Receiving button interactions
Hello there, I'd like some help regarding button interactions.
I know how to build a button row and send it (see code below)
But I don't know how to receive interactions from it... I've read this page : https://discordjs.guide/interactions/buttons.html#receiving-button-interactions But still.
So if someone can help a bit with that, it'd be appreciated ! :)
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
9 Replies
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
-- discord.js@14.8.0
v16.16.0
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I'm having trouble finding out where to put my code, as I already have an interaction handler in my
index.js
for command (/) interactions and it then triggers the right command (which is in its own file)
But as we're not talking about slash commands here, I'm not sure how to setup my file, what should I put instead of this :
For the functions which should be called on my button interaction
thank you, I think it's more clear now, I'll give it a try and let you know if I finally got it :)
2nd option, should I export the customId
in a data :
? Then what class should I use instead of SlashCommandBuilder
?
Something like this then ?
Then I have an error when deploying commands :
Do I need to deploy it or is it useless and I should rather put the button files in a separate folder ?
okay thanksok so it looks like it's working (logs + rôle added correctly) but I still get this :
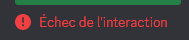
well I handle it like that :
oh I didn't know I needed an
interaction.reply()
, I just added one anyway
thank you again !Responding to interactions:
•
#reply
immediately respond with a message
• #update
immediately update the original message (buttons, select menus)
• #showModal
immediately show a modal (cannot be deferred)
• #deferReply/Update
respond later (up to 15 minutes)
• #followUp
post an additional message
The initial response has to happen within 3s of receiving the interaction!got it :)
oh and, as we're here, I'd like to move my handlers code to a dedicated file in a
handlers
folder (just so the code is cleaner) but I don't really know how to do that
(I want to move those : https://discord.com/channels/222078108977594368/1089990904451960903/1090355280090509424)
My problem is that I don't know how to exactly setup the file and have the index.js
to run them correctly before login