Simple JS operand
I got curious about something, but, let's present the following case:
Why is it
a = a * ( 2 + b )
, instead of a = a * 2 + b
. Is there any logic behind it? Or do I just accept the fact that a short hand for *=
would assume anything after would be in a parenthesis?33 Replies
I don't think there's any logic behind it, it's just a shortcut to multiply (and re-assign) the variable
a
by the value on the right, so naturally it must come in parenthesis. Or in other words, it must be resolved before the operation can be performed.Why? Because that's how it's designed to work:
The multiplication assignment (*=
) operator multiplies a variable by the value of the right operand and assigns the result to the variable.
āhttps://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Multiplication_assignment
The right-side of the =
is evaluated then multiplied by the left-side. If you want it to work differently you can't use the multiplication assignment operator.I see, so it's just life. Makes sense.
If you expect JS to make sense, I have some real bad news, my friend
I'm not expecting anything to be frank. I just wanted to understand if there was any logic behind it.
If there's not, that's just the new logic to me.
There is logic, yes. In this case not the logic you expect
[the more you know].gif
This is a bit more tricky to me, but why exactly is this a string called "NaN"?
Because
grapes
, bananas
and pears
are not numbers. If you try to add a not-number to a number you get NaN (Not a Number)But it's a "NaN" string?
It's not just a NaN, it's a string.
That's because
"NaN"
is, indeed, a string
That being said, typeof Number.NaN
is Number
:pSo,
let fruits = grapes + 7 + bananas - 7
= NaN
But let fruits = grapes + 7 + bananas - 7 + pears
= "NaN"?
Why is that I guess that's why I'm confused.Here's the thing,
"NaN"
is a string, but NaN
(without the quotes) is of type Number
. It's Number.NaN
However, the JS parser doesn't give you Number.NaN
it gives you "NaN"
the string. For some reasonShouldn't it just be NaN?
It should, but for some reason it's not
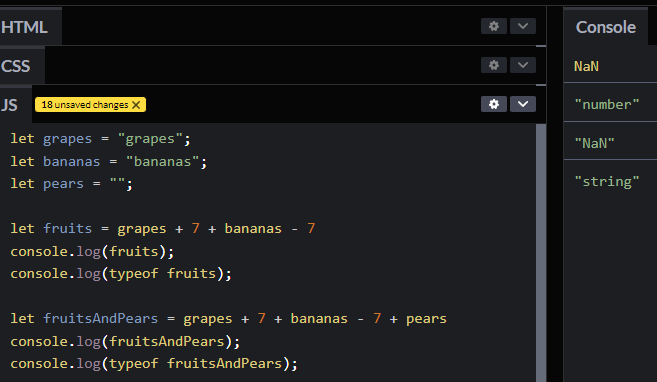
That's why it had me 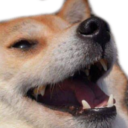
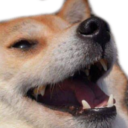
Interesting
Obviously it has something to do with the empty string represented by
pears
but I have no idea whatš¤
I was doing some exercises, and started trying things, and came across this on my own.
And I was confused if I learnt it incorrectly, or not.
At least my understanding was:
"string" (grapes) + "number" (7) => "string" (grapes7)
"string" (grapes7) + "string" (bananas) => "string" (grapes7bananas)
"string" (grapes7bananas) - "number" (7) => NaN
NaN + "string" ( empty ) => "string"? (NaN)?
It's like adding the NaN into the empty string and treating it as a "string".
INTERESTING
If the last operand is
ā
then it's Number.NaN
!Well, depends, if the previous is a string with a number inside of it, and no letters, it will treat it as a number.
But if it has any letter, it will be treated as a string, hence NaN.
So "7" - 7 = 0, but "7px" - 7 = NaN.
Well, yes. That's how JS works: if it sees a math operator then it tries to coerce things to numbers
But I still don't understand where the logic comes from to make NaN a 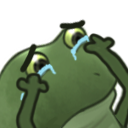
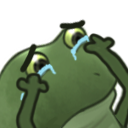
The
+
operator can be used for two things:
⢠Addition
⢠String concatenation
So if there's a string that can't be coerced into a number it treats it as multiple strings being concatenated.
However, the subtraction (and other mathematical) operator (ā
) is only used for maths. It will coerce everything to a number, and if it can't be for some reason ("apple"
) then it becomes Number.NaN
. And if you try to do any maths on Number.NaN
you will get Number.NaN
.
So for your above examples, any that don't include the subtraction operand will treat it like a string and make it a string at the end (even if that "string" is Number.NaN
)
And because JavaScript follows mathematical order of operations, if the subtraction is not the last operator, then it'll be coerced back into a string if there's another + [string]
afterwards
Another thing that dilutes the "what the eff is the +
anyway?" is that it also is the Unary Plus operator! If anything is proceeded by +
then it makes it a number! +[something]
is a shortcut for Number([something])
.
The plus sign is indeed multitalented and multi-annoying!Thank you for the explanation.
I was going through javascript.info course in the operators.
I hope it makes some sort of sense >_<
I was simply confused for the behavior.
The JS.info course explains it pretty well.
Nice!
But as usual, my intrusive thoughts wanted to try different examples.
And that's how I got 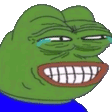
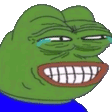
JS.info is great
Trying and succeeding or failing is how we grow
Today you learned something you wouldn't have if you didn't try to break things.
Basically.
My intrusive brain always wants to break what I'm given, lol.
I try to break shit all the time. Some times it breaks and sometimes I break lol
Thank you for the help :)
Of course!