If statement is NOT working
I am making a Rock, paper, scissor game. But the If statement of the code is not working. What can I do?
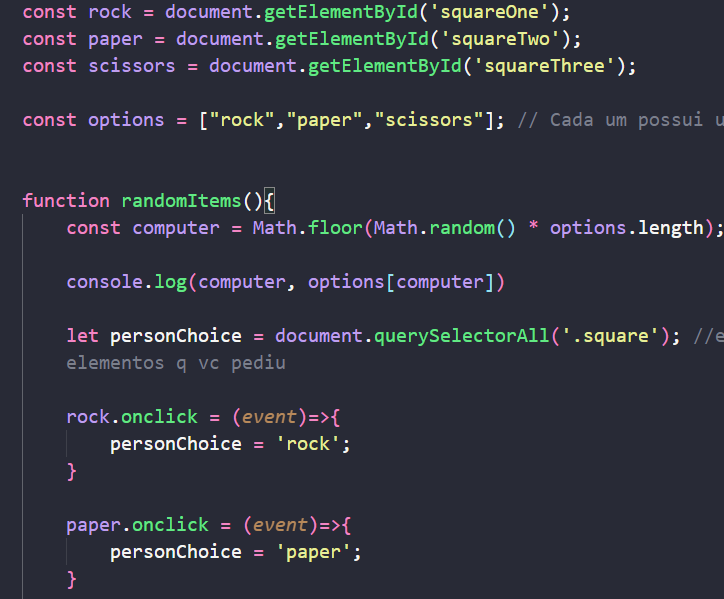
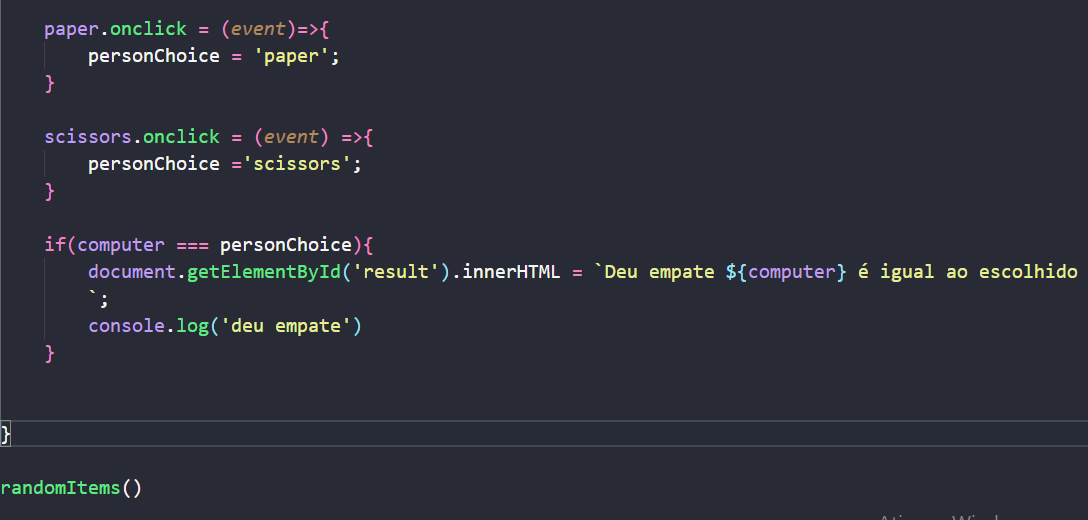
47 Replies
I am going to guess that you intend for the if statement to run after the
onclick
methods fire. Unfortunately, it is defined right on the window. So it gets called as soon as the file loads.woww. What can I do to resolve it?
Actually it looks like its inside the randomItems function, or?
I am immediately wrong, I missed the context.
