Cannot read properties of undefined.
Hello 👋
So I'm trying to create a class for an alert card. And now whenever i try to use the class methods it throws an error saying
Cannot read properties of undefined ( reading 'classList')
What am I missing here?6 Replies
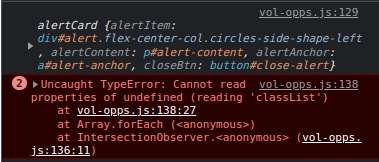
And this is the error.
I don't understand why when i console log the whole object it displays it with its every property. But when i use any of the class methods it says the element is undefined.
I also tried to change the object class from outside of the Class by accessing the properties. and it throws the same error.
So what am I missing here?
when you create a card instance, you'll have to store it in a variable to be able to get access to it's methods and properties outside the class
Also, a good practice is to name classes this a captital letter
also, if you want to access methods outside the class, they can not be static or private
there were some other issues as well
i made it a bit cleaner by passing the id selector into the constructor function, so you dont have to select it first
inside the constructor we select the element and the other parts you need
Thank you so much Mark!
It's working now 🙌
And thanks for the Tips!😊
no problem. good luck!