Noob Question Many-to-Many in prisma after nextAuth
Hello all! I'm trying to create my first many-to-many relationship in Prisma.
schema:
but i cant for the life of me figure out how to write the createTeam() function - this is as close as i've gotten...
20 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah i thought so too!

@iynaix i wonder whats up
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I think your problem is that you're using
connect
where you should be using create
. You're creating a new team for an existing user, so you're also going to create a new relation record. Using connect
like you have requires providing an ID to lookup, but that's not possible since no record exists yet and you need to create
it.Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
just got offa. call, let me see
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
it compiled, but jest is giving me some trouble... will check it in a min
hopefully
Invalid
Foreign key constraint failed on the field:
An operation failed because it depends on one or more records that were required but not found. No 'User' record(s) (needed to inline the relation on 'usersInTeams' record(s)) was found for a nested connect on one-to-many relation 'UserTousersInTeams'. seems correct let me check my test looks like the right kind of error 🙂 @iynaix! it worked! thank you users: creates the user join table for the user and then connects the user is that the correct understanding?
prisma.team.create()
invocation:
Foreign key constraint failed on the field:
userId
trying the second one
( which is closer to what i throught it should be )
Invalid prisma.team.create()
invocation:
An operation failed because it depends on one or more records that were required but not found. No 'User' record(s) (needed to inline the relation on 'usersInTeams' record(s)) was found for a nested connect on one-to-many relation 'UserTousersInTeams'. seems correct let me check my test looks like the right kind of error 🙂 @iynaix! it worked! thank you users: creates the user join table for the user and then connects the user is that the correct understanding?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
hmm where do i put the role?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
@iynaix haha i read that question too but i wasnt smart enough to extract an answer
thank you!
big help
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
now next question @iynaix if you know it
hah
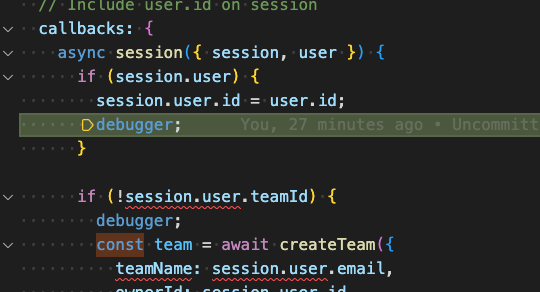
in [..nextauth] - where is the database query for the user? can i get it to add more info to the user?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
thanks then, its gone a long way alrady!