Convert Array to String
Hello, I have some problems to convert Array to String. So in my example code i have an array of 4 items. I do know that i can just arr.join(" ") to convert it to a string. However, i don't want to include the first item from the array in my new string.
I have created a code that kinda works but is there any better way to do it?
This is my example code
10 Replies
Right now, you don't have an array, you only have a string...
You need to wrap it in square brackets to make an array, and then you'll want each item be have quotes around it too, since each one is a string, or else it'll just be an array with one item in it
let arr = ["Item1", "Item2", "Item3", "Item4"]
I am using arr.split(" ") to convert the string to an array
Then i want to convert it back to a string but not include "Item1 "
If you check the MDN you can see that it does convert the string to an array
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/split
String.prototype.split() - JavaScript | MDN
The split() method takes a pattern and divides a String into an ordered list of substrings by searching for the pattern, puts these substrings into an array, and returns the array.
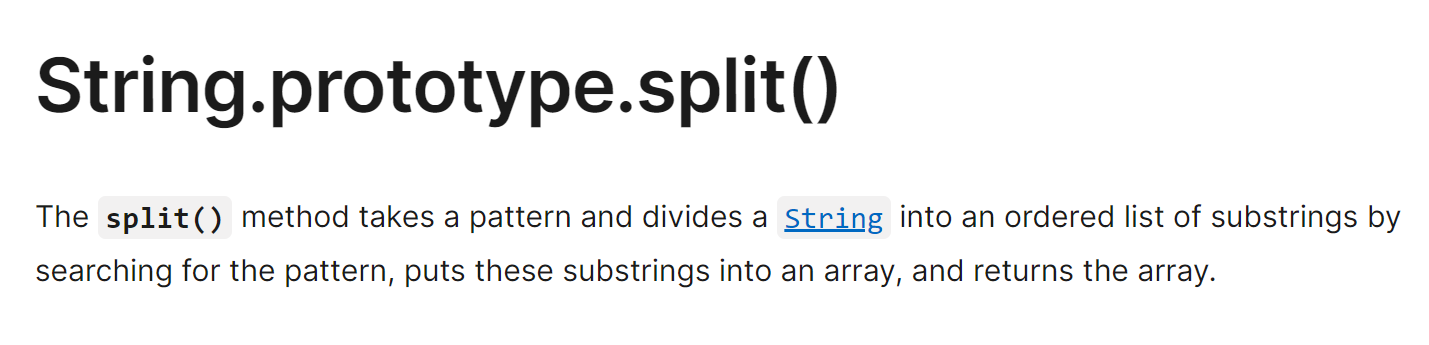
and also
Array.isArray(arr)
does return true which confirms that it is indeed an arrayoh, fair enough, lol. I just saw the first line and stopped looking, my bad 😅
Aaah heh :p it's fine 😂
arr.slice(1) will make an array starting from index 1, then it joins with a space
if you want to drop the first word from the text, without splitting it to an array, you can do
Oh thanks. This seems to work !
with your original loop approach you can simplify it to
Oh alright. Thank you very much, i will try this 🙂