Turning rating (number) into rating stars
Hello, let's say I have some testimonials and each testimonial has it's rating, (e.g. 3.5, 4, 2.5 etc.). So what I'm trying to build is if the rating is 3.5 for example it should display 3 full stars, one half star, and one empty star.
Just to give you a better example of what I'm trying to build, I have two testimonials, one has rating of 4, and other one has rating of 4.5, so this is how they should display:
If you'd like to play with it: https://codesandbox.io/s/ratings-zhlnhx?file=/src/App.js
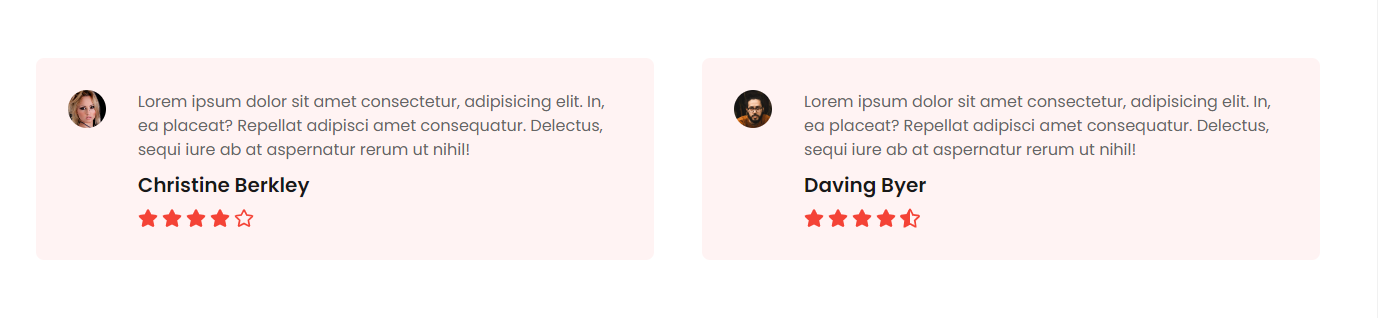
12 Replies
well, you can just use svg or a font icon that is full, half or empty
for exmple ion-icon (there a more)
ref: https://ionic.io/ionicons/usage
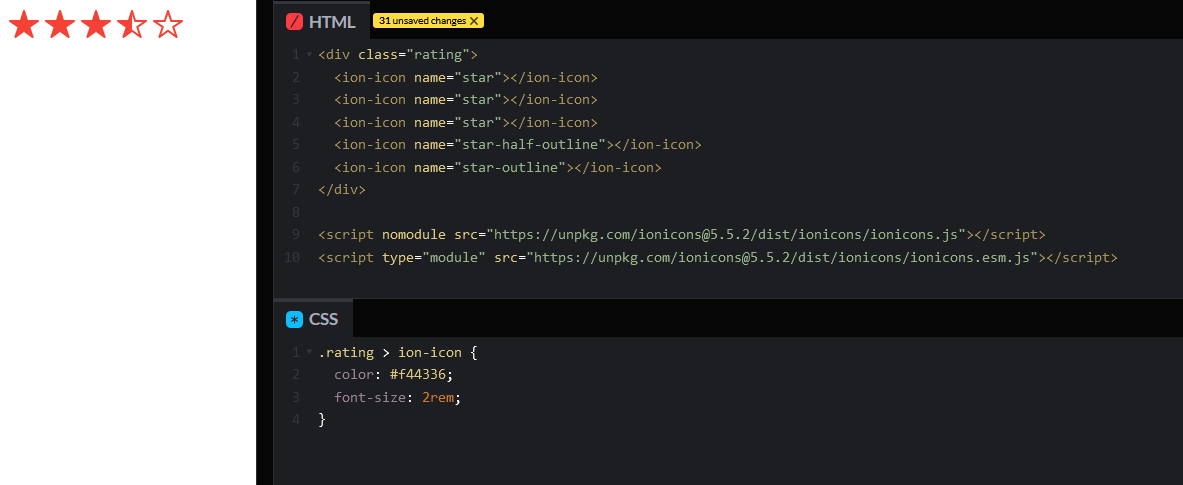
played with it a bit more, here a way with font-awesome in a single div, and css calculating the filled stars
https://codepen.io/MarkBoots/pen/yLqWpmd
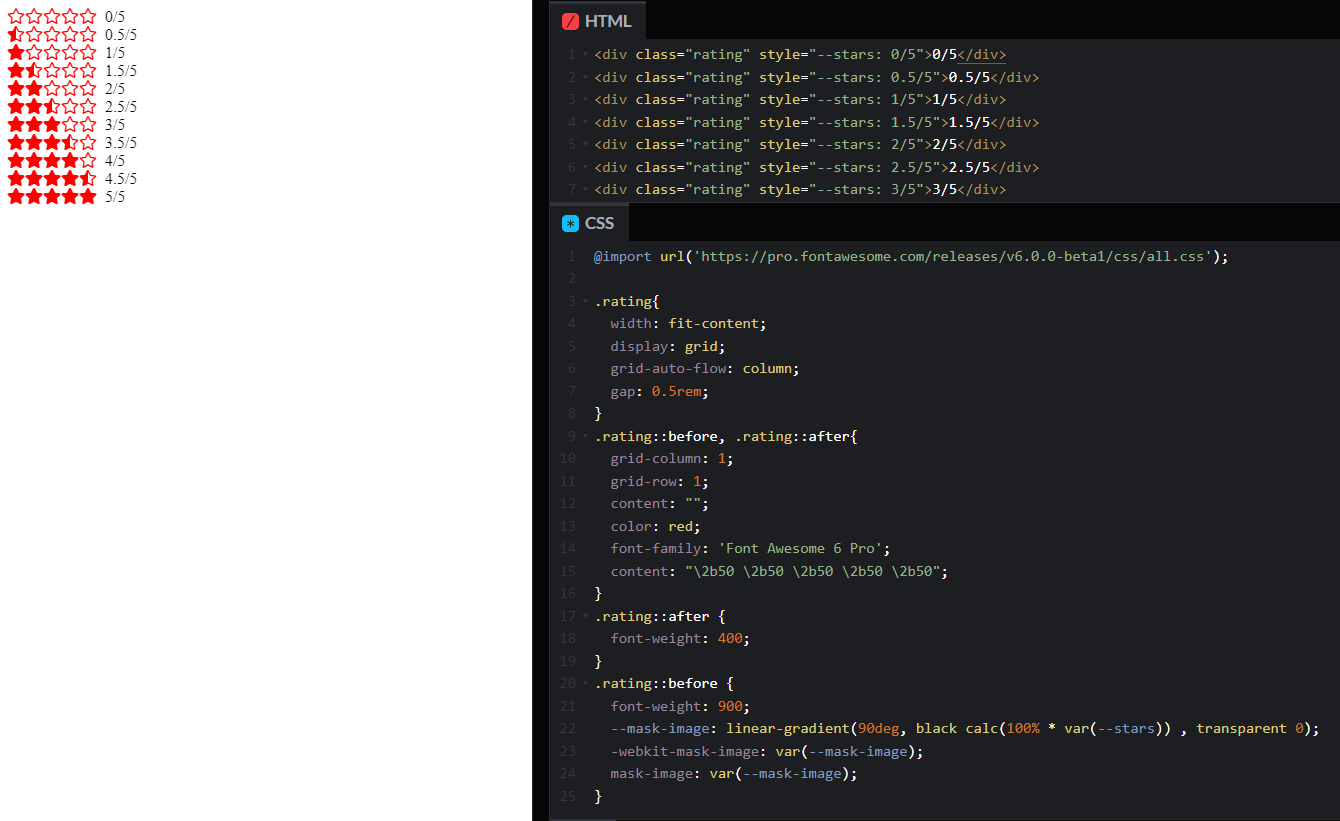
I was actually looking for Javascript, or rather mathematical solution to this. So it should see that rating is e.g. 3.5 and using (probably) some for loops and if statements it would display needed stars for it (3 full stars, one half star, and 1 empty stars). But I guess if I can't find better solution I can just put it all inside of one big object and work with that:
I would 2nd Marks' suggestion as that is much more performant BUT I would suggest that the elements be inputs rather than divs and use the steps to control the star rating. This will allow it to be accessible
make certain that the input range has steps set to 10 i.e.
then programatically you can update the aria-valuenow to the appropriate star rating
I do agree with Coder_Carl and his additions
But still want to show what an option could be incase of JS and instead of a big object
You could make a little helper function that takes the score and amount of stars and returns the elements (strings in my case)
Something like this (could be cleaner and more efficient probably, but I just woke up)
Yes! that is exactly what I needed, thank you! I also remembered ChatGPT exists so I asked him too, here's his solution if you'd like to see it:
Pretty similar to yours
Wow, chatGPT stole my work, or `maybe I am chatGPT 🤔
It still misses the check for 0.5 and it could be more generic with the amount of stars though
well somehow it works for 0.5 lol and I don't think there's need for amount of stars since it'll probably always be 5
Not sure, if still relevant, but here's my approach
https://codepen.io/CodeNascher/pen/ZEjNJew
Might not be the best solution, rather a quick idea that worked well enough
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View