getServerSideProps() fetches NextAuth session put fails to pass props to Next.js page components
I'm building a note-taking app with ct3a, currently working on implementing basic auth features in the UI. Here's my GitHub repo - the relevant code can be found in the
auth
branch:
https://github.com/ChromeUniverse/luccanotes/tree/auth
I'm trying to call getServerSideProps()
in /pages/notes/index.tsx
to fetch my NextAuth session with ct3a's getServerAuthSession()
wrapper and pass it as a prop to the main NotesPage()
page component. Next.js appears to be fetching the auth session properly - I confirm this through the serialized __NEXT_DATA__
in the browser - but for reason the session won't get passed down into the main page component. Server-side console logs also confirm this:
I've tried strongly typing getServerSideProps
and my main NotesPage
page component with Next's TypeScript types but still no luck:
What am I missing here? Did I misspell any functions or forget to export a function that Next.js needs for server-side rendering?GitHub
GitHub - ChromeUniverse/luccanotes at auth
A full-stack note-taking app for Markdown lovers ❤️ - GitHub - ChromeUniverse/luccanotes at auth
25 Replies
Here is the serialized
__NEXT_DATA__
in the browser. I'm assuming this means that getServerSideProps()
is working? But why isn't the actual page component receiving the props?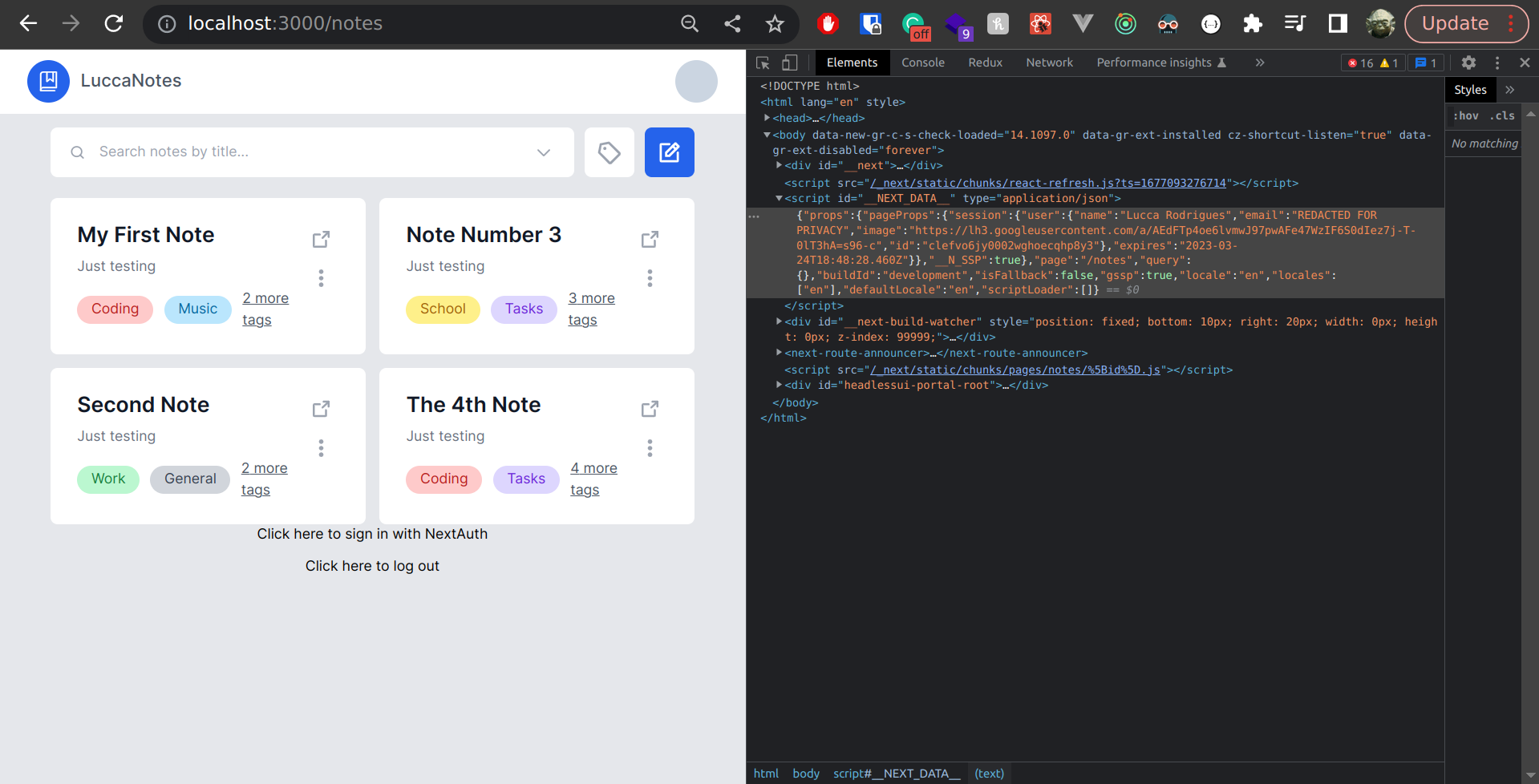
