Guidance on how to handle .json with many objects which contain .svg images
#general
I have a "jobs.json" file which holds many 'job posting' objects which will be imported into my index.js and then looped thru, in order to ultimately have their information be added to the html via template literals.
My issue is that each object includes, as its "image" property, an .svg image.
What would be the correct way of setting up these .svg images inside the objects in my jobs.json, to ensure that I can successfully import and access them from my index.js?
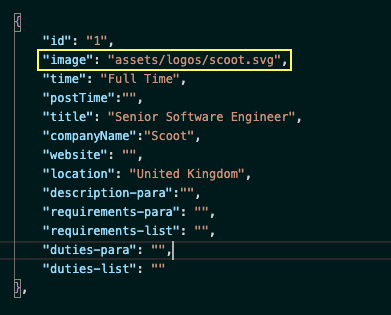
4 Replies
If you have a full stack application, you would usually want to store your images in something like a AWS S3 Bucket and have a field in your database that points to the Bucket link. And then your server processes that links as a file and sends it to your frontend using an API (I could be getting some details wrong, but I think this is generally how you go about it).
If you are only working with a frontend, I would set your repo up to use absolute paths so that you can reference your assets directory easily.
So for example:
Let me know if this doesn't answer your question/doesn't work; I didn't test it
This very helpful, thank you.
The "alt" parameter in the "createImage" function.. is that just for another version of the image, in case I had another one in a different size or something?
The alt parameter is for the img's alt attribute
GitHub Repo: https://github.com/nnall/FEM__Devjobs.git <-- node_modules folder not included
Live: https://nnall.github.io/FEM__Devjobs/
So I was able to use a template literal to insert the cards. The only issue I'm now having has to do with the <img> child elements of the cards not displaying their src images (attmt 1), which are .svg's.
I happen to be running Parcel bundler in this project, and according to its docs (https://parceljs.org/recipes/image/#javascript), in order to access an image from JavaScript, which I'm doing here with this template literal, I need to use a URL Constructor, which I'm now attempting to implement (attmt 3), but which I'm having trouble with.
Although when I console log the created URL {} objects (line 76), I do see the objects appear, along with the correct 'href' path to the correct .svg file, when I attempt to then reference the created object inside the template literal, as the <img>'s src value, I am then met with a "not allowed to load local resource" error.
So I'm trying to understand now how I am to integrate the constructed URL {} objects (containing the path to my .svg's) into my template literal so that they'll display.
GitHub
GitHub - nnall/FEM__Devjobs
Contribute to nnall/FEM__Devjobs development by creating an account on GitHub.
Image
Parcel has built in support for resizing, converting, and optimizing images. Images can be referenced from HTML, CSS, JavaScript, or any other file type.
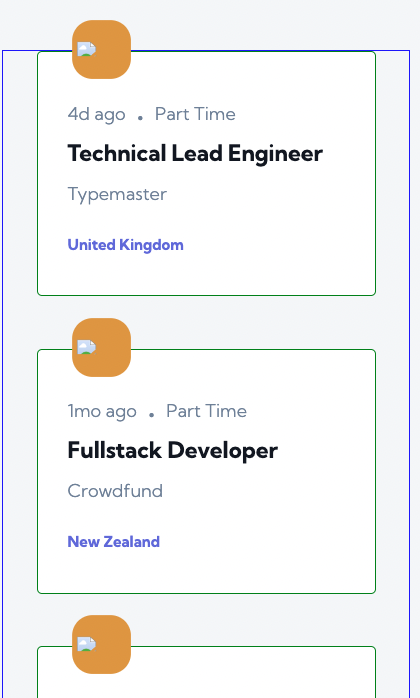
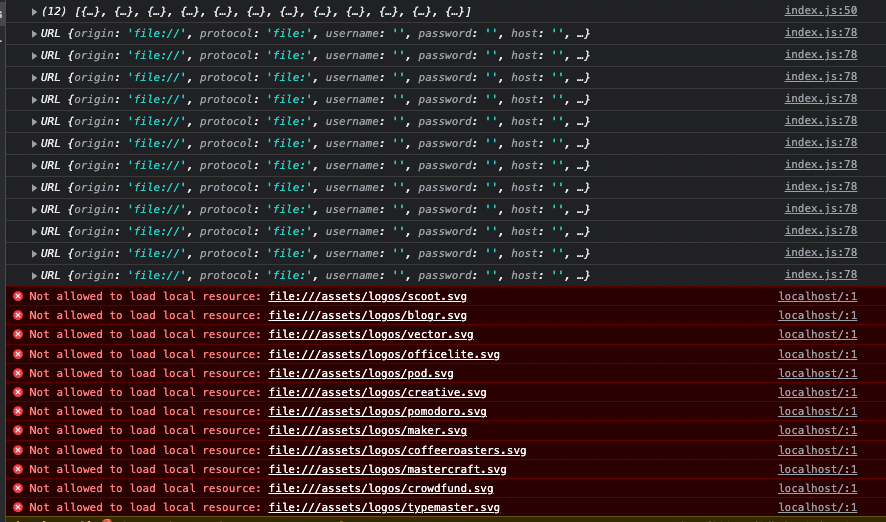
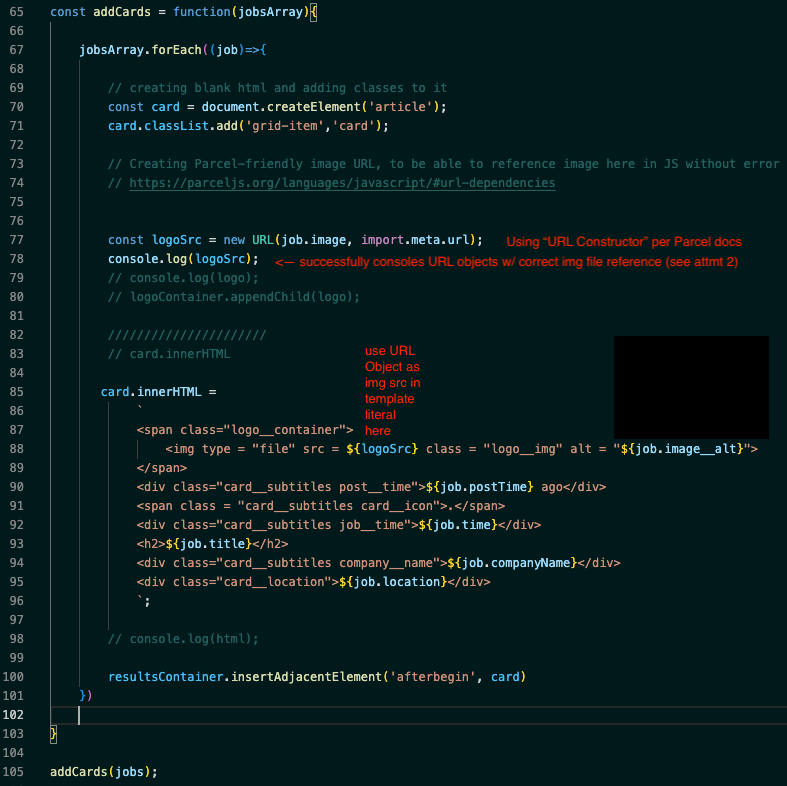