Copy to clipboard button
I have this structure:
See sample: https://i.gyazo.com/792cda5b3f1b25e4cf385a4700f62764.png
The button retrieves the content inside
autoloot-text
, on which every one has a unique id, like seen in the example.
Currently I use this JS function:
The issue is that Astro doesn't seem to pick up the inline JS there is in HTML, for a reason I still don't understand, so it breaks.
Tried looking online for solutions, and found this one that is pretty helpful:
https://codepen.io/olawanlejoel/pen/MWGLXyX?editors=1010
The problem is that it targets a specific class, and in this case, every p
would be different to one another, and I cannot come across for a solution to it :(
My lack of knowledge and/or understanding of Astro is making this worse.
I tried putting the JS in the page, it works, but then when I build it, it stops working, then I defer it, it works partially, then I put it as an import
, it works pre-build, and breaks after, then inside a script, doesn't recognize the document
(since it's server side apparently - I still don't understand the difference).
So any help would be... extremely appreciated.15 Replies
that function has jQuery though?
here is one I use
Does that still require inline JS?
na you can just use an event listener
Mind showing me an example?
In this case I have 7 different ids.
All of them a
p
to be copied, with content in it.so ids need to be unique or should be
they are all named
All-Illusion-Autoloot
?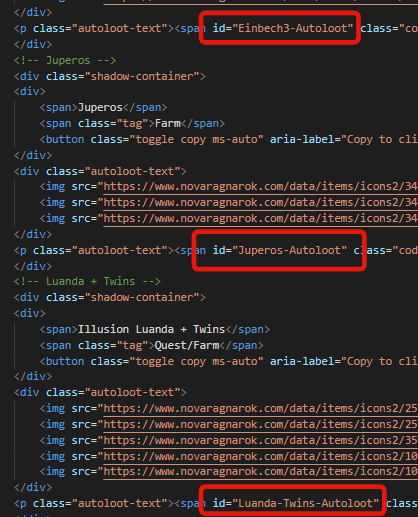
This is an example.
They all have an id with the name "something-Autoloot".
do they all share a parent?
Yeah, class "autoloot-list".
sorry trying to get the kids in bed
There is probably a better way than surfing the dom but I probably want some kinda Event Delegation to limit the code a bit
https://codepen.io/b1mind/pen/gOKreLL?editors=1011
Oh I thought I got your structure right but yea its a bit off, maybe cleaner if you use datasets 🤔
I'm a bit confused by the p > span honestly xD but yea I updated again with no dom surfing and used your class names for the most part. let me know if you have questions.
I have a lot of questions.
That's mostly because the
p
hides/shows on click. So it's essentially a "div", or a poor div.
In this case, why let
is the right choice over a const
? Simply because it's local?
From what I understand from the first function is that, it uses the Copy API, right?
Then you define a variable tempInput
on which you create a textarea.
You assignate a value to it? I don't understand why.
Then you append this to the body.
Select it.
Execute the copy command (which is striked for a reason in my JS).
Essentially, you create a "fake" area on where to "paste" this information, right?
Then the bottom one basically uses this "dad div" and tells it "hey, the closest event, that happens to be a button, I want you to store a variable here".
If the variable is "empty", just go back.
But if not, then search for the closest "dad" with the class of "shadow-container", and select from within an ID that has within its name "Autoloot", its text?
Then console it? (don't get why)
And "fetch"? the previously API command?
Or no... copy the text from the event listener, and paste it on the Copy Clipboard, which is for the final user?I use let cause I'm lazy and its less chars... don't be like me
I don't use the clipboard API cause it was not supported when I wrote that snippet, so its basically ya making a fake textarea to copy from, then removing it. Its just the way.
I console.dir() cause its nice to see the dom/methods tree when I'm screwing around
So the rest is basic understanding of how Functions in JS work
You are still not learning it fully yet?
func(param)
So value is what ever you pass into the function like line 18
so essentially I'm passing the innerText into to the copy() function that then saves it
value
into the input
I'm bad at explaining things sorry xD
Also I forget where you are in knowledge sometimes. Your websites coming along btw. Liking it 😄 keep up the hard work
Yea you get it though 😄
also my snippet is pretty much the exact same as the one you had above, accept mine takes a string vs an element. Well that and it was jQuery
so onclick="copyToClipboard('#id')"
you were sending it a string, it would still have had to query for that id
oo I lied $(element)
would have query'd the string/id
jQuery! I should sleep lolI'm still JS newbie
thank you for the help!
The logic behind making a JS seems clear as water sometimes, to me, but...
When it comes to know what to use, is when I crumble. I guess experience will give me that.
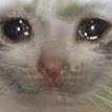
Yea I mean you seem to read it and make sense of it well for not knowing it
Thank you. I will keep giving out my best.