JavaScript most complicated code
i found this code on MDN in array.from and this is really really complicated for me , i mean what does the parameter _ and i stand for ? and how did they even come here they haven't get declared i wish i get a comprehensive answer
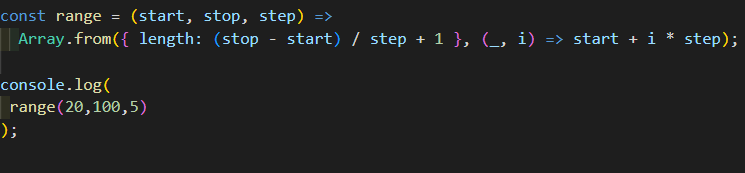
9 Replies
if you take a look at the MDN article on
Array.from
, you can see that it takes one to three arguments. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/from
The first argument is "An iterable or array-like object", which is further defined lower down on the page. "Array like objects" are "objects with a length property and indexed elements". They define the length as <unimportant math based on the function inputs>, and add no direct way to access internal indexes, so it's technically an incomplete parameter and not array-like at all, but close enough for this case because of the next argument
The second argument is a mapping function, that's called for every element in the array. That argument expects a function that takes two arguments, the current element, and the current index.
_
is a bit of a convention in programming in general and usually used as a variable that isn't going to be used at all. Sometimes you need to accept a value from something, but you don't actually need it, so you store it in _
so you and other programmers will know later that it's not used at all. i
is just shorthand for index
and used all the time.
The reason they're not declared, is that they're function parameters. everything after the object (so after },
) is an arrow function with an implicit return.
An implicit return is a special function of an arrow function, where if you omit the curly braces when you define the function and only have one expression in the body, it simply returns the value of that expression without you putting return
in front of it. It's handy for when you're writing inline functions to pass to other functions.
What this is doing, is relying on the implementation of Array.from
not really caring or checking that the array-like is actually an array, but assuming it is if it sees a length property. It iterates over it by doing a for loop, where it just starts at 0 and keeps going until length - 1.
So what it does in the end, is figure out how long the return array needs to be to have elements in it that start at 20 and end at 100, with 5 in between each element. That is fed to Array.from
which sees the length property and assumes it can just start calling the mapping function with some unimportant value (likely just undefined
) for the _
parameter, and the current index in the i
parameter. That then returns the proper value for that index, without ever referencing the "array-like" that was passed in, because it doesn't need it
you could also write the range function like this
it's effectively identical, just much more verbose
purely as commentary, I don't think it's a very good idea to write code that looks like that. It's harder to read and reason about, and it's much harder to fix code than it is to write it. I wouldn't go as verbose with it as the code I wrote, but nesting implicit returns feels messy, uncommented math can be hard to reason about especially when you rely on operator precedence, and it's just much harder to read than something like this
@aburaish ^@jochemm thank you very much i really needed these tips and information
I hope it's all clear 🙂 if not, feel free to ask more (though I'll probably answer some time tomorrow if someone else doesn't, I'm heading off for the night soon)
@jochemm really appreciate your help but i have another question , why _ value here is undefined
I'm not 100% sure it is undefined, but the reason I think it might be is because in the description for Array.from, it says that that's the current element on the array-like. The array-like in this thing doesn't have any elements though, so if you try to access ararylike[3] for example, it'll return undefined
@jochemm Understood , and thank you again
no worries 🙂
Here's a really good explanation of the underscore, which essentially is a variable we use when we don't want to define a specific variable name for something unused: https://stackoverflow.com/a/42857472/5994673
Stack Overflow
In javaScript, what does the underscore stand for when used within ...
This was derived from another coders Codewars solution
Aim: The code at this point in time is used to return the sum of rows and columns and diagonals, to see if all the sums stated of this 4x4 ...
I also thought the same I mostly only use array.from to store my nodelist in an array form to access some prototype methods