context menus not working
code:
interactionCreate event:
Issue:
bot returns
The application did not respond
For some reason console.log(contextCommand)
doesn't return anything15 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
someone?
if
console.log(interaction.commandId)
didn't log anything, then it did not execute
this means that either this listener didn't execute due to it not being registered properly, or it was unable to find a command in your commands
collection with that keyIf you aren't getting any errors, try to place
console.log
checkpoints throughout your code to find out where execution stops.
• Once you do, log relevant values and if-conditions
• More sophisticated debugging methods are breakpoints and runtime inspections: learn moregot this
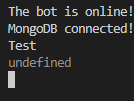
is
Test
the name of the command you used?yes
then it was unable to find a command with the key
Test
in your commands
collectionwhat could be the reason
not setting it properly, since that's your collection that you created
I logged
commands
, idk if this helps in anything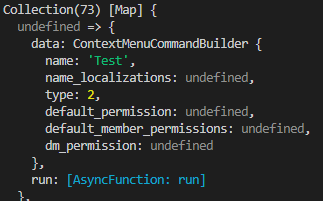
since the issue is that it wasn't set properly, the issue will be where you set the commands in the
commands
collection 🙂here?
yep
command
in this context will be the object exported by your command files, so that means it has data
and run
likely you wanted to access command.data.name
for the command's name, rather than command.name
fixed it, ty