@Sstephanyyy Can you share the code over on codepen.io or similar?
yes, it is inside
yeaH. Just a moment,pls
But actually I think you are correct in that the event listener is being added every time the function is called. Rather, the event listener should be moved up to the window. That may be it
it doesn't show any errors?
in console
not
in the console, show the computer choice
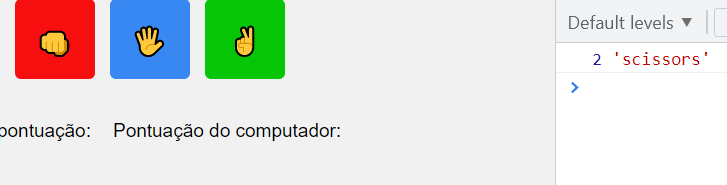
I like your style @Sstephanyyy - it looks good.
thanks. Sorry, it is in another language
The problem appears to be that the logic that does the judging isn't getting called on the click.
really? why?
It gets called immediately when the file loads, which does create the listeners, but those listeners only update the state (
personChoice
) without calling on the code to update.
Try moving this:
Into a function, and then call it in the event handlers.here, since u r defining the value of personalChoice using
querySelectorAll
, doesn't that mean the personalChoise is actually an Array here?I used the queryselectorall to get all the squares and then put the onclik. So, I think it is an array
so if it's an array, how can u define the value of an array using =
what do you mean? I am still a beginner, can not understand properly what you are saying
is already has 3 values
so doing
personalChoice = "rock" / "paper" / "sissor" doesn't make sense
what is the right way to do it?
The gist of this game is that you need to calculate the winning conditions on every round. So everytime you click on a square, you have to 1) check where the user clicked and make a selection accordingly, 2) randomly generate a selection for the computer and 3) compare them.
in the if statement, I am trying to compare the computer choice with the person choice
well delete the query selector part
try only keeping
let personChoice
basically keep the value undefined
then you'll give it a value depending on which button the user clicks
let personChoice = ' ';
this way?
noo
let peronChoice;
that'll keep the value undefined
untill user clicks the button
okay
just this or I have to change more thigns in the code?
The logic to compare the options is correct. The reason why it doesn't seem to work is because you are expecting it to check whenever you click on it. Currently, you are only running that code when the page loads.
because, still doens't work
and also put the if condition inside a function
and then call the function when clicked
I get it what you said, but I don'tknow how to write this in code format
This code clears up a lot of the problems:
rn the if runs as soon as js loads
u want it to run when button is clicked
it is already inside
yes
yes so you need to put the if condition in it's own function
and call it when button is clicked
rn it's inside the same function with the button.onclick
Ok let me give you a hint just a sec
thankss. But why did you create two functions?
where is the condition tho
The important changes:
1.) call judge when the user clicks
2.) create a new random value each click (good work on generating this "AI" portion by the way)
3.) the "computer" value was actually be used as a number instead of an index into the options array
The randomItems function can be removed entirely if you want. I just didn't want to rewrite everything you were doing. The important function is
judge
which I call every time they click. What is important is not that they exist in a function, but when the function is called.
randomItems
gets called only once. When the file loads. judge
gets called in each event handler.
Ok first of all, I've renamed the elements from squareOne, etc to their actual values: rock, paper, scissors
This makes things so much easier later on. For example you can just know right ahead what the user clicked based on the element's id.
Notice
options
now is what you called squares. That's jsut my own way of thinking and the anme here does not matter. What matters is that I'm telling it to listen for click events.
This way, every time you click on it it will run the function we are providing.
Human choice will be equal to the id of the element we clicked, which conveniently is one of rock, paper or scissors. Or undefined, if you click on the background which is why I'm checking for that as well. If it's undefined just return, do nothing.
Once you have the humanChoice variable, you can run another function that I'm calling calculateWinner. HEre is where you can calculate the computer's option and compare it.
For reference, in the future don't use el.onClick
as that's the old syntax that while it works is not recommended. Create an event listener like I did.
The reason being that you can later remove them and keep track of them much easily.
If none of this makes sense, just ask 😄thanks for the help Joao
could I use the foreach functionality as well?
Of course 🙂
@Sstephanyyy https://codepen.io/-bloop-/pen/ExeJaqe
k so after opening your code in my editor i realized there was quite a few mistakes u made
the biggest one was,
your compuer variable has an integer value
but your personChoice had string value
and in the condition u were trying to math the string with the integer
if u compare my code with yours you'll understand what I'm talking about
thanks for the help
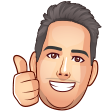