use mysql values for export const
Hey quick question.
I got my values of the embed (title, color, desc, footer, etc) in my mysql database. I want them in my
embed.js
for all the new EmbedBuilder()
. After that I want to export my Builder to my interactionCreate.js
or messageCreate.js
.
How is that possible? Can you may send me some advice or documentation? :D45 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.Make a function that gets the data from the db, puts it into the builder and returns it
Like this:
?
Return doesn’t work here bc ur in a nested callback
oh yea I see
You would either have to make a new Promise constructor or just use the promise wrapper of MySQL
okay that's what I wanted to hear
Let me try this, thanks
Hey, I searched a lot and, if I use an promise I can't
export const test_embed = new EmbedBuilder()
, can I?No
Well, you prob could w/ es6 import/export
But how should I deal with this then? I need to export it :D
tried
doesn't work either
You can’t use export in callbacks tho, it has to be top-level
That sounds to heavy for me tho
That’s why I just suggested to make a function that makes the embed
Wait let me explain
You can memoize it if you want to
I have an file called
embed.js
there should all embed get builded for my other files like interactionCreate.js
, messageCreate.js
, etc.
I worked for this with ES6 no problem worked fine.
I worked without the ES6 module, also do the db query in my messageCreate.js
(with the values of db) worked fine aswell.
But to make my code much clearer I decided to move my db connection to my builder file. In this builder file I wanted to do what my problem is. :c
And I guess that's not working as you said
@kinect3000 One idea
You know I have in the file messageCreate.js
the
Can I export the function from the db into this? Or may loadScript the embed.js
-file?Can’t you do the same w/ putting it on the top-level
Or you said doesn’t work in non-es6
Bro, be careful I'm an beginner I won't get this top level things 💀
It’s easier to just use mysql2/promise
okay yes
Do you know what top-level means?
Sadly no, I heard of that while I was researching but ignored it.
Ah does that mean it has to be on the top of the file?
Not necessarily
oh 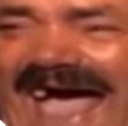
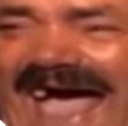
It has to due w/ scopes
Basically any code that isn’t inside of a function
Can this work:
messageCreate.js
in embed.js
I guess with some changes but do you get my idea? Or is this shit xd
Or could this work in an promise?
GitHub
node-mysql2/Promise-Wrapper.md at master · sidorares/node-mysql2
:zap: fast mysqljs/mysql compatible mysql driver for node.js - node-mysql2/Promise-Wrapper.md at master · sidorares/node-mysql2
It’s much easier to just use async/await
Otherwise, you have to make ur own Promise
Bruh that's an level to high for me
Async/await is pretty easy imo
I guess I know what I do now: learn more about java script
const data = await myPromise
Where data
is the result of myPromise
sure, it is, but it's not for my case
You have to use the promise wrapper for MySQL
oh, ok wait that explain something
whats what I never did read lmao
can I use this aswell:
export const data = await myPromise
?Yea
Only w/ esm tho
esm is activated
for sure
kinda dumb question, how do I define my promise ?
with mysql2?
Are you not using mysql2?
I do
You use the code I linked to use promises and async/await instead of callback-style promises
I did it wrong?
Didn't you say that I should use async/await ?
Not sure where you got testPromise from
Ur db.query is still using callback-style promise
oh I though it could be an string, my bad
Okay, my plan did change. I do not need to export anymore because, what if I need any interactionvalue like
${interaction.id}
. So I moved query into the messageCreate.js.
It works fine now. But maybe you can tell me how I get like the message.author.id
into my query?
Code:
I guess I've to work with promise aswell right?