The application did not respond
When I try to run my command, it doesn't respond and there are no errors
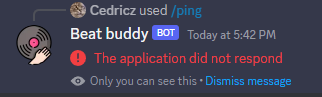
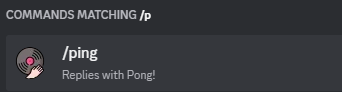
7 Replies
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
const command = interaction.client.commands.get(); should throw an error, at least it did in my code once
Do you get an error?
yes
no
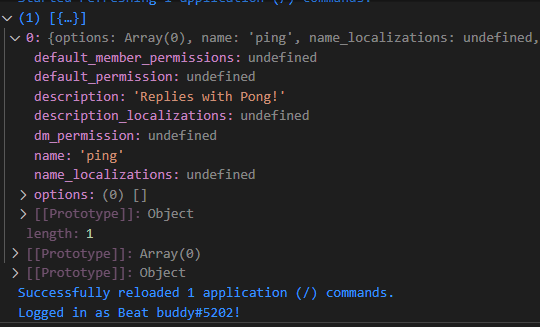
everything looks fine and it should work
If you aren't getting any errors, try to place
console.log
checkpoints throughout your code to find out where execution stops.
• Once you do, log relevant values and if-conditions
• More sophisticated debugging methods are breakpoints and runtime inspections: learn moreforgot 1 line of code in my index file =-=