You still need to call useSession in the component
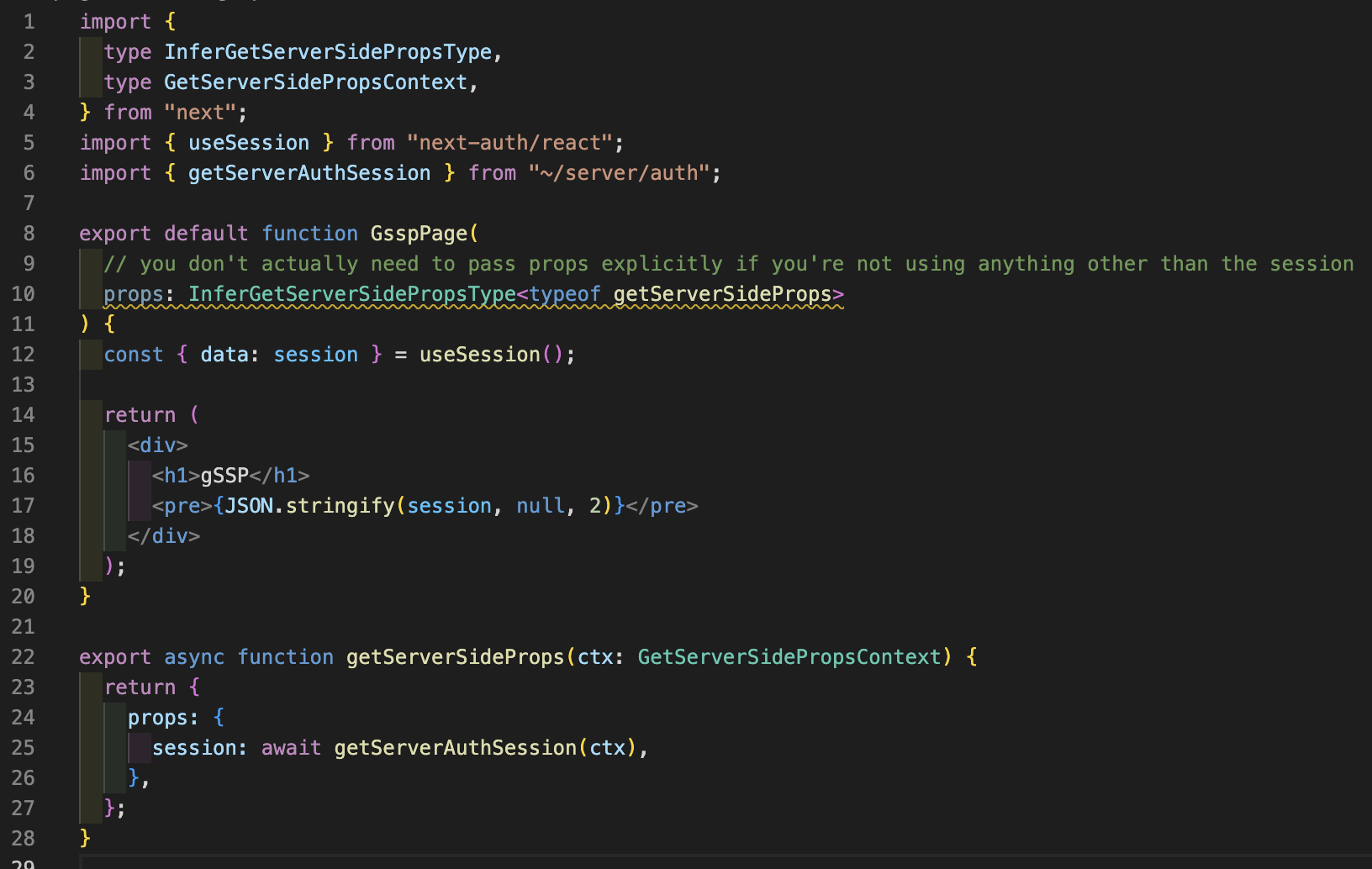
But why would that be case? If you're already passing the session to your component through
getServerSideProps()
what's the point of the useSession()
hook? I couldn't find anything mentioning this in the NextAuth docsThe session lives in context
look at what happens when you pass a second arg in props
i agree it feels a bit like magic and could be documented more clearly
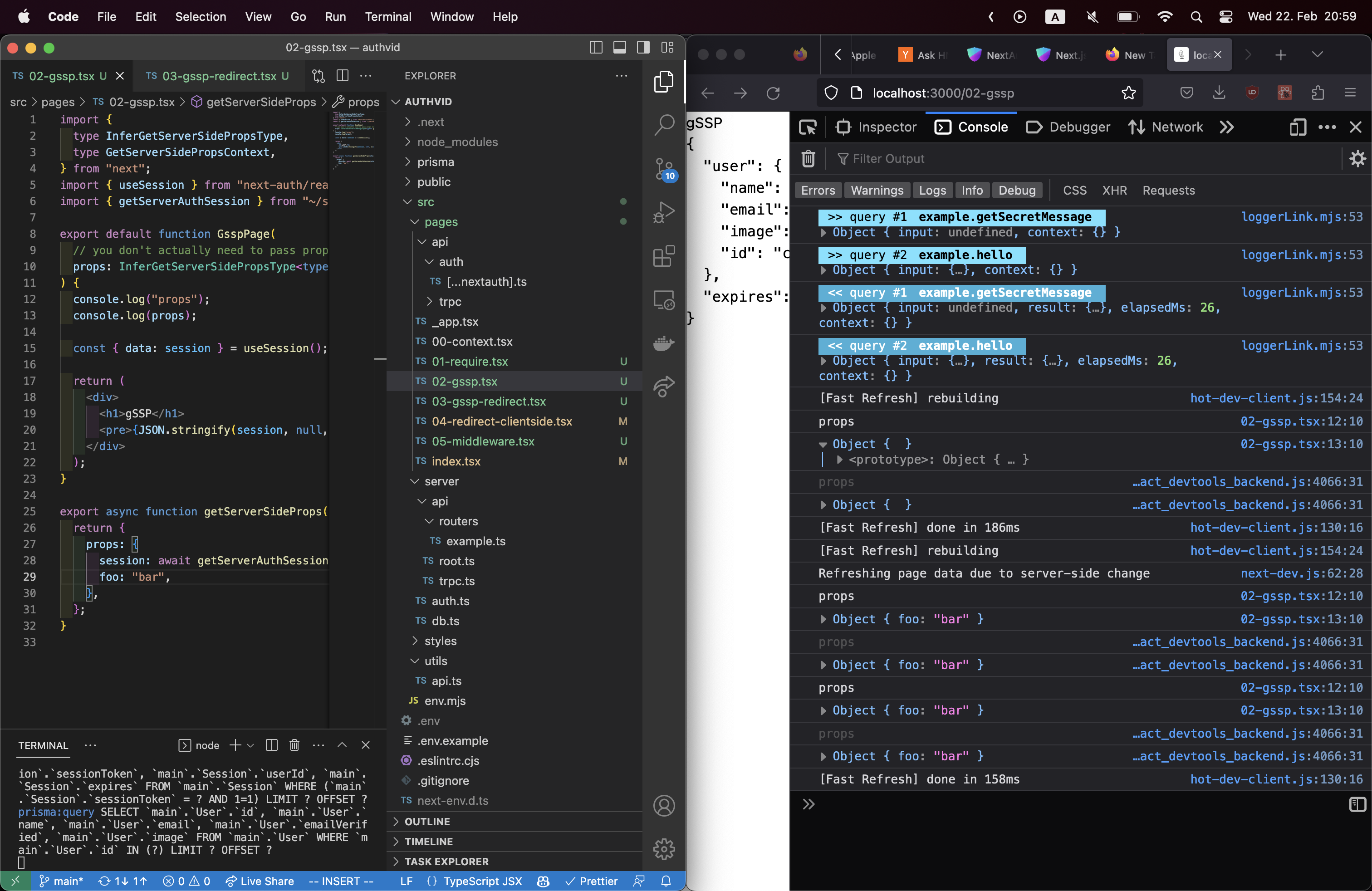
Interesting. Got the same results here. So literally every other arg will get passed to components as props expect the NextAuth session?
