Battling TypeScript Types
I have been struggling with this typescript issue for a bit now and not sure how to fix.
I have an object containing some data that I import. It looks like this.
boom
has a crazy long type considering each of the objects in the array. (where I think this is making things hard).
The type for each item is automatically created, but I would rather it just be name: string and cost: Record<string, number>. How can I just simplify the types? My app works fine but I just cant get rid of this typescript error. Thanks!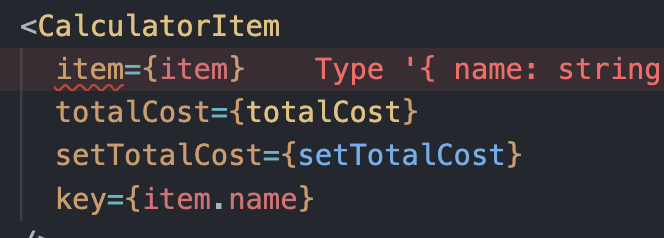
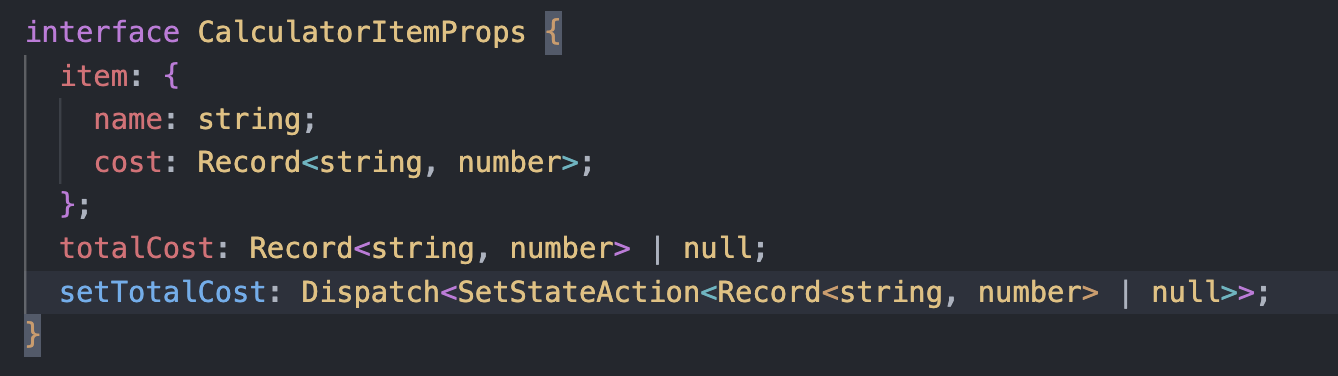
33 Replies
for complex types like this, you should declare the type rather than infering. This is a good case for types/interfaces.
I'll go with Record<string, number> for now for cost, but you really should also define these in a union type or enum, assuming their names are known ahead of time.
This kind of sounds like a game, so I'd probably opt for interfaces over types. Games are one situations where Object Oriented patterns can be useful
Ahh this helped a lot. “Declare instead of infer”. Could you give me a sample of what u meant by defining a union type or enum? I import this array of items, then map the array and pass the item to a component. In the component I type the item the same as Explosive in ur example and it works better. However, how can u be more specific? (There is nothing I have to specifically do when it’s a certain item)
Assuming there are known keys for the items in cost, then it would be good to define them.
Or maybe Material is another object you'd like to define
Alternatively, you can use an enum, even though these are a bit out of style today. They're still probably more appropriate in this case:
You can get as crazy as you want, really. Just think of any place where your IDE doesn't provide you proper autocompletion, and then see if there might be a better way to define your types. In this case, Record<string, number> is probably too broad. If you replace string with a union or enum, then you'll actually get autocomplete and type checking when you try to add a property to cost.
Cool, I will try to get a little more specific!
@exodus so I gave ur idea a shot and I am getting a strange error.

man is playing rust
Check it out!! haha
https://rust-meta.vercel.app/raiding/calculator
But in regards to that error, I am not sure why its expecting each cost object to have every single item, when the type for item is not requiring that
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
yh partial
Ohh ok, I did not think that was needed because of the
|
I think of that as OR
but probably shouldntyh no its mapping it
going through them all and doing
So
sulfur
and charcoal
are in every item, so a better type for this would be something like
lets see if that even works 😆
looks like it did 👀
So now my object.entries is being annoying because its not typing the destructured key nicely


anyone see how this is even possible? much appreciated lol
lol
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
Ok so for option 1 (just gonna cast for now), that worked well.
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
I use it twice (for now) but I think the other undefined error is unrelated potentially.
My increment adds to existing fields or adds entirely new ones.
My decrement does the exact opposite (obv).
@kapobajza so now, I think typescript is basically telling me that the newTotalCost might not have the key that I am trying to decrement (even tho I know it will because this will only reach if amount > 0, which means that at least one of these items is part of the total cost).
I think i need to find a way to tell typescript newTotalCost will definitely contain all of the keys I am traversing for the current item (item.cost)
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
ohh interesting
How would u recommend I indicate that the cost is nothing? still use null like u showed there? My main state uses the type
const [totalCost, setTotalCost] = useState<Cost>(null);
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
OHH
I had it like that wayyyy earlier but thought I was making it cleaner by doing it all in the Cost type
lmao, let me see how this works now, thank you!
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
Yep just changed that as well, I am still getting the undefined error somehow, might be something small
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
well that works, but is technically a little weird because it could go negative
theoretically it should never but I have a feeling its not exactly correct
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
@kapobajza resolved this madness by greatly reducing my state complexity. I now just store every item in the cost object starting at 0 and render the ones that are greater than 0.
no errors and much much much more concise code
AWesome. Yeah I should have pointed out that Record is usually something you reach for for dynamic keys...i.e. something that you don't know at compile time. Sorry, I don't know why i didn't go that route first