Prisma schema changes when moving from NextAuth to Clerk
With NextAuth I have a User schema that contains many relation fields. For example:
If I were to move to clerk and therefore no longer manage my users in my database, would I just have to delete the User model and then remove the relation in the Post like so?
and then adjust my procedures accordingly? Is this all I would have to do or are there other changes to keep in mind?
13 Replies
heres what I did
and then whenever you have a write involving a account just
upsert
Cool thanks
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
did not even know that was a thing
Sync data to your backend | Clerk
Learn how to sync Clerk data to your application's database
@Ramsay I would look into this as well
heres what I am moving to
then you can just
the one asterisk is that as pointed out in the clerk page I linked it is asynchronous, so there is a slight delay between a user creating an account and the account existing in the database.
Should be fine as long as you arent doing anything with that account at the exact same time as they sign in
actually
I was going to say theres no need to store the other data besides the id in my database because it would just get out of sync with clerk, and its easy enough to fetch from clerk whenever you need a users email or something
but with a webhook you are informed whenever a update changes so you could have a cached version of the user's entire info if you wanted that updates on a webhook
just depends on your usecase
Awesome! I'll check that out
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
So full disclaimer I am not a database person so very open to being corrected on this.
Basically my understanding (I watched this video https://youtu.be/xAQga907NVU) is that indexing is a way to store a specific part of your table in a faster to search data structure.
In this case we know that the majority of our operations on this table will be searching by ID, so by indexing the ID we are greatly improving the performance of any lookups.
Computer Science
YouTube
Database Index Fundamentals
This video explains the fundamental principles of indexing table columns in a database to speed up queries. It illustrates the difference between clustered indexes and non-clustered indexes, which are also known as secondary keys. It explains that the primary key of a table is normally the one and only clustered index, because this defines the...
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
thanks for sending this, my method was very scrappy and this looks like the right way to do this
only question (and just lmk if this is a question for clerk and not you) is how do I replicate the svix header signature during testing (I'm using webhookthing)
I see these are what they should be but just copying over is not valid
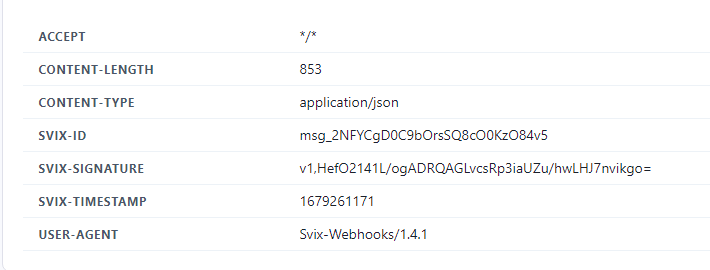
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Just for any future people who search this
clerk is working on a intergration with webhookthing: https://discord.com/channels/856971667393609759/1082526277216522251/1087493688453771346