that's pretty weird ngl
Yeah I've been double-checking the NextAuth docs and I still haven't found any mention of this
but using gssp and
useSession()
totally works
The page is in fact server-side rendered but the session gets passed as a prop behind the scenes and is only acessible through useSession()...? I think?Look in app.tsx
When you log props in your component, you’re not logging directly what gssp passes
Session gets destructured iirc
Oh, you mean this? (line 20)
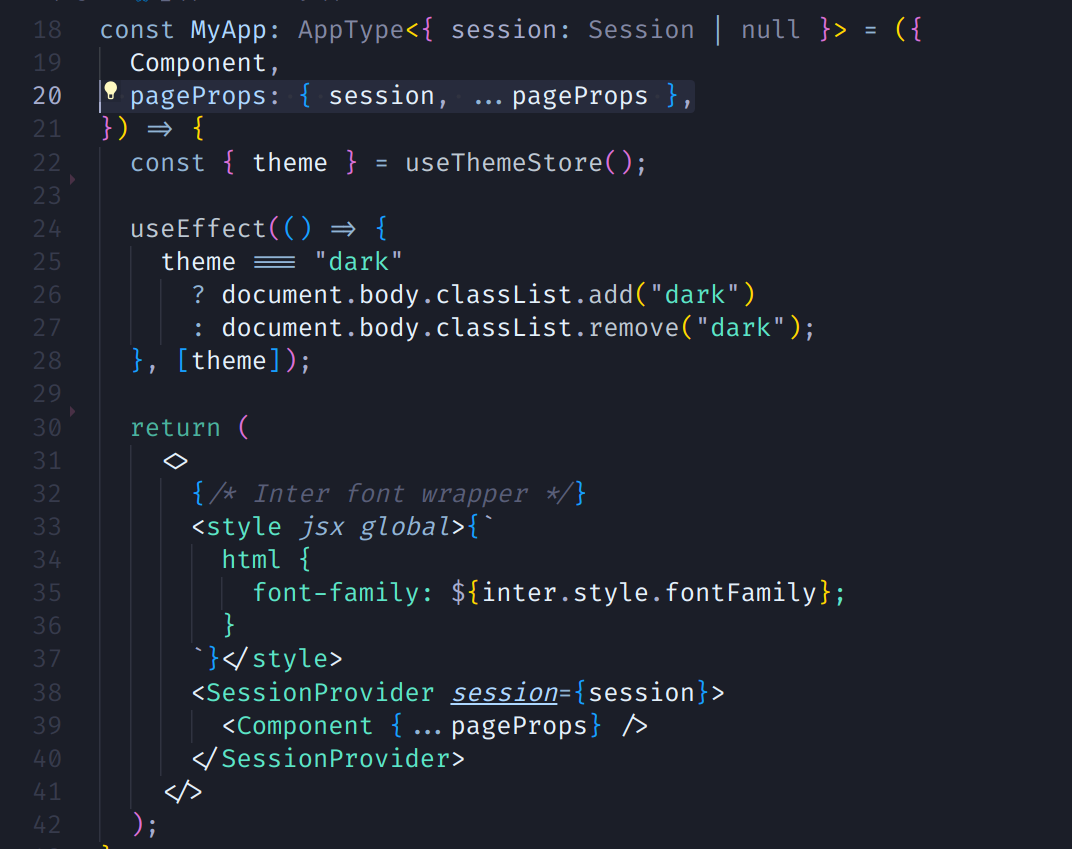
Yea
Session gets put in the provider and everything else gets passed down
well shit
that actually makes sense
Having session as a random object in your props wouldn’t actually do much
Okay but something that's still kinda confusing is that
useSession()
still works even if you don't use gssp in your page
I'm not sure what it does behind the scenes (an API request maybe?) but it can still return your current auth session without the page being server-rendered firstUseSession first checks the provider
Or actually that’s not really true
It always only checks the provider
But if the provider has no session or an outdated session, it makes a network request
Iirc it actually treats the gssp session as outdated so it fetches again on hydration
huh
the more you know
tysm! @cje
btw how did you figure this out? I mean, the session destruct in
_app.tsx
seems kinda obvious in hindsight but again, there's nothing in the docs about this useSession()
behavior, and using gssp seems like a pretty common use-case too imoI put some console.logs in the callback functions that you can customise
Also read some of the library code a while ago
When create t3 app’s auth. was suddenly broken due to a bug in next-auth
Oh i see, cool cool cool
I guess I'm just surprised I've haven't seen this mentioned elsewhere
thanks again tho
If in doubt, command-click the import 🙂
in this case what import should I cmd-click exactly? 😅
Whichever one you don’t understand what it does
But that usually just points to TS type definitions right?
This might just be my noob brain kicking in but I'm not sure how to take advantage of this (in this particular scenario with
useSession()
specifically)All the libraries are just JavaScript
So you can read them
It’s difficult at first, but a good thing to get started with early
Also when you’re reading files in your node_modules, you can stick console logs etc in there
Oh right, I see what you mean
Yeah I'm ngl, reading through lib code seems kinda daunting
Messing around with code in node_modules seems kinda dangerous lol
I mean it's not even version-tracked, is this actually safe to do?
If i end deleting something on accident, do I just
npm i
again?Yea
okie dokie then lol
Yeah imma give it a go sometime later then
Thanks again!