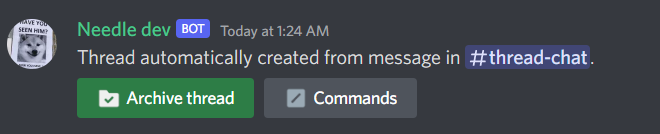
member.roles.cache.some(r => guild.commands.permissions.has(...))
should let you know if any role has an overwritefor a role yeah, I guess I would also do it for the user that clicks the button
Yeah, a single user check would be another line
I kinda see where this is going
And if both of those are false, you can assume its default permissions
And first condition would be if the user has admin perms
yeah thats an easy check
yep
Yeah I'd pass this off to a re-usable utility function for sure
you said channel overrides are not needed, I couldn't really understand why?
Iterating over every channel isnt
right just check the channel we're pressing the button in
Do you mean that the admin might have disabled the /archivethread command in this spot, so you dont want the button to work?
yup
And you already have
<ButtonInteraction>.memberPermissions
which is the calculated set for that channelbut since it's in a thread I would have to get the parent's channel ID
which can be NULL 😉 shoutout to this issue https://github.com/discordjs/discord.js/issues/8471
but yeah that's whatever I guess
Is it documented anywhere in what order the permission overrides are calculated? Like does channel take precedence over user and does allow go over deny, etc
because I would have to follow those rules too
What to check first and whether an explicit disallow for a user makes an explicit allow on a role useless or not
Dunno tbh
I imagine Channel > User > Role
I guess I have some experimenting to do then, I'll post here when I've figured it out for future reference
Thanks for everything so far, just needed a nudge in the right direction
Alright
I think I've done it
It became longer than I thought
I don't know how well it will work for private threads or other edge cases but here it is
test
why is clyde complaining when I send the rest of the code that I'm not friends with the recipient lmao

enjoy
this.id
is the command ID and this.defaultPermissions
is the bigint default perms
This was correct