I am trying to run a background promise
I am trying to run a background promise to drain logs in a durable object that looks like this:
With
logger.Drain()
looking like:
Looking at my execution logs within cloudflare, I see only the draining logs!
log, but not the done!
log. I've also tried returning the googleDestinationFunction
log directly (which also contains logs that I do not see) and it just seems like the DO is exiting once the promise starts running... It is my understanding that the DO should let any other promises run the in background, correct?8 Replies
In more detail, I am using the
hono
framework and the route looks like:
(I adjusted the desintationfunction and same issue)added some logs in the promise and I can see that it only gets to internal log 1/8 before it exits
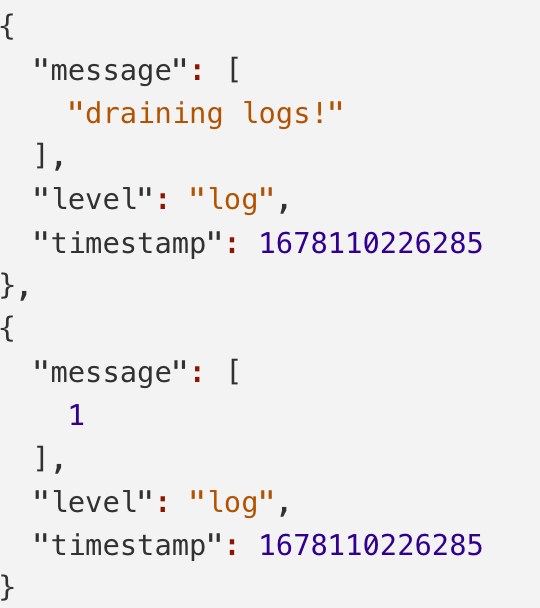
unless it only includes logs before the response.... but still I am not getting the logs in google
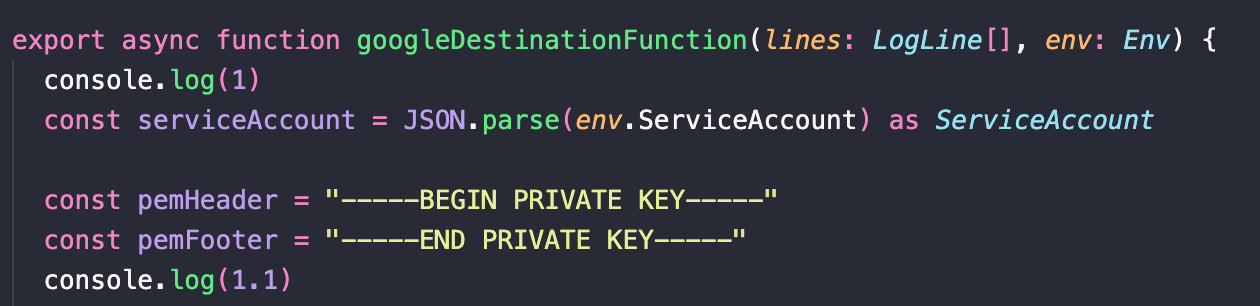
you're not awaiting logger.Drain() so this seems like expected behaviour to me
if you don't wrap it in a waitUntil then the Worker will just close after returning a Response
https://developers.cloudflare.com/workers/runtime-apis/durable-objects/#durable-object-lifespan
According to this I don't need to await for DOs
Ah, a DO
Nevermind then, no idea haha
D:
there are no exceptions too so it's not erroring on the
JSON.parse()
WOW ok figured it out
this is cursed
changed to return googleDestinationFunction(lines, this.env)
(using the DO reference to .env
vs the hono reference)