How do headless-ui expose context variable for child components?
Hey I'm trying to recreate a similar pattern to what is used in
headless-ui
Menu components.
https://headlessui.com/react/menu
As you can see open
is passable as a variable to be consumed by the children. How can I recreate such a pattern?Headless UI
Completely unstyled, fully accessible UI components, designed to integrate beautifully with Tailwind CSS.
8 Replies
This example probably shows better what I'm trying to achieve (but is wrong):
I've not seen this way of passing probs before, seems like headless calls it 'slots' but I can only find Vue style examples
Ohh... you can just pass children the value like:
Does this have a name? :D
When I use in typescript, it's saying ReactNodes aren't callable
I just replaced the type like so:
Not sure if that is a hack or not lol...
ok.. seems like im running into a boundary error.
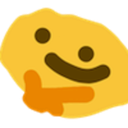
Functions cannot be passed directly to Client Components because they're not serializable.
:(I was working on sort-of wrapping the Headless-UI components so that I could create reusable UI components, the goal was to basically have some base classes, but still keep the same pattern, where children could consume props from parents
I didn't exactly nail it, I still ended up with some bugs, but I could share some of that code if it helps
For example, in the H/UI Listbox component, the children are typed as:
To me it looks like you were on the right track at least
Oh nice thank you! I'll have a look at those types, but really my issue now is with server/client rendering with next app.
Ah, I'm not sure about server/client rendering
I believe my stuff is entirely client rendered. I'll create a gist with the Select component (wrapper around Listbox), maybe it helps.
Yeah I'm trying to make a suspense preview component 'generic':
page.tsx
PreviewTest.tsx
Thanks I'll check it out!!
@.3819 I'm running into a similar error here, did you figure anything out?