Type 'StoreState | undefined' must have a '[Symbol.iterator]()' method that returns an iterator.
I'm trying to setup a simple context provider plz help 😦
and use it in a component
but
const [socket, { set }] = useSocketIo()
gives me an error18 Replies
useContext
returns T | undefined
by default because the context might be missing. And you cannot destructure an undefined
useContext
accepts a second value that will be used as a fallback
or so thisoh alright, I was basing my code on this example https://codesandbox.io/s/counter-context-gur76?file=/counter-store.tsx:433-438
ryansolid
CodeSandbox
Counter Context - CodeSandbox
Counter Context by ryansolid using babel-preset-solid, solid-js
ooh noice
this example is better (better ts)
https://www.solidjs.com/examples/context
SolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
you're da MVP damn
wait I'm dumb I missed this section on the website 😂
thanks for your time !
hum okay everything work but if I initialize the socket to false I get a new error
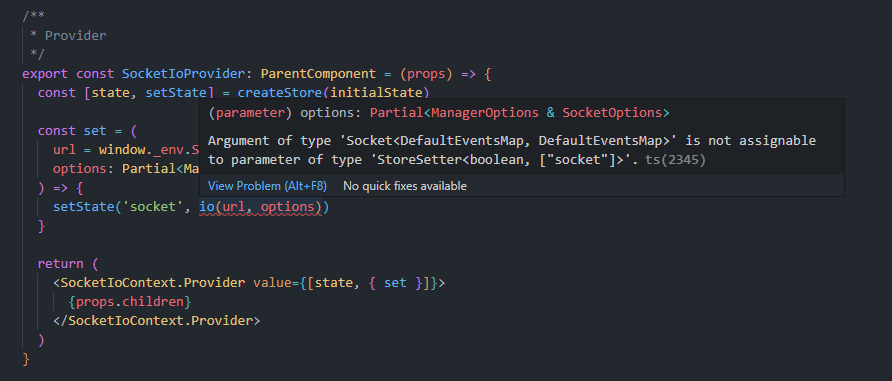
even worse if I set the type to
false | Socket
I get an error at createContext
full code
I think maybe I should start from scratch and actually learn how this framework works... I might be trying to do stuff like I used to with react... for ex my process with this before was:
a user login -> we get the authToken and store it -> redirect the user to the protected parts, then use this token to configure the socket globally on the app with zustand using a hook that expose methods to initialize the socket then connect to itcreateStore<{ socket: boolean | TypeOfTheSocketThing }>(initialState)
store infers it as boolean only
from the initial value
or use the StoreState
or new ts 4.9 satisfying syntax
man this is black magic
yeah I'm dumb...
Man I though that it was gonna be easier since the syntax is close to react 😂
I'm lost but thankfully you're here
not sure how context works in react, but I know that typescript works the same way...
reading and understanding ts errors will help you in every framework
Well I was relying entirely on zustand, and indeed using different frameworks will help to understand new stuff, and solid might actually be the one where I will learn the most it seems 😂
I guess zustand might be guiding you better
solid just gives you a couple of primitives and says have fun
Well for zustand it's pretty much basic object manipulation, so you define the type once and voila
also where I needed to do specific stuff with types was pretty centralized since I would use only one
createStore
so I had to do "weird" manipulation once and it would simplify my type declaration everywhere as I import my slices onto the store
and yeah there wasn't much to play around either, pretty much straight forwardwell that was easy
here's zustand for you I guess
https://playground.solidjs.com/anonymous/4143ce0a-5683-4db6-9227-96d8583f0421
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
lmao you actually did it 😂
But I asked in general and it wasn't really useful since Solid is pretty good for state management, the implementation is interesting nontheless
wait
what I though you actually used zustand but no
it is pointless, this was just to prove a point
man 😂
yeah solid is indeed amazing for state management, I'm really happy I stubbled upon it