Multiple renders of the same chat sent via Pusher
So I'm making a T3-based chat room app. I'm running into a weird problem where the chats are randomly starting to get rerendered more and more times. I'm not sure what's triggering it, on initial page load of the client, everything's working just fine, then after a couple minutes it'll start to render each message 3x, then 4x, 5x, etc...
I'm using the timeSent(timeSent: new Date()) as the key for the map. Pretty sure it doesn't have anything to do with pusher itself, since the chats are only rerendering multiple times on a per-client basis(i.e. even if it's rerendering 6 times on my client, the other person in the chat room sees everything as normal, till the bug starts happening to them later down the road)
Pusher useEffect:
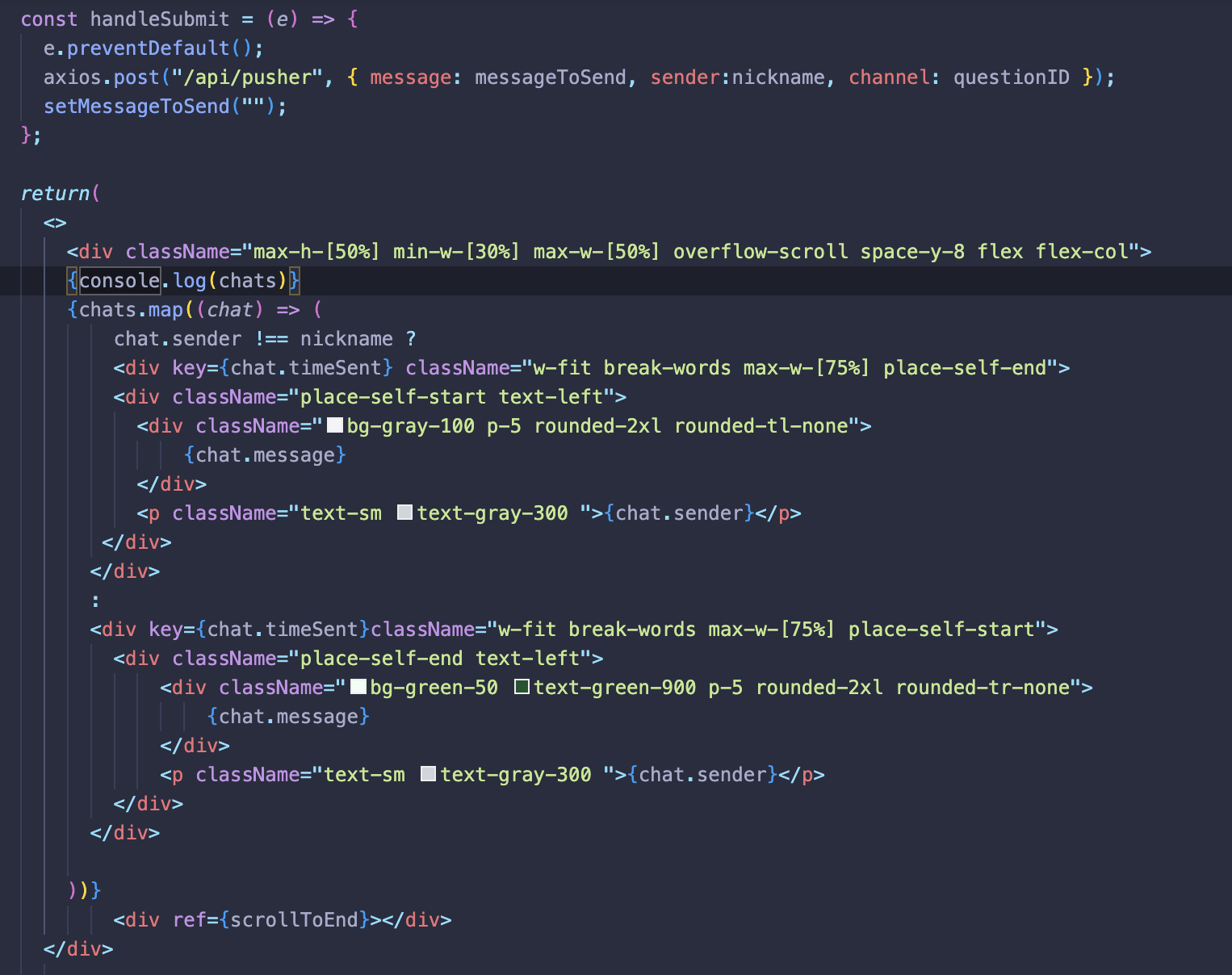
9 Replies
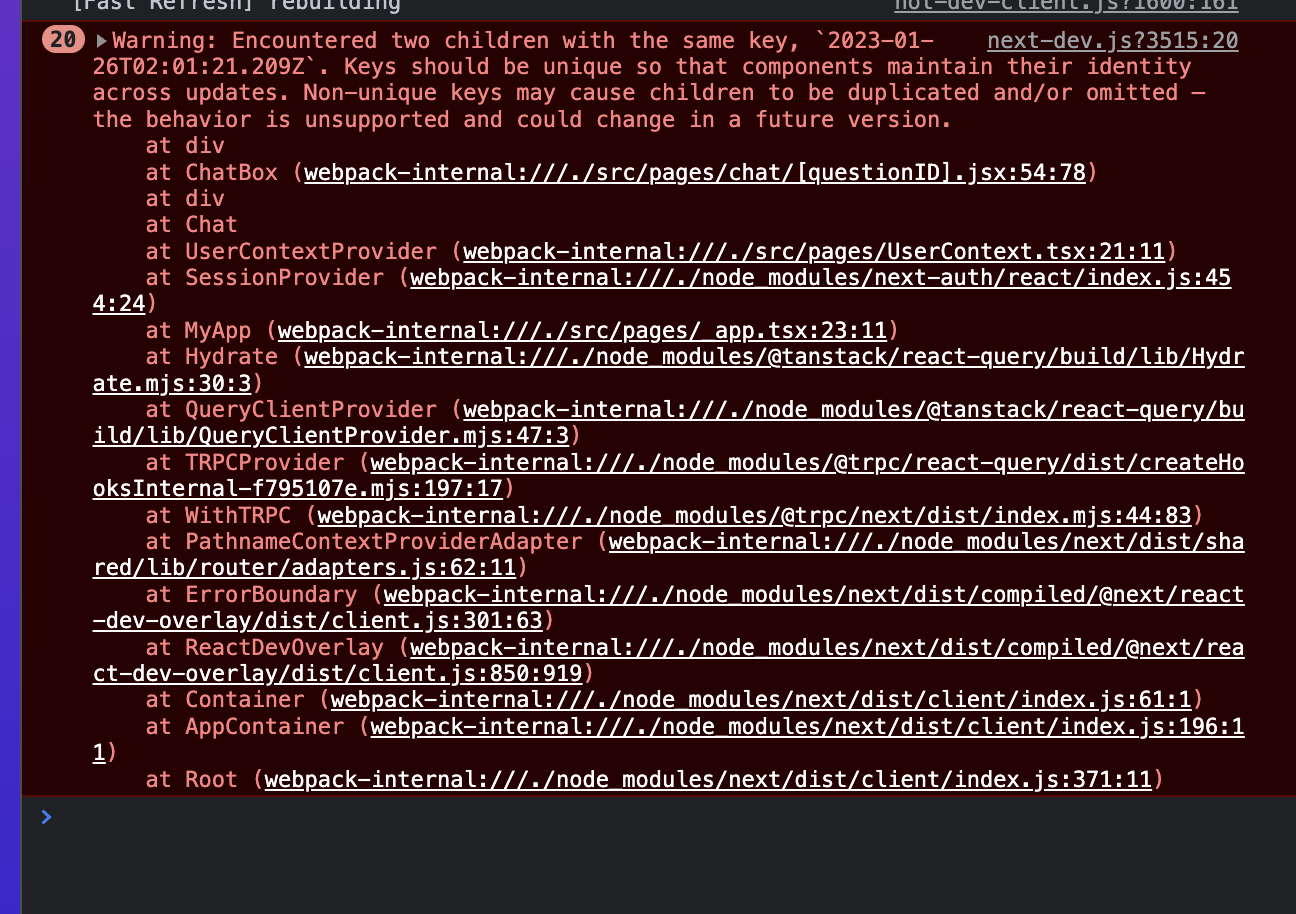
So this is client one
As expected
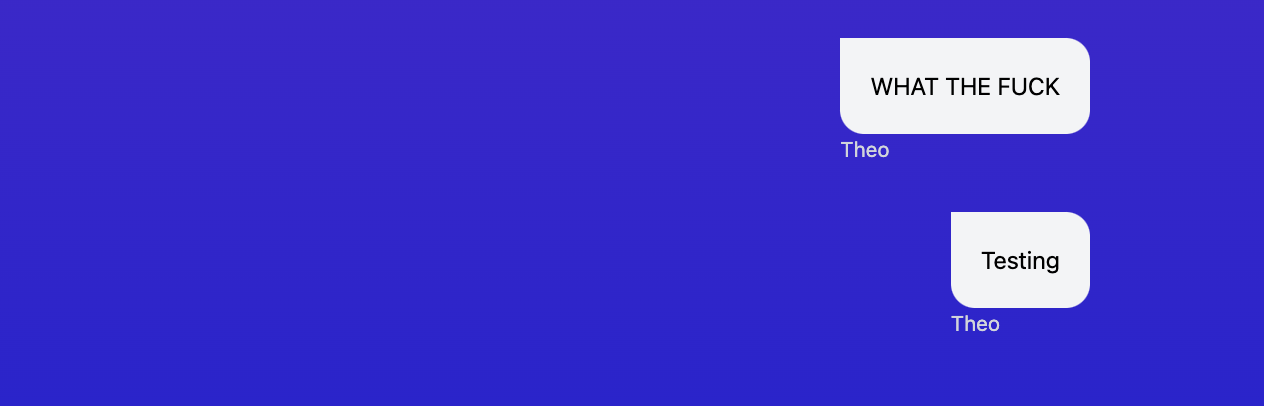
Client 2, misbehaving
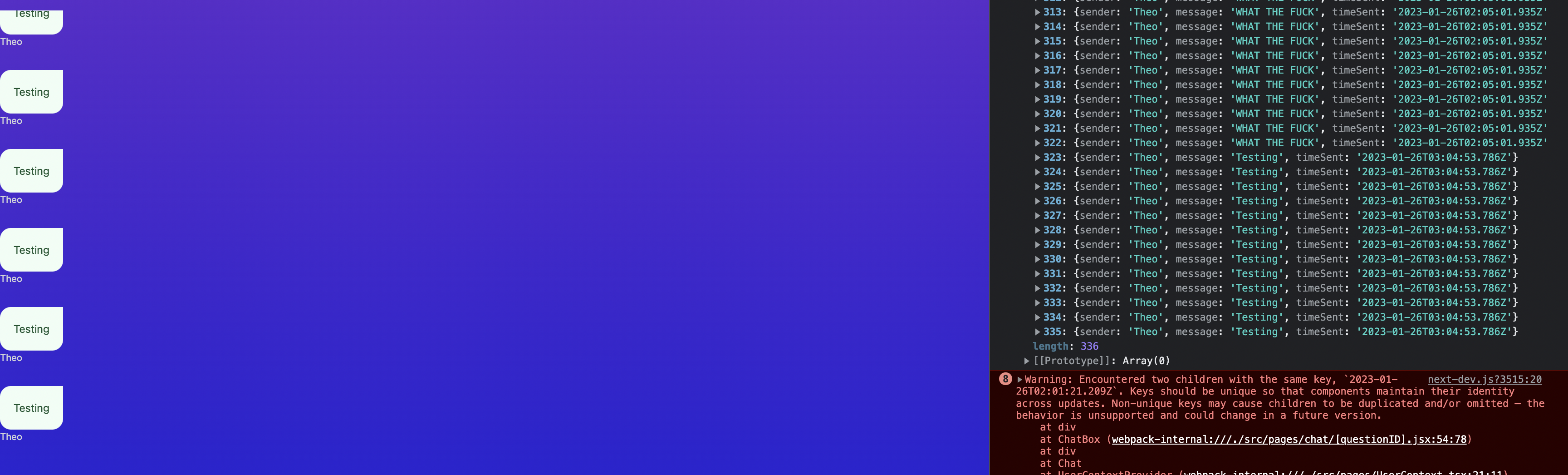
Idk man
I don’t use pusher or t3
Srry
Np 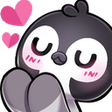
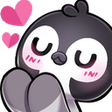
have you checked this out? https://github.com/pingdotgg/zapdos
GitHub
GitHub - pingdotgg/zapdos
Contribute to pingdotgg/zapdos development by creating an account on GitHub.
uses Pusher and syncs it to react using zustand
I have not, I'll take a look at it tho! Zustand seems a bit overkill tho since I don't need to share the state between components.
To anyone wondering, the issue ended up being that the cleanup