Error concatenating strings in a for loop
Hello, I'm trying to generate a random string of length 12
What I'm trying to do is, first, generate a random character, using the ASCII table starting at 21 till 127. Then with the String.fromCharCode, I get the character equivalent of the number generated.
The idea is that, I have an empty string and I use the += operator to concatenate whatever char I get from the loop, easy peasy lemon squeezy, nothing out of the ordinary, BUT, and this may be a conceptual mistake, when I run this code I get a reference error saying that
id has already BEEN DECLARED
but I don't see myself redeclaring it so I don't understand.
Also, I've tried with the .concat method and it still gives the same result. Using const doesn't work either.6 Replies
Issue found, id was defined else where when I opened my console
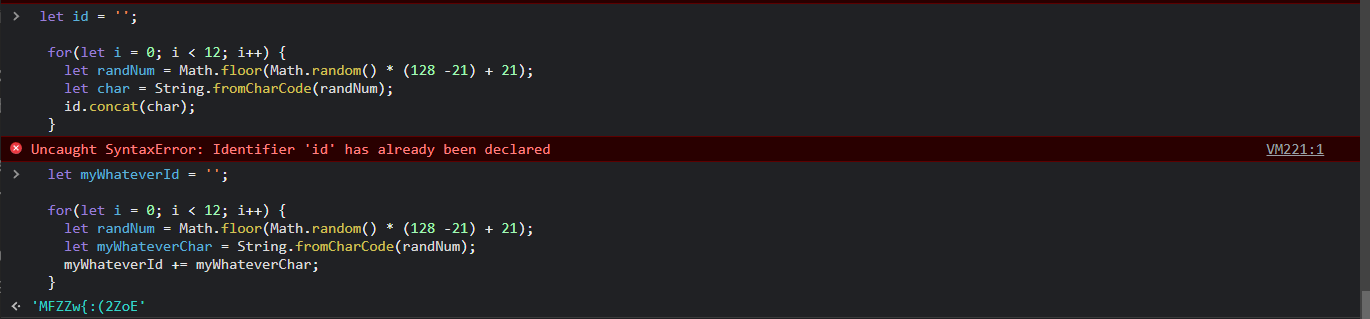
I was already confused because it worked for me
Guess using "id" or "char" are too common
make it a function so you wont run into that problem. Also you can pass different arguments. for example length
Something like this
Thanks!